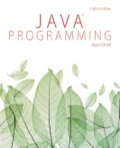
Explanation of Solution
Program:
File name: “Purchase.java”
//Define a class named Purchase
public class Purchase
{
//Declare the private variables
private int invoiceNumber;
private double saleAmount;
private double tax;
private static final double RATE = 0.05;
//Define a method to set the invoice number
public void setInvoiceNumber(int num)
{
invoiceNumber = num;
}
//Define a method to set the amount of sale
public void setSaleAmount(double amt)
{
saleAmount = amt;
//Calculate the amount of sales tax
tax = saleAmount * RATE;
}
/*Define a method to display the invoice number,
amount of sale, and amount of sales tax*/
public void display()
{
//Print the result
System.out.println("Invoice #" + invoiceNumber +
" Amount of sale: $" + saleAmount + " Tax: $" + tax);
}
}
File name: “PurchaseArray.java”
//Import necessary header files
import java.util.*;
//Define a class named PurchaseArray
public class PurchaseArray
{
//Define a main method
public static void main(String[] args)
{
//Declare a purchases object
Purchase[] purchases = new Purchase[5];
//Declare the variables
int num;
double amount;
String entry;
//Create an object for Scanner class
Scanner input = new Scanner(System.in);
//Declare the variables and initialize the values
int x;
final int LOW = 1000, HIGH = 8000;
//For loop to be executed until x exceeds 5
for(x = 0; x < purchases.length; ++x)
{
purchases[x] = new Purchase();
//Prompt the user to enter an invoice number
System.out.print("Enter invoice number >> ");
num = input...

Want to see the full answer?
Check out a sample textbook solution
- This is the question - In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Add get methods for the invoice number and sale amount fields so their values can be used in comparisons. Next, write a program that declares an array of five Purchase objects and prompt a user for their values. Then, in a loop that continues until a user inputs a sentinel value of Z, ask the user whether the Purchase objects should be sorted and displayed in invoice number order or sale amount order. This is the code I have. The programming isn't likeing what I have at all for the area where it says to write code - public class Purchase { private int invoiceNumber; private double saleAmount; private double tax; private static final double RATE = 0.05; public void setInvoiceNumber(int num) { invoiceNumber = num; } public void setSaleAmount(double amt) {…arrow_forwardWith reference to the classes defined question #1, write a method called identifyPersons() that receives an array of Person objects and then displays for each object the following information: name, birthdate, office hours (if the person is Faculty), title (if the person is Staff), class status (if the person is Student), the class name the object belongs to (Person, Employee, Student, Faculty, or Staff). Write a main method to test identifyPersons(). Make sure to call the method and pass array reference containing at least one object from each class.arrow_forwardThe local Driver’s License Office has asked you to write a program that grades the written portion of the driver’s license exam. The exam has 10 multiple-choice questions. Here are the correct answers: 1. B 2. D 3. A 4. A 5. C 6. A 7. B 8. A 9. C 10. D A student must correctly answer 7 of the 10 questions to pass the exam. Write a class named DriverExam that holds the correct answers to the exam in an array field. The class should also have an array field that holds the student’s answers. The class should have the following methods: • passed. Returns true if the student passed the exam, or false if the student failed. • totalCorrect. Returns the total number of correctly answered questions. • totalIncorrect. Returns the total number of incorrectly answered questions. • questionsMissed. An int array containing the question numbers of the questions that the student missed, i.e. answered incorrectly. Test the class in a separate class, DriverTest , that asks the user to enter a student’s…arrow_forward
- Create a class containing the main method. In the main method, create an integer array and initialize it with the numbers: 1,3,5,7,9,11,13,15,17,19 Pass the array as an argument to a method. Use a loop to add 1 to each element of the array and return the array to the main method. In the main method, use a loop to add the array elements and display the result. Note: In the loops, use the array field that holds the length of the array and do not use a number for array length.arrow_forwardThe local Driver’s License Office has asked you to write a program that grades the written portion of the driver’s license exam. The exam has 20 multiple choice questions. Here are the correct answers: B D A A C A B A C D B C D A D C C B D A A student must correctly answer 15 of the 20 questions to pass the exam. Write a class named DriverExam that holds the correct answers to the exam in an array field. The class should also have an array field that holds the student’s answers. The class should have the following methods: • passed. Returns true if the student passed the exam, or false if the student failed • totalCorrect. Returns the total number of correctly answered questions • totalIncorrect. Returns the total number of incorrectly answered questions • questionsMissed. An int array containing the question numbers of the questions that the student missed Demonstrate the class in a complete program that asks the user to enter a student’s answers, and then displays the…arrow_forwardA teacher has three exam grades for all the members of her class, and she worries that the grades are too low. You need to complete the method, named testAverage, in the class named Grades.java. There are two parameters to this method: the first is an integer representing the number of students in the class and, the second is a two-dimensional array of integers. In other words, each row represents the three grades for one student, while the columns represent the three sets of test grades. The return value should be the number of students with an average exam grade below seventy. The grades should be treated as double variables. For example, consider the grades for the four students in the following 2-D array, [88, 84, 89] [76, 64, 67] [85, 76, 79] [95, 90, 91] The first student has an exam average of 87.0 (= (88+84+89)/3 = 261/3), the second student has an exam average of 69.0 (= (77+64+67)/3 = 207/3), the third student has an exam average of 80.0 (= (85+76+79)/3 = 240/3), while the…arrow_forward
- A teacher has three exam grades for all the members of her class, and she worries that the grades are too low. You need to complete the method, named testAverage, in the class named Grades.java. There are two parameters to this method: the first is an integer representing the number of students in the class and, the second is a two-dimensional array of integers. In other words, each row represents the three grades for one student, while the columns represent the three sets of test grades. The return value should be the number of students with an average exam grade below seventy. The grades should be treated as double variables. For example, consider the grades for the four students in the following 2-D array, [ [88, 84, 89] [76, 64, 67] [85, 76, 79] [95, 90, 91] ] The first student has an exam average of 87.0 (= (88+84+89)/3 = 261/3), the second student has an exam average of 69.0 (= (77+64+67)/3 = 207/3), the third student has an exam average of 80.0 (= (85+76+79)/3 = 240/3), while…arrow_forwardCreate a class named Problem1, and create a main method, inside the main method prompt the user to enter a positive integer n. The program (Java) must error check (keep prompting and reading integers until you get a positive integer n). The program then will create an integer array arr of size n, and fill up that array with random integers between 1 and 500. - Create a method named getMax, which takes an integer array arr and returns an integer, the method should find the maximum value in the integer array arr. - Create a method named getMin, which takes an integer array arr and returns an integer, the method should find the minimum value in the integer array arr. - Create a method named sumValues, which takes an integer array arr and returns an integer, the method should find the sum of all values in the integer array arr. - Create a method named getAverage, which takes an integer array arr and returns a double, the method should find the average of all values in the integer array…arrow_forwardTHIS IS JAVA PROGRAMMING When the user enters 4 at the menu prompt, your program will access all objects in the array to calculate and display only the largest number of coin denomination, and the total number of coins for the largest denomination. If there are more than one coin denominations having equal amount, you will need to list down all of them. After processing the output for menu option 4, the menu is re-displayed. For example: Customer: Jane 65 cent Change: 50 cent: 1 10 cent: 1 5 cent: 1 Customer: John 75 cent Change: 50 cent: 1 20 cent: 1 5 cent: 1 Menu option 4 should return as follows.arrow_forward
- Use the Account class created in Programming Exercise9.7 to simulate an ATM machine. Create 10 accounts in an array with id 0,1, . . . , 9, and an initial balance of $100. The system prompts the user to enteran id. If the id is entered incorrectly, ask the user to enter a correct id. Once anid is accepted, the main menu is displayed as shown in the sample run. You canenter choice 1 for viewing the current balance, 2 for withdrawing money, 3 fordepositing money, and 4 for exiting the main menu. Once you exit, the systemwill prompt for an id again. Thus, once the system starts, it will not stop. Enter an id: 4 ↵EnterMain menu1: check balance2: withdraw3: deposit4: exitEnter a choice: 1 ↵EnterThe balance is 100.0Main menu1: check balance2: withdraw3: deposit4: exitEnter a choice: 2 ↵EnterEnter an amount to withdraw: 3 ↵EnterMain menu1: check balance2: withdraw3: deposit4: exitEnter a choice: 1↵EnterThe balance is 97.0arrow_forwardWrite an application that displays a series of at least five student ID numbers (that you have stored in an array) and asks the user to enter a numeric test score for the student. Create a ScoreException class, and throw a ScoreException for the class if the user does not enter a valid score (less than or equal to 100). Catch the ScoreException and then display an appropriate message. In addition, store a 0 for the student’s score. At the end of the application, display all the student IDs and scores. Save the files as ScoreException.java and TestScore.java.arrow_forwardThe assignment will be similar to an assignment used frequently in CS116 classes. You will be given an integer array of positive numbers. Your program should process the array and do the following for each entry: Determine if the number is odd or even. Add to a counter for each type, i.e. an even counter and an odd counter. Add the number to a sum for each type, i.e. a total of the odd numbers and a total of the even numbers. A value of -999 indicates the end of the array. Your program should end at that point. The input will be the following variable in your program: L3Array DCD 22,9,333,47,72,128,111,44,-999 The actual number of array entries and their values will be different when your program is graded. The last number will still be -999. The output of your program will be the counts and totals placed in the following variables: L3OddCt DCD 0 ; count of odd numbers L3OddTot DCD 0 ; total of odd numbers L3EvCt DCD 0 ; count of even numbers L3EvTot DCD 0 ; total of even…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
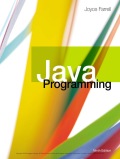
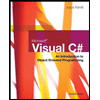