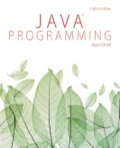
a.
Explanation of Solution
Program:
File name: “CollegeCourse.java”
//Define a class named CollegeCourse
public class CollegeCourse
{
//Declare the private variables
private String courseID;
private int credits;
private char grade;
//Define a get method that returns the course ID
public String getID()
{
//Return the value
return courseID;
}
//Define a get method that returns the credit hours
public int getCredits()
{
//Return the value
return credits;
}
//Define a get method that returns the letter grade
public char getGrade()
{
//Return the value
return grade;
}
//Define a set method that takes the course ID
public void setID(String idNum)
{
//Assign the value
courseID = idNum;
}
//Define a set method that takes the credit hours
public void setCredits(int cr)
{
//Assign the value
credits = cr;
}
//Define a set method that takes the letter grade
public void setGrade(char gr)
{
//Assign the value
grade = gr;
}
}
File name: “Student...
b.
Explanation of Solution
Program:
File name: “InputGrades.java”
//Import necessary header files
import college.CollegeCourse;
import college.Student;
import javax.swing.*;
//Define a class named InputGrades
public class InputGrades
{
//Define a main method
public static void main(String[] args)
{
//Declare an array to store data of 10 students
Student[] students = new Student[10];
//Declare the variables and initialize the values
int x, y, z;
String courseEntry, entry = "", message;
int idEntry, credits;
char gradeEntry = ' ';
boolean isGoodGrade = false;
final int NUM_COURSES = 5;
//Declare an array to store five grades
char[] grades = {'A', 'B', 'C', 'D', 'F'};
//For loop to be executed until x exceeds 10
for(x = 0; x < students.length; ++x)
{
//Create a student object
Student stu = new Student();
//Prompt the user to enter the student ID
entry = JOptionPane.showInputDialog(null, "For student #" +
(x + 1) + ", enter the student ID");
idEntry = Integer.parseInt(entry);
//Function call
stu.setID(idEntry);
//For loop to be executed until y exceeds 5
for(y = 0; y < NUM_COURSES; ++y)
{
//Prompt the user to enter the course
courseEntry = JOptionPane.showInputDialog(null,
"For student #" + (x + 1) + ", enter course #" +
(y + 1));
//Prompt the user to enter the credits for each //course
entry = JOptionPane.showInputDialog(null,
"For student #" + (x + 1) +
", enter credits for course #" + (y + 1));
credits = Integer.parseInt(entry);
//Assign the value false to isGoodGrade
isGoodGrade = false;
//While the user does not enter isGoodGrade
while(!isGoodGrade)
{
//Prompt the user to enter the grade for //course
entry = JOptionPane.showInputDialog(null,
"For student #" + (x + 1) +
", enter grade for course #" + (y + 1));
gradeEntry = entry...

Trending nowThis is a popular solution!

- a. Write a FractionDemo program that instantiates several Fraction objects and demonstrates that their methods work correctly. Create a Fraction class with fields that hold a whole number, a numerator, and a denominator. In addition: Create properties for each field. The set access or for the denominator should not allow a 0 value; the value defaults to 1. Add three constructors. One takes three parameters for a whole number, numerator, and denominator. Another accepts two parameters for the numerator and denominator; when this constructor is used, the whole number value is 0. The last constructor is parameterless; it sets the whole number and numerator to 0 and the denominator to 1. (After construction, Fractions do not have to be reduced to proper form. For example, even though 3/9 could be reduced to 1/3, your constructors do not have to perform this task.) Add a Reduce() method that reduces a Fraction if it is in improper form. For example, 2/4 should be reduced to 1/2. Add an operator+() method that adds two Fractions. To add two fractions, first eliminate any whole number part of the value. For example, 2 1/4 becomes 9/4 and 1 3/5 becomes 8/5. Find a common denominator and convert the fractions to it. For example, when adding 9/4 and 8/5, you can convert them to 45/20 and 32/20. Then you can add the numerators, giving 77/20. Finally, call the Reduce() method to reduce the result, restoring any whole number value so the fractional part of the number is less than 1. For example, 77/20 becomes 3 17/20. Include a function that returns a string that contains a Fraction in the usual display format—the whole number, a space, the numerator, a slash (D, and a denominator. When the whole number is 0, just the Fraction part of the value should be displayed (for example, 1/2 instead of 0 1/2). If the numerator is 0, just the whole number should be displayed (for example, 2 instead of 2 0/3). b. Add an operator*() method to the Fraction class created in Exercise 11a so that it correctly multiplies two Fractions. The result should be in proper, reduced format. Demonstrate that the method works correctly in a program named FractionDemo2. c. Write a program named FractionDem03 that includes an array of four Fractions. Prompt the user for values for each. Display every possible combination of addition results and every possible combination of multiplication results for each Fraction pair (that is, each type will have 16 results).arrow_forwardThis is the question I am stuck on - A. Create a CollegeCourse class. The class contains fields for the course ID (for example, CIS 210), credit hours (for example, 3), and a letter grade (for example, A). Include get and set methods for each field. Create a Student class containing an ID number and an array of five CollegeCourse objects. Create a get and set method for the Student ID number. Also create a get method that returns one of the Student’s CollegeCourses; the method takes an integer argument and returns the CollegeCourse in that position (0 through 4). Next, create a set method that sets the value of one of the Student’s CollegeCourse objects; the method takes two arguments—a CollegeCourse and an integer representing the CollegeCourse’s position (0 through 4). B. Write an application that prompts a professor to enter grades for five different courses each for 10 students. Prompt the professor to enter data for one student at a time, including student ID and course data for…arrow_forwardCreate a class Car with parameters companyName, color, maxSpeed (Not more than 150) andcategory. Use appropriate data types and access specifiers. Use default constructor to create objectsof cars. Write methods to set and get the values of parameters of car. Write methods to find categoryof car and to display the complete details of car along with category. Create another class TestCarwhich creates array of n number of cars, uses appropriate set methods to set the values of parametersof every car. Display the details of each car. (If max speed is between 120 and 150 then category is“High speed” otherwise “Normal”) (JAVA)arrow_forward
- Create an Employee data type, and then create three employee objects. Each employee object has his/her name, employee ID, and balance. Set the first employee object’s name as “Lacey”, ID as 1234, and balance as 100; Set the second employee object’s name as “Dylan”, ID as 5678, and balance as 0; Set the third employee object’s name as “James”, ID as 9012, and balance as 50; Write a method for withdrawing money; Write a method for depositing money; Create one constructor for the objects Lacey and James, and one constructor for the object Dylan; Set the fields as private. Withdraw 50 on all three employees, and after that display each of their names, IDs, and balances.arrow_forward1. Create a class called Student, with the following attributes/variables:a. studentName (String)b. studentNumber (int)sc. regYear(long)d. faculty(String)e. department(String)2. Add the following methods in Student:a. accessors and mutators for all variablesb. toString methodi. Formulate your toString so that it only shows studentName,studentNumber and faculty3. Create a class called MyFriends, with the following attributes variables:a. studentArray(Student[])4. Add the following methods in MyFriends:a. fillArrayi. Using scanner ask how many students does the user want to inputii. Capture user input for all of his friends(Of course we are assuming all your friends would be similarUWC students, go ask around and meet new friends, alsopreferably not in the same Computer Science department … ifpossible … only if possible)b. maini. Call the fillArray methodii. Count how many Computer Science studentsiii. If half or more students are Computer Science students, thendisplay “You … need more…arrow_forwardPart A Create a class named Apartment that holds an apartment number as aptNumber, number of bedrooms as bedrooms, number of baths as baths, and rent amount as rent. Create a default constructor that accepts no arguments and an overloaded constructor that accepts values for each data field. Also create a get method for each field. Part B Write an application called TestApartments that creates at least five Apartment objects. Then prompt a user to enter a minimum number of bedrooms required, a minimum number of baths required, and a maximum rent the user is willing to pay. Display data for all the Apartment objects that meet the user’s criteria or an appropriate message if no such apartments are available. An example of the program is shown below: Enter minimum number of bedrooms needed >> 2 Enter minimum number of bathrooms needed >> 1.5 Enter maximum rent willing to pay >> 1200 Apartments meeting criteria of at least 2 bedrooms, at least 1.5 baths, and no more than…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
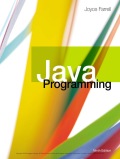
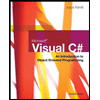