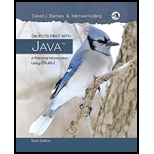
Concept explainers
Challenge exercise Implement a method in StockManager to print details of all products with stock levels below a given value (passed as a parameter to the method).
Modify the addProduct method so that a new product cannot be added to the product list with the same ID as an existing one.
Add to StockManager a method that finds a product from its name rather than its ID:
public Product findProduct (String name)
In order to do this, you need to know that two String objects, s1 and s2, can be tested for equality with the boolean expression
S1. equals (s2)
More details about comparing Strings can be found in Chapter 6.

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Additional Engineering Textbook Solutions
Java How To Program (Early Objects)
Starting Out with C++: Early Objects
Artificial Intelligence: A Modern Approach
Computer Science: An Overview (12th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- Implement solutions for the following methods: • getCourseSize() – returns the number of students registered in the course (not in the waitlist). It should maintain the public size variable that keeps track of the number of students registered. • getRegisteredIDs() – returns an array of int[], namely registered student id’s. The length of the array is the size (number of students) in the course. • getRegisteredStudents() – returns an array of type Student[], namely the registered Students. The length of the array is the current size (number of students) of the course. • getWaitlistedIDs() – returns an array of type int[], namely the ids of students in the waitlist. • getWaitlistedStudents() – returns an array of Students in the waitlist. public class Course { public String code; public int capacity; public SLinkedList<Student>[] studentTable; public int size; public SLinkedList<Student> waitlist; public Course(String code) {…arrow_forwardAdd a method (call it spotterAnimalArea) to AnimalLab that takes two parameters —animalType and areaID — and returns a String containing the IDs of spotters that haveseen the animal in that particular area. You should include only spotters who reported asighting with count greater than zero. You should exclude duplicate spotter IDs.import java.util.ArrayList;import java.util.List;import java.util.stream.Collectors; */public class AnimalLab{ private ArrayList<SightingRecord> records; private ArrayList<String > dubID; /** * Create an AnimalLab. */ public AnimalLab() { records = new ArrayList<>(); addSightingRecords("records.csv"); } /** * Add the sightings recorded in the given filename to the current list. * @param filename A CSV file of Sighting records. */ private void addSightingRecords(String filename) { SightingReader reader = new SightingReader();…arrow_forwardJust a few words on what a method is, and then we can move on to the three components.arrow_forward
- Implement keys() for SeparateChainingHashST and LinearProbingHashST. 0 Add a method to LinearProbingHashST that computes the average cost of a search hit in the table, assuming that each key in the table is equally likely to be sought.arrow_forwardCalculate the time complexity of the following issue and provide a method. Divide a list of n unique positive numbers into two sublists, each of size n/2, so that the difference in the sums of the integers in the two sublists is as large as possible. You may assume that n is a power of two.arrow_forwardAnswer the given question with a proper explanation and step-by-step solution. USING JAVA: ---Sorting the Invoices via dates--- Another error that will still be showing is that there is not Comparable/compareTo() method setup on the Invoice class file. That is something you need to fix and code. Implement the use of the Comparable interface and add the compareTo() method to the Invoice class. The compareTo() method will take a little work here. We are going to compare via the date of the invoice. The dates of the Invoice class are by default saved in a MM-DD-YYYY format. So they have a dash '-' between each part of the date. So you will need to split the date of the current invoice AND split the date of the object sent to the method. We have three things to compare against here. First we need to check the year. If the years are the same then you should go another step forward and compare the months. If the months are the same then you will lastly have to compare the day. Use whatever…arrow_forward
- Write the Java implementation of an instance method, called countRange, inside the IntArrayBag class such that the method takes two input integer parameters, called start and end. The method then counts and returns how many elements in the bag fall in the range between start and end inclusive. For example, if start is 5 and end is 8, then the method counts how many elements in the bag are equal to 5,6,7, or 8. The method returns zero if start > end. Make sure to include the method header.arrow_forwardHashMap is a parameterized class. List those of its methods that depend on the types used to parameterize it. Do you think the same type could be used for both of its parameters?arrow_forwardWrite a BlueJ project consisting in a class named Lecture whose goal is to store a list of the student names (of type String) attending it. In addition to declaring and initializing the necessary fields, the class has to implement: • A method boolean addStudent(String name) that adds a name to the (internal) list and returns an appropriate value (see Hint): the returned boolean value must be the same returned by the method add of the “internal list”. • A method int getHomonymNumber(String name) that returns the number of students that have the indicated name. • A method void printCSList() that prints the list of student names, in one single line and separated by a comma. For example: Goofy, Donald, Mickey Notice that you may not print a comma after the last name! • A method boolean swap(int index1, int index2) that swaps 2 students names in the list and returns true if the operation is successful. • A method void testIt() that adds at least 4 students (at least two with the same name),…arrow_forward
- Implement the design of the ECustomer class so that the following output is produced:[Your code should work for any number of products added in the setProductDetails method]# Write your codes here.print("Total E-Customer:", ECustomer.count)c1 = ECustomer("James")c1.setProductDetails("TV",35000,"Air Cooler", 9000)c2 = ECustomer("Mike")c2.setProductDetails("Mobile",20000,"Headphone",1200,"Fridge", 45000)c3 = ECustomer("Sarah")c3.setProductDetails("Headphone", 1200)print("=========================")c1.printDetail()print("=========================")c2.printDetail()print("=========================")c3.printDetail()print("=========================")print("Total E-Customer:", ECustomer.count)Output:Total E-Customer: 0=========================Name: JamesProducts: TV, Air CoolerTotal cost: 44000=========================Name: MikeProducts: Mobile, Headphone, FridgeTotal cost: 66200=========================Name: SarahProducts: HeadphoneTotal cost: 1200=========================Total E-Customer:…arrow_forwardConsider the BookArrayBag from slide #6 in the lecture-03-jan-28.pptx. Write an instance method belonging to BookArrayBag that takes one integer input, called year, and returns as output the number of books published in that year.arrow_forwardIn the existing class UnsortedTableMap, the method put(k,v) is used to add an entry to a map. This method spends time to locate an existing item with the given key. Provide a non-static method named putOnlyIfAbsent(k,v) that adds the entry to the map only if there is no entry with a key k. In case an entry with key k already exists, then just return the existing value corresponding to the key. The new method should be provided in the class UnsortedTableMap. Write the testing code in the main method of the class UnsortedTableMap.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
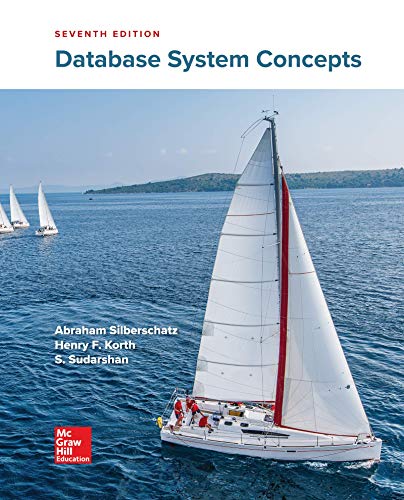
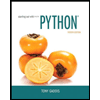
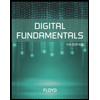
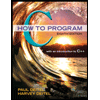
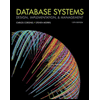
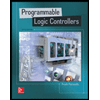