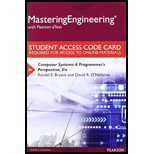
Concept explainers
Explanation of Solution
Corresponding modified code for “show_bytes”:
#include <stdio.h>
//Define variable "byte_pointer" in char datatype.
typedef unsigned char* byte_pointer;
//Function definition for show_bytes.
void show_bytes(byte_pointer start, size_t len)
{
//Declare variable "i" in int data type.
int i;
/* "For" loop to determine byte representation in hexadecimal */
for (i = 0; i < len; i++)
//Display each bytes in "2" digits hexadecimal value.
printf(" %.2x", start[i]);
printf("\n");
}
//Function to determine byte for "int" data type value.
void show_int(int x)
{
//Call show_bytes function with integer value.
show_bytes((byte_pointer) &x, sizeof(int));
}
//Function to determine byte for "float" data type value.
void show_float(float x)
{
//Call show_bytes function float value.
show_bytes((byte_pointer) &x, sizeof(float));
}
//Function to determine byte for "pointer" value.
void show_pointer(void *x)
{
//Call show_bytes function with pointer value.
show_bytes((byte_pointer) &x, sizeof(void *));
}
//Function to determine byte for "short" data type value.
void show_short(short x)
{
//Call show_bytes function with short int value.
show_bytes((byte_pointer) &x, sizeof(short));
}
//Function to determine byte for "long" data type value.
void show_long(long x)
{
//Call show_bytes function with long value.
show_bytes((byte_pointer) &x, sizeof(long));
}
//Function to determine byte for "double" data type value.
void show_double(double x)
{
//Call show_bytes function with double value.
show_bytes((byte_pointer) &x, sizeof(double));
}
//Test all show bytes.
void test_show_bytes(int val)
{
//Define variables.
int ival = val;
float fval = (float) ival;
int *pval = &ival;
//Call function.
show_int(ival);
show_float(fval);
show_pointer(pval);
//Define variables.
short sval = (short) ival;
long lval = (long) ival;
double dval = (double) ival;
//Call function.
show_short(sval);
show_long(lval);
show_double(dval);
}
//Main function.
int main(int argc, char* argv[])
{
//Define the sample value.
int sampleValue = 1024;
//Call test_show_bytes function.
test_show_bytes(sampleValue);
return 0;
}
The given program is used to display the byte representation of different program objects by using casting...

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Systems: Program... -Access
- Write a C program to check whether a given string is palindrome or not, using pointers. Every line in the code should be commented and the code should be well explained.arrow_forwardWrite a program in C that will add the numbers from 1 up to and including a given positive integer, ignoring all numbers which are evenly divisible by 3 or 5arrow_forwardWrite a program in C++ that includes two different enumeration types and has asignificant number of operations using the enumeration types. Give the source code inyour answer.arrow_forward
- Write a program in C++ using arrays, C-trings, classes, and libraries. Write a program to handle a user's rolodex entries. (A rolodex is a system with tagged cards each representing a contact. It would contain a name, address, and phone number. In this day and age, it would probably have an email address as well.) Typical operations people want to do to a rolodex entry are: 1) Add entry 2) Edit entry 3) Delete entry 4) Find entry 5) Print all entries 6) Quit You can decide what the maximum number of rolodex entries is and how long each part of an entry is (name, address, etc.). When they choose to edit an entry, give them the option of selecting from the current rolodex entries or returning to the main menu — don't force them to edit someone just because they chose that option. Similarly for deleting an entry. Also don't forget that when deleting an entry, you must move all following entries down to fill in the gap. If they want to add an entry and the rolodex is full, offer them the…arrow_forwardYour task is to print the size of primitive data types in c Like int, float, double, chararrow_forwardWrite and execute a program in C++ that will count from 1 to 21 and print the count, and its cubed value, for each count. 1 1 2 8 3 27 etc..arrow_forward
- write a code in c++ to solve any question in bisection method numerically and take screenshot contains the codearrow_forwardDevelop a code in java, to do the double entry book, all information has to be save in txt file.arrow_forwardWrite a program in C++ which prints words of a string in reverse order for example if a user enter “I love programming ” it should print “ programming love I ”.arrow_forward
- Programming in JAVA (I asked this question earlier, and I was helped... in C... which I don't know how to use yet haha. How do I implement a file with sets of numbers, such as 0 4 2 6 0 9 1 5 to an adjacency matrix JAVA?arrow_forwardGood day please help me with his C PROGRAMMING and the topic is all about RECURSION..PLEASE give the output of the following codes and explain how we got that answer (TRACING)... Solution is also needed...PLEASE DO SEND YOUR ANSWER USING A PICTURE OF YOUR WRITTEN ANSWER PLEASE. SO I CAN EASILY UNDERSTAND IT.I WILL GIVE HELPFUL RATING AFTER..arrow_forwardWrite just the function count(String s) and write it in JAVAarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
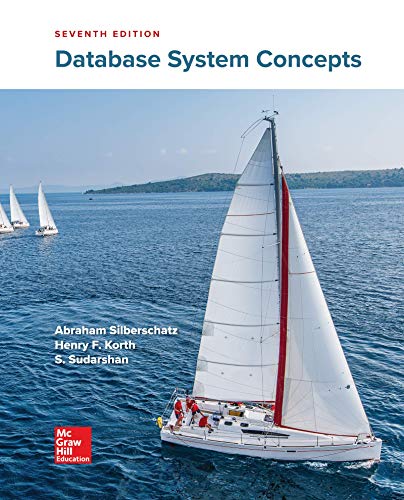
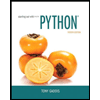
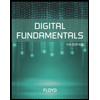
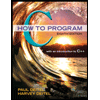
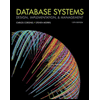
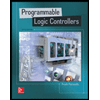