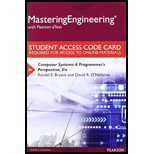
We are running
We generate arbitrary integer values x, y, and z, and convert them to values of type double as follows:
/* Create some arbitrary values */
int x = random ();
int y = random ();
int z = random ();
/* Convert to double */
double dx = (double) x;
double dy = (double) y;
double dz = (double) z;
For each of the following C expressions, you are to indicate whether or not the expression always yields 1. If it always yields 1, describe the underlying mathematical principles. Otherwise, give an example of arguments that make it yield 0. Note that you cannot use an IA32 machine running GCC to test your answers, since it would use the 80-bit extended-precision representation for both float and double.
A. (float) x == (float) dx
B. dx - dy == (double) (x-y)
C. (dx + dy) + dz == dx + (dy + dz)
D. (dx * dy) * dz == dx * (dy * dz)
E. dx / dx == dz/dz

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Systems: Program... -Access
Additional Engineering Textbook Solutions
Programming in C
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Using MIS (10th Edition)
C Programming Language
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- Write a program that swaps 5th~11th bits in data_a with 25th~31th bits in data_bYour program must work for any data given, not just the example belowIn this question, we assume that the positions of bits count from right to left.That is, the first bit is the least significant bit.data_a DCD 0x77FFD1D1data_b DCD 0x12345678arrow_forwardWrite a program that asks the user to enter an unsigned number and read it. Then swap the bits at odd positions with those at even positions and display the resulting number. For example, if the user enters the number 9, which has binary representation of 1001, then bit 0 is swapped with bit 1, and bit 2 is swapped with bit 3, resulting in the binary number 0110. Thus, the program should display 6. using MIPS assembly codearrow_forwardWrite a program that asks the user to enter a signed number and read it. Then display the content of multiplying this number by 5. 2. Write a program that asks the user to enter an unsigned number and read it. Then swap the bits at odd positions with those at even positions and display the resulting number. For example, if the user enters the number 9, which has binary representation of 1001, then bit 0 is swapped with bit 1, and bit 2 is swapped with bit 3, resulting in the binary number 0110. Thus, the program should display 6. #tow question using MIPSarrow_forward
- Write a program that having as an input a positive integer n, lists all the bit sequences of length n that do not have a pair of consecutive 0s.arrow_forwardConsider the following code which includes a parity check for the third digit: C=(000,011,101,110). You get the third digit by adding the first two and then using the remainder on division by 2. Can this code detect and count single errors? how can this be implemented?arrow_forwardIn c++ Now write a program for a double floating pointtype.•What are the number of significant bits that the double’s mantissa can hold, excluding the sign bit.•What is the largest number that a double’s mantissa can hold without roundoff error?•Repeat the program shown on the previous page but set to show where the double’s mantissa starts to exhibit roundoff errors.arrow_forward
- write a c++ program: Given a set of numbers where all elements occur even number of times except one number, find the odd occurring number” This problem can be efficiently solved by just doing XOR of all numbers.2- Use bitwise operators to compute division and multiplication by a number that is power of 2. For example: bitwise_divide/multiply(num, 8/16/32/2/4/128) should use only bitwise operators to compute result.3- Write code that checks if a number is odd or even using bitwise operators.4- Write a program that checks if a number is positive/negative/zero.5- Write a function that returns toggle case of a string using the bitwise operators in place.arrow_forwardWrite a MIPS procedure that takes as its three parameters the address of a zero-terminated string, a character c, and an integer n, and returns the number of distinct runs of consecutive instances of c in the string that are of length at least n. Also, write a simple main program to test your procedure. Your main program should input a string from the user (you may assume that the string has at most 20 characters), input the character and the integer, invoke your procedure, output the return value, and then terminate. For example, given the inputs “bbabbbbagbgbb”, “b”, and 2, the output should be 3. Your code must use the “standard” conventions covered in class for passing parameters and returning results. code in mipsarrow_forwardWrite a function sumSquare in RISC-V that, when given an integer n, returns the odd-number summation. If n is not positive, then the function returns 0. .data n: .word 7 .text main: la t0, n lw a0, 0(t0) jal ra, sumSquare addi a1, a0, 0 addi a0, x0, 1 ecall # Print Result addi a0, x0, 10 ecall # Exit sumSquare: #YOUR CODE HERE square: mul a0, a0, a0 jr raarrow_forward
- Implement the function (in C or C++) with the following prototype: /** Implement a function which rotates a word left by n-bits, and returns that rotated value. Assume 0 <= n < w Examples: when x = 0x12345678 and w = 32: n=4 -> 0x23456781 n=20 -> 0x67812345 */ unsigned rotate_left(unsigned x, int n); The function should follow the bit-level integer coding rules above. Be careful of the case n = 0.arrow_forwardWrite a program that swaps 5th~11th bits in data_a with 25th~31th bits in data_bYour program must work for any data given, not just the example belowIn this question, we assmue that the positions of bits count from right to left.That is, the first bit is the least significant bit.arrow_forwardimplement anyEvenBit(x) Return 1 if any even bit in x is set to 1 you are only allowed to use the following eight operators: ! ~ & ^ | + << >> “Max ops” field gives the maximum number of operators you are allowed to use to implement each function /* * anyEvenBit - return 1 if any even-numbered bit in word set to 1* Examples anyEvenBit(0xA) = 0, anyEvenBit(0xE) = 1* Legal ops: ! ~ & ^ | + << >>* Max ops: 12*/int anyEvenBit(int x) {return 2;}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
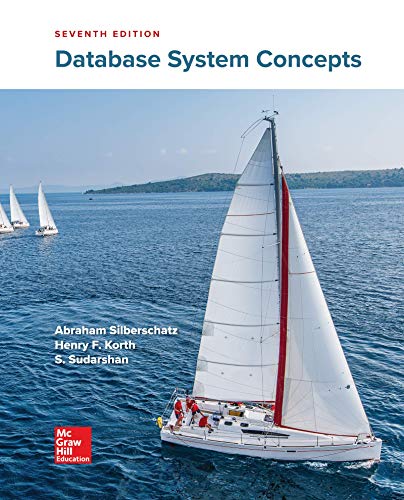
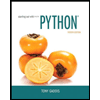
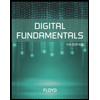
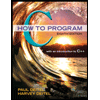
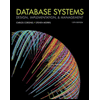
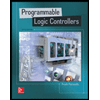