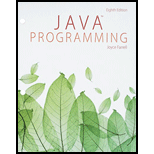
Explanation of Solution
Program code:
MyCharacter.java
//define a class MyCharacter
public class MyCharacter
{
//define the class member variables
private String name;
private int health;
private int power;
//constructor
public MyCharacter()
{
}
//constructor
public MyCharacter(String n, int h, int p)
{
//initialize the variables
name = n;
health = h;
power = p;
}
//define the method getName()
public String getName()
{
//return the value of name
return name;
}
//define the method setName()
public void setName(String name)
{
//set the value of name
this.name = name;
}
//define the method getHealth()
public int getHealth()
{
//return the value of health
return health;
}
//define the method setHealth()
public void setHealth(int health)
{
//set the value of health
this.health = health;
}
//define the method getPower()
public int getPower()
{
//return the value of power
return power;
}
//define the method setPower()
public void setPower(int power)
{
//set the value of power
this.power = power;
}
}
Explanation:
The above snippet of code is used to create a class “MyCharacter”. In the code,
- Import the required header files.
- Define a class “MyCharacter”
- Declare the class variables “name”, “health” and “power”.
- Define the constructor “MyCharacter”.
- Set the values of variables “name”, “health” and “power”.
- Define the “getName()” method.
- Return the value of “name”.
- Define the “setName()” method...

Trending nowThis is a popular solution!

Chapter 3 Solutions
Java Programming, Loose-leaf Version
- Create a simple java program that reads all the content in a .txt file, the user will enter the filename to be opened. In this case, the file will contain multiple lines of student details with each line containing a single student detail. Your task is to then create a student class that models the firstname, lastname, year of birth and gender of every student. The student class should further be able to assign every student created with a student number using a class variable[format is 2022000X, X is a number such as 1,2..] and be able to calculate the age of each student. Within the driver class add all the students read from file into an ArrayList and then print the first and last student in the list. You are additionally expected to deal with most common exceptionsarrow_forwardFor this exercise, you will create 2 classes: InputOutput class and AddNumbers class. ⦁ InputOutput Class: write a program that carries out the following tasks:⦁ Create an object of type PrintWriter and that writes into a file with the name greeting.txt.⦁ Print the message “Hello, world!” on the file.⦁ Close the file.⦁ Open the same file again.⦁ Read the message into a string variable (hint: use nextLine() method to read the text).⦁ Print the message on the screen. ⦁ AddNumbers Class: write a program that asks the user to input four floating-point numbers. Add all correctly specified values and print the sum when the user is done entering data. Use exception handling to detect improper inputs. For example, the user might enter letters instead of numbers. In this case, use the exception handling mechanism to print a proper message. (hint: use InputMismatchException) Don’t forget to import java.util.*.arrow_forwardI need to make a Java Application in Apache Netbeans 12.5 , I would like to ask for help please. The question is as follows: To encourage good grades, Hermosa High School has decided to award each student a bookstore credit that is 10 times the student’s grade point average. In other words, a student with a 3.2 grade point average receives a R 32 credit. Create a class that prompts a student for a name and grade point average, and then passes the values to a method that displays a descriptive message. The message uses the student’s name, echoes the grade point average, and computes and displays the credit. Save the application as BookstoreCredit.java.arrow_forward
- Please provide JAVA source code for following assignment. Please attach proper comments and read the full requirements. The local Driver’s License Office has asked you to write a program that grades the written portion of the driver’s license test The test has 20 multiple choice questions. Here are the correct answers: B D A A C A B A C D B C D A D C C D D A A student must correctly answer 15 of the 20 questions to pass the exam. Write a class named Drivertest that holds the correct answers to the test in an array field. The class should also have an array field that holds the student’s answers. The class should have the following methods: passed. Returns true if the student passed the test, or false if the student failed totalCorrect. Returns the total number of correctly answered questions totalIncorrect. Returns the total number of incorrectly answered questions questionsMissed. An int array containing the…arrow_forwardwrite program in java Create a Java Project With Your name as “EemanAsadProjectQNo1”, add a class to this Project, Class name should be Your Name with Your Reg No as “YourName18-arid-2860” Add a Method to Accept names and marks of nstudents from user, enter the value of nfrom Userb. Ensure that valid data is entered. If user enters an invalid data, then appropriate message should be displayed using Message Dialog Box e.g. ‘Name cannot be a number’ or ‘Marks must be an integer value’ Add another Method, to Display this data in descending order according to marks such that name of student having maximum marks should come at the top and the name of student having minimum marks should come at the bottom.arrow_forwardin java language You will write a method that will take a file name (String) as a parameter that is a file of numbers and will return the sum of the numbers in the file. You will then create a GUI with the following: 1. Some way for the user to specify a file name. 2. A mechanism for the user to begin processing the file. 3. Some place to display the sum of the numbers. keep everything in one class and andinclude the method that processes the file as a static method.arrow_forward
- ** in JAVA ** Write a Java program called ThreeWords that prompts (asks) the userto enter three words, separated by a space, on the same line and readin using the .nextLine( ) method of the Scanner class. You may NOTuse the .next( ) method of the Scanner class in this Task. Using the .next( ) method of the Scanner class will result in this Task being awarded 0, regardless of correct execution.(You may assume that the user always enters 3 words, each word separated by a single space.)Once the user has entered the three words, the program displays to the screen the longest word, that is, the word with the most characters. In the event of one or more of the words having the same number of characters, the program may decide which word it displays to the screen.arrow_forwardIn java, create a program that gathers user input and prints a paystub. This assignment requires one class per .java file, total of three classes. The first one is employee.java which gathers the user's id (fixed value), first name, last name, address, city, zip, phone, email, and hourly rate. The second one is payperiod.java, which gathers the user's hours worked (taking overtime into account), start date (fixed), end date (fixed), and paystub id (fixed). The third one is payrollmanager.java, which calculates and displays the data gathered from the first two. This class/file should also use methods such as: doubleCalculateGrossPay(Employee, PayPeriod), double CalculateRegularPay(Employee, PayPeriod), double CalculateOvertimePay(Employee, PayPeriod), and void PrintPaystub(Employee, PayPeriod).arrow_forwardThe extraterrestrials living in the planet Numerion revere a specific type of integers N. In particular, for those creatures, an integer N is holy if there exists an integer i >= 1 such that:N = i*i + iHere are some examples of holy numbers:2 (because 2 = 1*1 + 1) 6 (because 6 = 2*2 + 2) 12 (because 12 = 3*3 + 3) In a file called Numerion.java, write a program that: Asks the user to enter an integer N. It is OK if your program crashes when the user does not enter a valid integer. Prints out whether that number is a holy number in planet Numerion. For example: if the user enters 25, your program output should look EXACTLY like this: Enter an integer N: 25 25 is not a holy number in Numerion. Exiting... As another example: if the user enters 12 (a holy number, since 12 = 3*3 + 3), your program output should look EXACTLY like this:Enter an integer N: 12 12 is a holy number in Numerion. Exiting...arrow_forward
- Make a file named Rectangle.java, in that file create a following methods: //Ask the user for the length of the rectangle. public static double getLength(Scanner keyboard) //Ask the user for the width of the rectangle public static double getWidth(Scanner keyboard) //Calculate the area of the rectangle public static double getArea(double length, double width) //Display the information about the rectangle public static void displayData(double length, double width) Write the code in java and use test case # as an example result.arrow_forwardGiven the code below, write a Circle class (and save it in a file named Circle.java) that inherits from the Shape class. Include in your Circle class, a single private field double radius. Also include a method void setRadius(double r) (which also sets area) and a method double getRadius() (which also returns the current radius). Change the accessibility modifier for area in the Shape class to be more appropriate for a base class. Make sure that ShapeDriver's main() method executes and produces the following output: Shape: area: 78.53981633974483 radius: 5.0arrow_forwardPlease help me with this assignment below. I already have the code, you just need to fix something or add something in the code as what the assignment below requests. The assignment: Continue Exercise •• P13.1, checking the words against the /usr/share/dict/words on your computer, or the words.txt file in the companion code for this book. For a given number, only return actual words. The code of the resource class: import java.util.Scanner; public class U10P01R{ // This method to returns a character array containing all possible keypad // encodings for a digit in a standard phone char[] keysForButton(int n) { switch (n) { case 0: return new char[] { ' ' }; case 1: return new char[] { '.' }; case 2: return new char[] { 'A', 'B', 'C' }; case 3: return new char[] { 'D', 'E', 'F' }; case 4: return new char[] { 'G', 'H', 'I' }; case 5: return new char[] {…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
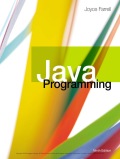