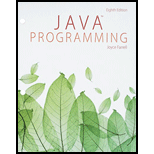
Concept explainers
Explanation of Solution
a.
Program code:
Sandwich.java
//create a class Sandwitch
public class Sandwich
{
//create string variables
private String ingredient, breadType;
//create double variable
private double price;
//define a constructor
public Sandwich()
{
}
//define a method getIngredient()
public String getIngredient()
{
//return the ingredient
return ingredient;
}
//define a method setIngredient()
public void setIngredient(String ingredient)
{
//set value of the variable ingredient
this.ingredient = ingredient;
}
//define a method getBreadType()
public String getBreadType()
{
//return the breadType
return breadType;
}
//define a method setBreadType()
public void setBreadType(String breadType)
{
//set value of the variable breadType
this.breadType = breadType;
}
//define a method getPrice()
public double getPrice()
{
//return the price
return price;
}
//define a method setPrice()
public void setPrice(double price)
{
//set value of the variable price
this.price = price;
}
}
Explanation:
The above snippet of code is used create a class “Sandwich”. The class contain different static methods for store the details of sandwich. In the code,
- Define a class “Sandwich”
- Define the constructor “Sandwich()” method.
- Define the “getIngredient()” method.
- Return the value of the variable “ingredient”.
- Define the “setIngredient()” method.
- Set the value of the variable “ingredient”.
- Define the “getBreadType()” method.
- Return the value of the variable “breadType”.
- Define the “setBreadType ()” method...

Trending nowThis is a popular solution!

Chapter 3 Solutions
Java Programming, Loose-leaf Version
- jada please Create a class named Purchase. Each Purchase contains an invoice number, amountof sale and sales tax. Include getter and setter methods for each property.Write a method called InvoiceTotal to compute the total of invoice. Save this infile called Purchase.javaCreate another class with the following:In this class, create a Purchase Object after prompting the user for necessarydetails.(You need to figure out what to ask the user for).When you prompt the user for invoice number do not let the user proceed unlessthe invoice number is between 5 and 55.For sales amount do not let the user proceed if they enter a negativevalue. After instantiating a Purchase object, show all the properties ofthe invoice object.arrow_forwarda. Create a class named Invoice that contains fields for an item number, name, quantity, price, and total cost. Create instance methods that set the item name, quantity, and price. Whenever the price or quantity is set, recalculate the total (price times quantity). Also include a displayLine() method that displays the item number, name, quantity, price, and total cost. Save the class as Invoice.java. b. Create a class named TestInvoice whose main() method declares three Invoice items. Create a method that prompts the user for and accepts values for the item number, name, quantity, and price for each Invoice. Then display each completed object. Save the application as TestInvoice.java.arrow_forwardPart A Create a class named Apartment that holds an apartment number as aptNumber, number of bedrooms as bedrooms, number of baths as baths, and rent amount as rent. Create a default constructor that accepts no arguments and an overloaded constructor that accepts values for each data field. Also create a get method for each field. Part B Write an application called TestApartments that creates at least five Apartment objects. Then prompt a user to enter a minimum number of bedrooms required, a minimum number of baths required, and a maximum rent the user is willing to pay. Display data for all the Apartment objects that meet the user’s criteria or an appropriate message if no such apartments are available. An example of the program is shown below: Enter minimum number of bedrooms needed >> 2 Enter minimum number of bathrooms needed >> 1.5 Enter maximum rent willing to pay >> 1200 Apartments meeting criteria of at least 2 bedrooms, at least 1.5 baths, and no more than…arrow_forward
- This is java question: Create a class named Bottle, which contains the following attributes: isFull (boolean), drinkType (ex: water), fillLevel (max: 100). Then, create a method called drink, which takes an argument of how much liquid the user drinks. When the user calls drink, subtract the amount drank from the fillLevel and make sure that isFull is set to false. Lastly, create a constructor that initializes the object as a full bottle containing water.arrow_forwardIn main(), prompt the user for two items and create two objects of the ItemToPurchase class. Before prompting for the second item, call scnr.nextLine(); to allow the user to input a new string.Ex: Item 1 Enter the item name: Chocolate Chips Enter the item price: 3 Enter the item quantity: 1 Item 2 Enter the item name: Bottled Water Enter the item price: 1 Enter the item quantity: 10arrow_forwardCreate a class IndependenceUSA and write code which asks the user to enter text that contains the American Independence day ( July 4th 1776). Read the date using the method next() 3 times. The user should enter the Independence day in the order month, day, year as shown in the first two runs below( we are not case sensitive) . Use the String method compareToIgnoreCase() to test the validi-ty of the date and print “Hurrah!” if the date entered is correct, or “No, no , no! Incorrect!” if the date incorrect is incorrect, or of the date entered in the wrong order. In other words, you compare your input to “July” , “4”, “1776” , in that order and you don't case for capital or small case letters.arrow_forward
- Create a class named BloodData that includes fields that hold a blood type (the four blood types are O, A, B, and AB) and an Rh factor (the factors are + and -). Create a default constructor that sets the fields to "O" and "+", and an overloaded constructor that requires values for both fields. Include get and set methods for each field. Save this file as BloodData.java. Create an application named TestBlood Data that demonstrates each method works correctly. Save the application as TestBloodData.java.arrow_forwardCreate a class named Checkup with fields that hold a patient number, two blood pressure figures (systolic and diastolic), and two cholesterol figures (LDL and HDL). Include methods to get and set each of the fields. Include a method named computeRatio() that divides LDL cholesterol by HDL cholesterol and displays the result. Include an additional method named explainRatio() that explains that HDL is known as “good cholesterol” and that a ratio of 3.5 or lower is considered optimum. Save the class as CheckupType.cpp. Create a tester program named TestCheckup whose main() method declares four Checkup objects. Call a getData() method four times. Within the method, prompt a user for values for each field for a Checkup, and return a Checkup object to the main() method where it is assigned to one of main()’s Checkup objects. Then, in main(), pass each Checkup object in turn to a showValues()method that displays the data. Blood pressure values are usually displayed with a slash between the…arrow_forwardWrite code to declare and create a Random class object (use the rand object reference variable). Then, using the nextInt method, create a list of expressions that produce random numbers in the following ranges, including the end points. Use the nextInt method's iteration that only takes an integer input.a. 0 to 10 b. 0 to 500c. 1 to 10d. 1 to 500e. 25 to 50f. -10 to 15 Write code to declare and create a Random class object (use the rand object reference variable). Then, using the nextInt method, create a list of expressions that produce random numbers in the following ranges, including the end points. Use the nextInt method's iteration that only takes an integer input.a. 0 to 10 b. 0 to 500c. 1 to 10d. 1 to 500e. 25 to 50f. -10 to 15arrow_forward
- Create a class named Purchase. Each Purchase contains an invoice number, amountof sale and sales tax. Include getter and setter methods for each property.Write a method called InvoiceTotal to compute the total of invoice. Save this infile called Purchase.javaCreate another class with the following:In this class, create a Purchase Object after prompting the user for necessarydetails.(You need to figure out what to ask the user for).When you prompt the user for invoice number do not let the user proceed unlessthe invoice number is between 5 and 55.For sales amount do not let the user proceed if they enter a negativevalue. After instantiating a Purchase object, show all the properties ofthe invoice object. Javaarrow_forwardModify class Time2 (pasted below) to include a tick method that increments the time stored in a Time2 object by one second. Provide method incrementMinute to increment the minute by one and method incrementHour to increment the hour by one. Write a program that tests the tick method, the incrementMinute method and the incrementHour method to ensure that they work correctly. Be sure to test the following cases: a. incrementing into the next minute, b. incrementing into the next hour and c. incrementing into the next day (i.e., 11:59:59 PM to 12:00:00 AM). public class Time2 {private int hour; // 0 - 23private int minute; // 0 - 59private int second; // 0 - 59 //no-argument constructor that initializes each instance variable to zeropublic Time2() {this(0, 0, 0); //invoke constructor with three arguments}//constructor, hour supplied, min and sec defaulted to 0public Time2(int hour) {this(hour, 0, 0); //invoke constructor}//hour and min suppliedpublic Time2(int hour, int minute)…arrow_forwardcreate a class named checkup with fields that you read a patient number, blood pressure figures (systolic or diastolic), and two cholesterol figures (ldl and hdl). include a method named computeratio() that divides ldl cholesterol by hdl cholesterol and displays the result. include an additional method named explainratio() that explains that hdl is knowns as “good cholesterol” and that a ratio of 3.5 or lower is considered optimum pseudo codearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
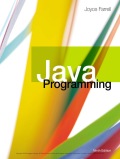
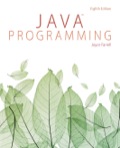
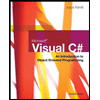