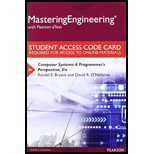
Explanation of Solution
Function definition for “replace_byte()” function:
The implementation for “replace_byte()” function is given below:
//Header file
#include <stdio.h>
#include <assert.h>
//Function definition for replace byte function
unsigned replace_byte(unsigned x, int i, unsigned char b)
{
//Check if value of "i" is less than "0", then
if (i < 0)
{
//Display error message
printf("error: value of i is negetive\n");
return x;
}
/* Check if value of "i" is greater than "sizeof(unsigned)-1", then */
if (i > sizeof(unsigned)-1)
{
//Display error message
printf("error: value of i is too high");
return x;
}
/* Assign the value of mask that is for byte
in general, 1 bye = 8 bits and then << implies *8 */
unsigned m = ((unsigned) 0xFF) << (i << 3);
//Defines the byte position
unsigned bytePosition = ((unsigned) b) << (i << 3);
//Return given value
return (x & ~m) | bytePosition;
}
//Main function
int main(int argc, char *argv[])
{
/* Call replace_byte function for replace byte 2 in given "x" by byte "0xAB" */
unsigned replaceByte2 = replace_byte(0x12345678, 2, 0xAB);
/* Call replace_byte function for replace byte 0 in given "x" by byte "0xAB" */
unsigned replaceByte0 = replace_byte(0x12345678, 0, 0xAB);
/* Check if the replaced byte equals to given value using "assert" function */
assert(replaceByte2 == 0x12AB5678);
assert(replaceByte0 == 0x123456AB);
return 0;
}
The given code is used to return an unsigned value in which byte “i” of argument “x” has been replaced by “b”...

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Systems: Program... -Access
- I want to write C or C++ functions that evaluate to ONE when the given conditions are true, and to ZERO when they are false. The following are the fourt conditions: int a(int x); //Any bit of x equals 0. int b(int x); // Any bit of x equals 1. int c(int x); //Any bit in the LSB of x equals 0. int d(int x); //Any bit in the MSB of x equals 1. The code should follow the bit-level integer coding rules, with the additional restriction that you may not use equality (==) or inequality (!=) tests.arrow_forwardWrite a function setbits(x,p,n,y) that returns x with the n bits that begin at position p set to the rightmost n bits of y, leaving the other bits unchanged.arrow_forwardWrite a C-function with two arguments (n and r) that has prototype: char clearbit(char k, char bits) The function clears (sets to 0) the bit number k (in the range of 0 to 7) in bits and returns the resulting value. For example, if k is 0x02 and bits is 0x07, the function would return bits with its k’th bit cleared, resulting in 0x03. It must not change other bits in bits. Hint: You may use any number of C-statements, but this task can be accomplished in as few as one!arrow_forward
- Write a function int bitset(int x, int n) to set nth bit of x to 1, if its (n-1)th bit is 1. if n <1 or n >15, the function will return -1 to thecalling functionarrow_forwardWrite a program that swaps 5th~11th bits in data_a with 25th~31th bits in data_bYour program must work for any data given, not just the example belowIn this question, we assume that the positions of bits count from right to left.That is, the first bit is the least significant bit.data_a DCD 0x77FFD1D1data_b DCD 0x12345678arrow_forwardImplement the function (in C or C++) with the following prototype: /** Implement a function which rotates a word left by n-bits, and returns that rotated value. Assume 0 <= n < w Examples: when x = 0x12345678 and w = 32: n=4 -> 0x23456781 n=20 -> 0x67812345 */ unsigned rotate_left(unsigned x, int n); The function should follow the bit-level integer coding rules above. Be careful of the case n = 0.arrow_forward
- Build a _lengOfSt function in MIPS, that takes an argument in $a1 which is the address of a null-terminated string, returning the length of the given string (number of characters excluding the null-character) in $v0.arrow_forwardThis exercise is about the bit-wise operators in C. Complete each functionskeleton using only straight-line code (i.e., no loops, conditionals, or functioncalls) and limited of C arithmetic and logical C operators. Specifically, youare only allowed to use the following eight operators: ! ∼, &,ˆ|, + <<>>.For more details on the Bit-Level Integer Coding Rules on p. 128/129 of thetext. A specific problem may restrict the list further: For example, to writea function to compute the bitwise xor of x and y, only using & |, ∼int bitXor(int x, int y){ return ((x&∼y) | (∼x & y));}(a) /*copyLSbit:Set all bits of result to least significant bit of x* Example: copyLSB(5) = 0xFFFFFFFF, copyLSB(6) = 0x00000000* Legal ops: ! ∼ & ˆ| + <<>>*/int copyLSbit(int x) {return 2;}(b) /* negate - return -x* Example: negate(1) = -1.* Legal ops:! ∼ & ˆ| + <<>>*/ int negate(int x) { return 2; }(c) /* isEqual - return 1 if x == y, and 0 otherwise*…arrow_forward// Task 3 // For this function, you must return the largest power of 2 that // is less than or equal to x (which will be positive). You may // not use multiplication or some sort of power function to do this, // and should instead rely on bitwise operations and the underlying // binary representation of x. If x is 0, then you should return 0. unsigned largest_po2_le(unsigned x) { return x; }arrow_forward
- 15. Consider the following Java-like code: int number = <<read number from user>>; int mask = MASK; int result = number OP mask; if (result != 0) { print("Bit 8 was set"); } The above code is supposed to print “Bit 8 was set” if bit 8 of user’s number was set to 1. If bit 8 was not set, then this code should not print anything (result==0). What hexadecimal value should MASK be, and what bit-wise operation should OP be, for the above code to work correctly?arrow_forwardI could use some help converting this problem into code for IA32 x86 Assembly floatIsEqual - Compute f == g for floating point arguments f and g. Both the arguments are passed as unsigned int's, but they are to be interpreted as the bit-level representations of single-precision floating point values. If either argument is NaN, return 0 +0 and -0 are considered equal.arrow_forwardConsider the following code which includes a parity check for the third digit: C=(000,011,101,110). You get the third digit by adding the first two and then using the remainder on division by 2. Can this code detect and count single errors? how can this be implemented?arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
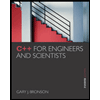