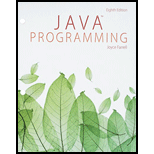
Explanation of Solution
a.
Program code:
Student.java
//define a class Student
class Student
{
//declare the class members
private String ID;
private int numberOfCredits;
private int points;
private double gradePoint;
//define the constructor
public Student()
{}
//define the constructor
public Student(String aID, int aNumberOfCredits, int aPoints)
{
//initialize the class members
super();
ID = aID;
numberOfCredits = aNumberOfCredits;
points = aPoints;
//call the method calculateGradePoint()
calculateGradePoint();
}
//define the method getID()
public String getID()
{
//return the value of ID
return ID;
}
//define the method setID()
public void setID(String aID)
{
//set the value of ID
ID = aID;
}
//define the method getNumberOfCredits()
public int getNumberOfCredits()
{
//return the value of numberOfCredits
return numberOfCredits;
}
//define the method setNumberOfCredits()
public void setNumberOfCredits(int aNumberOfCredits)
{
//set the value of numberOfCredits
numberOfCredits = aNumberOfCredits;
}
//define the method getPoints()
public int getPoints()
{
//return the value of points
return points;
}
//define the method setPoints()
public void setPoints(int aPoints)
{
//set the value of points
points = aPoints;
}
//define the method toString()
public String toString()
{
//return the value
return "ID : " + ID + " NumberOfCredits : " + numberOfCredits + " Points : " + points+" Grade Point : "+gradePoint;
}
//define the method calculateGradePoint()
public void calculateGradePoint()
{
//calculate the value of gradePoint
gradePoint=points/(double)numberOfCredits;
}
}
Explanation:
The above snippet of code is used create a class “Student”. The class contain different static methods for store the details of a student. In the code,
- Define a class “Student”
- Define the constructor “Student ()” method.
- Define the constructor “Student ()” method.
- Initialize the class members.
- Call the method “calculateGradePoint()”.
- Define the “getID()” method.
- Return the value of the variable “ID”.
- Define the “setID()” method.
- Set the value of the variable “ID”.
- Define the “getNumberOfCredits()” method.
- Return the value of the variable “NumberOfCredits”...

Trending nowThis is a popular solution!

Chapter 3 Solutions
Java Programming, Loose-leaf Version
- Following the example of the Rectangle class of Chapter 9 Exercise 1, design a class named Cuboid to represent a cuboid. The class contains: Three data fields named length, width, and height. A constructor that creates a cuboid with the specified length, width, and height. A method named getVolume() that returns the volume of this cuboid. Then, please create an object mycuboid = Cuboid (2, 3, 4), and invoke getVolume to print the volume of mycuboid.arrow_forwardWrite a class RangeInput that allows users to enter a value within a range of values that is provided in the constructor. An example would be a temperature control switch in a car that allows inputs between 60 and 80 degrees Fahrenheit. The input control has “up” and “down” buttons. Provide up and down methods to change the current value. The initial value is the midpoint between the limits. As with the preceding exercises, use Math.min and Math.max to limit the value. Write a sample program that simulates clicks on controls for the passenger and driver seats. Please ensure that it works with the test class provided.arrow_forwardGiven the code below, write a Circle class (and save it in a file named Circle.java) that inherits from the Shape class. Include in your Circle class, a single private field double radius. Also include a method void setRadius(double r) (which also sets area) and a method double getRadius() (which also returns the current radius). Change the accessibility modifier for area in the Shape class to be more appropriate for a base class. Make sure that ShapeDriver's main() method executes and produces the following output: Shape: area: 78.53981633974483 radius: 5.0arrow_forward
- a. Carly's Catering provides meals for parties and special events. In Chapters 3 and 4, you created an Event class for the company. Now, make the following changes to the class: Currently, the class contains a field that holds the price for an Event. Now add another field that holds the price per guest, and add a public method to return its value. Currently, the class contains a constant for the price per guest. Replace that field with two fields—a lower price per guest that is $32, and a higher price per guest that is $35. Add a new method named isLargeEvent() that returns true if the number of guests is 50 or greater and otherwise returns false. Modify the method that sets the number of guests so that a large Event (more than 50 guests) uses the lower price per guest to set the new pricePerGuest field and calculate the total Event price. A small Event uses the higher price. Save the file as Event.java. b. In Chapter 4, you modified the EventDemo class to demonstrate two Event…arrow_forwardcreate a class named checkup with fields that you read a patient number, blood pressure figures (systolic or diastolic), and two cholesterol figures (ldl and hdl). include a method named computeratio() that divides ldl cholesterol by hdl cholesterol and displays the result. include an additional method named explainratio() that explains that hdl is knowns as “good cholesterol” and that a ratio of 3.5 or lower is considered optimum pseudo codearrow_forwardCreate a class named Lease with fields that hold an apartment tenant’s name, apartmentnumber, monthly rent amount, and term of the lease in months. Include a constructor thatinitializes the name to “XXX”, the apartment number to 0, the rent to 1000, and the term to12. Also include methods to get and set each of the fields. Include a nonstatic methodnamed addPetFee() that adds $10 to the monthly rent value and calls a static methodnamed explainPetPolicy() that explains the pet fee. Save the class asLeaseYourlastname.java. Replace ‘Yourlastname’ with your real last name.b) Create a class named TestLease whose main() method declares four Lease objects. Call agetData() method three times. Within the method, prompt a user for values for each fieldfor a Lease, and return a Lease object to the main() method where it is assigned to one ofmain()’s Lease objects. Do not prompt the user for values for the fourth Lease object, but letit continue to hold the default values. Then, in main(), pass…arrow_forward
- For this lab task, you will work with classes and objects. Create a class named text that works similar to the built-in string class. You will need to define a constructor that can be used to initialize objects of this new class with some literal text. Next, define three methods: to_upper() that will convert all characters to uppercase, reverse() that will reverse the text and length() that will return the length of the text. After you have completed this part, copy the following mainfunction to your code and check if the implementation is correct. int main() { text sample = "This is a sample text"; cout << sample.to_upper(); // This should display "THIS IS A SAMPLE TEXT" cout << endl;cout << sample.reverse(); // This should display "txet elpmas a si sihT"cout << endl; cout << sample.length(); // This should display 21 }arrow_forwardThese two pictures are my resource and driver classes. Please, help me with the error in my resource class. I want my resource class to have getName, getSalary, getThePercentageThatTheEmployeeWantToRaise, and calculateTheNewSalary methods. For my driver class, I want the driver class to ask the user to input their name, salary, how much they want to raise, and print out their new salary.arrow_forwardI need to write a jave program to keep track of a team sport. Write a class, Team.java, that has the following: * two private instance variables: a String named color and an int named score. * a default constructor with no parameters that initializes color to "Red" and score to zero. * a constructor that takes two parameters (one for each instance variable) and sets the values of the instance variables. * a public getter and setter method for each instance variable. * a toString method that returns the color and score in the following format: "Red Team with 0 points."arrow_forward
- Create a class Course, which has one field: String courseName Create the constructor, accessor, and mutator for the class. Then, in the main method of this class, create an instance of the class with the name "CST1201". Write an equivalent while statement to replace the following for statement for (int i=2; i<100; i=i+2) { System.out.println(i); }arrow_forward(The Rectangle class) Following the example of the Circle class in Section 8.2,design a class named Rectangle to represent a rectangle. The class contains:■ Two double data fields named width and height that specify the width andheight of the rectangle. The default values are 1 for both width and height.■ A no-arg constructor that creates a default rectangle.■ A constructor that creates a rectangle with the specified width and height.■ A method named getArea() that returns the area of this rectangle.■ A method named getPerimeter() that returns the perimeter.Draw the UML diagram for the class and then implement the class. Write a testprogram that creates two Rectangle objects—one with width 4 and height 40and the other with width 3.5 and height 35.9. Display the width, height, area,and perimeter of each rectangle in this order.arrow_forwardGiven main(), define the Artist class (in file Artist.java) with constructors to initialize an artist's information, get methods, and a printInfo() method. The default constructor should initialize the artist's name to "unknown" and the years of birth and death to -1. printInfo() displays "Artist:", then a space, then the artist's name, then another space, then the birth and death dates in one of three formats: (XXXX to YYYY) if both the birth and death years are nonnegative (XXXX to present) if the birth year is nonnegative and the death year is negative (unknown) otherwise Define the Artwork class (in file Artwork.java) with constructors to initialize an artwork's information, get methods, and a printInfo() method. The default constructor should initialize the title to "unknown", the year created to -1. printInfo() displays an artist's information by calling the printInfo() method in Artist.java, followed by the artwork's title and the year created. Declare a private field of type…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
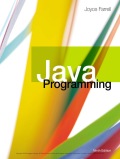
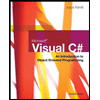