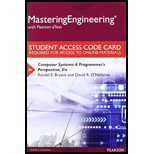
You have been assigned the task of writing a C function to compute.a floating-point representation of 2X. You decide that the best way to do this is to directly construct the IEEE single-precision representation of the result. When x is too small, your routine will return 0.0. When x is too large, it will return +∞. Fill in the blank portions of the code that follows to compute the correct result. Assume the function u2f returns a floating-point value having an identical bit representation as its unsigned argument.
Float fpwr2(int x)
{
/* Result exponent and fraction */
unsigned exp, frac;
unsigned u;
if (x <____________) {
/* Too small. Return 0.0 */
exp = _________;
frac = _________;
} else if (x < _______) {
/* Denormalized result */
exp = _________;
frac = _________;
} else if (x < _______) {
/* Normalized result. */
exp = _________;
frac = _________;
} else {
/* Too big. Return +∞ */
exp = _________;
frac = _________;
}
/* Pack exp and frac into ,32 bits */
u = exp << 23 | frac;
/* Return as float */
Return u2f (u);
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Systems: Program... -Access
Additional Engineering Textbook Solutions
Software Engineering (10th Edition)
Concepts of Programming Languages (11th Edition)
Programming in C
Starting Out with Java: Early Objects (6th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
- please provide the answer in C++ with simple step by step coding 9. (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters to 0, and then generate a large number of pseudorandom integers between 0 and 9. each time a 0 occurs, increment the variable you have designated as the zero counter; when a 1 occurs increment the counter variable that's keeping count of the 1s that occur; and so on. finally display the number of 0s, 1s, 2s, and so on that occurred and the percentage of the time they occurred.arrow_forwardWith c programming and without pointer and function Harry and Larry are now bored playing with numbers. Now they want to play with plus and minus signs. This time they don’t want to give signs one by one. Anyone can give any number of signs at a time but at most N signs. Can you tell the maximum of all signs that they gave sequentially? For example: If the input is 7 + + - + - - - , then the answer is 3 as 3 minus signs are given sequentially and it is the maximum. If the input is 7 + + + + + - - , then the answer is 5. Input Format First line will contain a positive integer N Second line will contain N signs (+ or -) Constraints 0 < N <= 100 Output Format Output a single integer, the maximum of all signs that came sequentially. Sample Input 0 7++++--- Sample Output 0 4arrow_forwardWrite C code that contains a function called triangle_generator that outputs a triangle wave at a specified frequency (in hertz) using a specified sample rate (in hertz), and for a specified time duration (in seconds). These parameters are float type. The output you generate are floating point numbers between -1 and 1, one number per output line. The math trig functions in C use radians while all the specifications here are in hertz (cycles per second). One hertz is 2*Pi radians.arrow_forward
- Consider the two versions of the function computing the combinations. Add a global variable counter and increment it by one at the top of both functions. This will count the number of function calls required to complete the calculations in each case. Try the two versions of the function for a few pairs of numbers n and m and print out the value of the counter after each of them. Don't forget to reset the counter to 0 before each call. Comment on the observed difference between the numbers of function calls.arrow_forwardWrite a C-function with two arguments (n and r) that has prototype: char clearbit(char k, char bits) The function clears (sets to 0) the bit number k (in the range of 0 to 7) in bits and returns the resulting value. For example, if k is 0x02 and bits is 0x07, the function would return bits with its k’th bit cleared, resulting in 0x03. It must not change other bits in bits. Hint: You may use any number of C-statements, but this task can be accomplished in as few as one!arrow_forwardI want to write C or C++ functions that evaluate to ONE when the given conditions are true, and to ZERO when they are false. The following are the fourt conditions: int a(int x); //Any bit of x equals 0. int b(int x); // Any bit of x equals 1. int c(int x); //Any bit in the LSB of x equals 0. int d(int x); //Any bit in the MSB of x equals 1. The code should follow the bit-level integer coding rules, with the additional restriction that you may not use equality (==) or inequality (!=) tests.arrow_forward
- Write a procedure is_little_endian that will return 1 when compiled and run on a little-endian machine, and will return 0 when compiled and run on a big-endian machine. This program should run on any machine, regardless of its word size. For this problem, write a function instead and call the function by sending it an integer. C program.arrow_forwardWrite a “C” program to find the summation of the following series: 1/8+ 1/27 + 1/64 + 1/125 + 1/216 + .............. + 1/(n+1)3Hint: To compute the power of a number, you can apply basic mathematics multiplications equations or use the pow() function computes the power of a number.The basic mathematics multiplications equations:For example: [In Mathematics] x4 = x*x*x*x [In programming]The pow() function:The pow() function takes two arguments (base value and power value) and, returns the power raised to the base number.For example, [In Mathematics] xy = pow(x, y) [In programming]The pow() function is defined in <math.h> header file (#include<math.h>)arrow_forwardWrite a Python function that computes the Fourier series expansion for a square wave for a given value of n. Ensure that your plot includes the expansion for n=1, 5 and 100. You should assume that h = 1, and T = 1 to simplify the task of plotting this series expansion.arrow_forward
- This exercise is about the bit-wise operators in C. Complete each functionskeleton using only straight-line code (i.e., no loops, conditionals, or functioncalls) and limited of C arithmetic and logical C operators. Specifically, youare only allowed to use the following eight operators: ! ∼, &,ˆ|, + <<>>.For more details on the Bit-Level Integer Coding Rules on p. 128/129 of thetext. A specific problem may restrict the list further: For example, to writea function to compute the bitwise xor of x and y, only using & |, ∼int bitXor(int x, int y){ return ((x&∼y) | (∼x & y));}(a) /*copyLSbit:Set all bits of result to least significant bit of x* Example: copyLSB(5) = 0xFFFFFFFF, copyLSB(6) = 0x00000000* Legal ops: ! ∼ & ˆ| + <<>>*/int copyLSbit(int x) {return 2;}(b) /* negate - return -x* Example: negate(1) = -1.* Legal ops:! ∼ & ˆ| + <<>>*/ int negate(int x) { return 2; }(c) /* isEqual - return 1 if x == y, and 0 otherwise*…arrow_forwardQ1.What is the smallest 32-bit floating point number f such that 128 + f > 128 ? What is the smallest 32-bit floating point number g such that 1 + g > 1 ? What is the relationship between f and g? Where f and g are differentiable functions. We have found that the given functions have the same derivative. In general, if two differentiable functions differs by a constant at x, its derivatives slopes at this point are the same. Write a short C++ or Python program to support your answer (for example, show that you checked whether there is no smaller number than your f by trying 128+f/2 == 128. Q2. Explain what changes are needed to change the 4x3 memory presented on Slide 62 of Chapter 3 to a 16x6 memory.arrow_forwardWrite a program to calculate the function sin (x) or cos (x) by developing into a Taylor series around point 0. In other words, you will program the sine or cosine function yourself, without using another existing solution. You can enter angles in degrees or radians. The program must work for any inputs, e.g. -4500 ° or + 8649 °. The function will have two arguments: float sinus (float radians, float epsilon); Use one of the following relationships for your own implementation (just program either sine or cosine, you don't need both): In C language plz . Thank youarrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
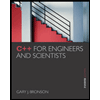