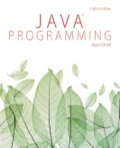
a.
Explanation of Solution
Program:
File name: “Die.java”
//Define a class named Die
public class Die
{
//Declare the private variables and initialize the value
private int value;
private static final int HIGHEST_DIE_VALUE = 6;
private static final int LOWEST_DIE_VALUE = 1;
//Define a Die method
public Die()
{
//Calculate the value
value = ((int)(Math.random() * 100) % HIGHEST_DIE_VALUE +
LOWEST_DIE_VALUE);
}
//Define a getValue method
public int getValue()
{
//Return the value
return value;
}
}
File name: “FiveDice2.java”
//Define a class named FiveDice2
public class FiveDice2
{
//Define a main method
public static void main(String[] args)
{
//Declare a variable and initialize the value
final int NUM = 5;
//Store the five dice rolled value for computer
Die[] comp = new Die[NUM];
//Store the five dice rolled value for player
Die[] player = new Die[NUM];
//Declare a variable
int x;
//For loop to be executed until x exceeds NUM
for(x = 0; x < NUM; ++x)
{
//Allocating memory for the array objects
comp[x] = new Die();
player[x] = new Die();
}
//Declare the variables
int compMatch, playerMatch;
//Get computer name
String computerName = "Computer";
//Get player name
String playerName = "You";
//Display the message
display(computerName, comp, NUM);
display(playerName, player, NUM);
//Calls the method to find same numbers for computer
compMatch = howManySame(comp, NUM);
//Calls the method to find same numbers for player
playerMatch = howManySame(player, NUM);
//If computer has one of a kind
if(compMatch == 1)
//Print the result
System.out.println("Computer has nothing");
//Else computer has a pair, three, four, or five of a //kind
else
//Print the result
System.out.println("Computer has " + compMatch + " of a kind");
//If player has one of a kind
if(playerMatch == 1)
//Print the result
System.out.println("You have nothing");
//Else player has a pair, three, four, or five of a kind
else
//Print the result
System.out.println("You have " + playerMatch + " of a kind");
//If any higher combination of computer beats a lower //one of player
if(compMatch > playerMatch)
//Print the result
System...
b.
Explanation of Solution
Program:
File name: “Die.java”
//Define a class named Die
public class Die
{
//Declare the private variables and initialize the value
private int value;
private static final int HIGHEST_DIE_VALUE = 6;
private static final int LOWEST_DIE_VALUE = 1;
//Define a Die method
public Die()
{
//Calculate the value
value = ((int)(Math.random() * 100) % HIGHEST_DIE_VALUE +
LOWEST_DIE_VALUE);
}
//Define a getValue method
public int getValue()
{
//Return the value
return value;
}
}
File name: “FiveDice3.java”
//Define a class named FiveDice3
public class FiveDice3
{
//Define a main method
public static void main(String[] args)
{
//Declare a variable and initialize the value
final int NUM = 5;
//Store the five dice rolled value for computer
Die[] comp = new Die[NUM];
//Store the five dice rolled value for player
Die[] player = new Die[NUM];
//Declare a variable
int x;
//For loop to be executed until x exceeds NUM
for(x = 0; x < NUM; ++x)
{
//Allocating memory for the array objects
comp[x] = new Die();
player[x] = new Die();
}
//Declare the variables
int compMatch, playerMatch;
int compHigh, playerHigh;
//Get computer name
String computerName = "Computer";
//Get player name
String playerName = "You";
//Display the message
display(computerName, comp, NUM);
display(playerName, player, NUM);
//Calls the method to find same numbers for computer
compMatch = howManySame(comp, NUM);
//Calls the method to find same numbers for player
playerMatch = howManySame(player, NUM);
//Compute the value
compHigh = compMatch / 10;
playerHigh = playerMatch / 10;
compMatch = compMatch % 10;
playerMatch = playerMatch % 10;
//If computer has one of a kind
if(compMatch == 1)
//Print the result
System.out.println("Computer has nothing");
//Else computer has a pair, three, four, or five of a //kind
else
//Print the result
System.out.println("Computer has " + compMatch + " of a kind");
//If player has one of a kind
if(playerMatch == 1)
//Print the result
System.out.println("You have nothing");
//Else player has a pair, three, four, or five of a kind
else
//Print the result
System.out.println("You have " + playerMatch + " of a kind");
//If any higher combination of computer beats a lower one of player
if(compMatch > playerMatch)
//Print the result
System.out.println("Computer wins");
//Else
else
//If any higher combination of player beats
//a lower one of computer
if(compMatch < playerMatch)
//Print the result
System.out.println("You win");
//Else
else
{
//If values of computer are greater than player's value
if(compHigh > playerHigh)
//Print the result
System...

Trending nowThis is a popular solution!

- In Chapter 2, you created a program for Marshalls Murals that prompts a user for the number of interior and exterior murals scheduled to be painted during the next month. The program computes the expected revenue for each type of mural when interior murals cost $500 each and exterior murals cost $750 each. The application should display, by mural type, the number of murals ordered, the cost for each type, and a subtotal. The application also displays the total expected revenue and a statement that indicates whether more interior murals are scheduled than exterior ones. Now create an interactive GUI program named MarshallsRevenueGUI that performs all the same tasks.arrow_forwardCreate a lottery game application named Lottery. Generate three random numbers, each between 1 and 4. Allow the user to guess three numbers. Compare each of the users guesses to the three random numbers, and display a message that includes the users guess, the randomly determined three-digit number, and the amount of money the user has won as follows: Â Make certain that your application accommodates repeating digits. For example, if a user guesses 1, 2, and 3, and the randomly generated digits are 1, 1, and 1, do not give the user credit for three correct guesses-just one.arrow_forwardA Costume shop requests a Computer application that advertizes Costume rentals. This week's Costume rental specials are as follows : Table Costume Price per week Renaissance Fair $40 Stormtrooper $49 Batman / Batgirl $36 Pirate $29 Write an application that allows the user to select any of the five costume rental specials. When the user selects a costume, the corresponding cost and a picture of the costume should be displayed. Clear each prior price and picture when the user selects a different costume. After selecting a costume, the user should be able to book the costume rental and then CLOSE the window. in visiual basic formarrow_forward
- (In Java) In the game Clacker, the numbers 1 through 12 are initially displayed. The player throws two dice and may cover the number representing the total or the two numbers on the dice. For example, for a throw of 3 and 5, the player may cover the 3 and the 5 or just the 8. Play continues until all the numbers are covered. The goal is to cover all numbers in the fewest rolls. Create a Clacker application that displays 12 buttons numbered 1 through 12. Each of these buttons can be clicked to ‘cover’ it. A covered button displays nothing. Another button labelled Roll can be clicked to roll the dice. Include labels to display the appropriate die images for each roll. Another label displays the number of rolls taken. A New Game button can be clicked to clear the labels and uncover the buttons for a new game.arrow_forwardYou are a manager of a Wally's Training Gym and you encourage your trainers to enroll new members. Input is the trainer's last name and the number of new enrollees, and names of each new member. Output is the number of trainers who have enrolled members in each of three categories: 0-5 new members, 6-10 new members, and 11 to 15 new members, as well as the names of each new enrollee. Write an application that allows the user to enter any number Trainer's names, number of new members, and names of each new member. Output is to display the names of trainers who are in each category, and the new members enrolled. Use good programming techniques and use appropriate variable names, use an array to store the names and number of new enrollees, and use prompts for the input and labels with the display. Write your program using Python. You may use a free version of Python at Replit. com Both header comments and step comments are encouraged as it will help for logic to be better. Header…arrow_forward(In Java) The SalesCenter application stores information about three employees. There is one manager (Diego Martin, salary $55,000), and two associates (Kylie Walter earning $18.50 per hour and Michael Rose earning $16.75 per hour). SalesCenter should be able to display the name and title for a specified employee. Additionally, the SalesCenter application should calculate and display the pay for a specified employee based on the pay argument entered by the user. The pay argument should correlate to hours worked if the pay for an associate is to be calculated. The pay argument for a manager should correlate to the number of weeks the manager is to be paid for. The SalesCenter interface should provide a menu of options. Depending on the option selected, additional input may be needed. Create client code that will generate the following SalesCenter output sketch: Employee/Pay/Quit Enter choice: E Enter employee number(1, 2, or 3): 2 Kylie Walter, associate Employee\Pay\Quit Enter…arrow_forward
- The Freemont Automobile Factory has discovered that the longer a worker has been on the job, the more parts the worker can produce. Write an application that computes and displays a worker’s anticipated output each month for 24 months assuming the worker starts by producing 4,000 parts and increases production by 6 percent each month. Also display the month in which production exceeds 7,000 parts (when the worker deserves a raise!) as follows: The month in which production exceeds 7000.0 is month X. I need the code.arrow_forwardThe date June 10, 1960, is special because when it is written in the following format, the month times the day equals the year: 6/10/60 Create an application that lets the user enter a month (in numeric form), a day, and a two-digit year. The program should then determine whether the month times the day equals the year. If so, it should display a message saying the date is magic. Otherwise, it should display a message saying the date is not magic.arrow_forwardCreate an application that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Do not display the computer’s choice yet.)2. The user selects his or her choice of rock, paper, or scissors. To get this input you can use Button controls, or clickable PictureBox controls displaying some of the artwork that you will find in the student sample files.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Rock smashes scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.)• If one player chooses…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
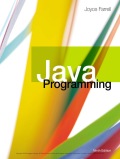
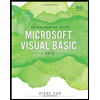
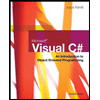
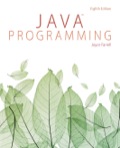