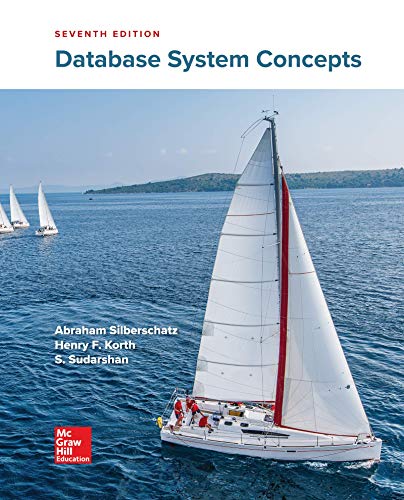
CAN AN EXPERT HELP FIX MY CODE
here is my code
import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.ArrayList; import java.util.Collections; import java.util.List; public class User implements Comparable<User> { private String username; private String password; public User(String username, String password) { this.username = username; this.password = password; } @Override public int compareTo(User other) { int cmp = this.password.length() - other.password.length(); if (cmp != 0) { return cmp; } cmp = this.password.toLowerCase().compareTo(other.password.toLowerCase()); if (cmp != 0) { return cmp; } return this.username.toLowerCase().compareTo(other.username.toLowerCase()); } @Override public String toString() { return String.format("%20s%20s", password, username); } public static void main(String args[]){ List<User> l= new ArrayList<>(); try{ BufferedReader br= new BufferedReader(new FileReader(new File("user-database.txt"))); String st, username, password; while((st=br.readLine())!=null){ username= st.split("\t")[0]; password= st.split("\t")[1]; l.add(new User(username,password)); } br.close(); BufferedReader in= new BufferedReader(new InputStreamReader(System.in)); Collections.sort(l); System.out.println("Enter a starting point and ending point"); String inp[]= in.readLine().trim().split(" "); int start= Integer.parseInt(inp[0]); int end= Integer.parseInt(inp[1]); for(int i=start;i<end;i++){ User u= l.get(i); System.out.println(u); } } catch(IOException e){ System.out.println(e.getMessage()); } } }
it should display
Enter a starting point and ending point\n
493 562ENTER
fpCqTjEfk cjacmardpr\n
gAmfepkQX cbidgodc4\n
GheSmEhte pianillipm\n
GMHoz13Ko lmcamishdl\n
Gr3yzaUYQ sheeranc8\n
Gv2zAbRx6 tcraddy8c\n
h7FUxSdUz rrosingb\n
HDLFE0tEN rfryd1l\n
HIlCcbD87 htongo8\n
hXB6xBgNW bfogdeneg\n
HzqustkgH mhansardpv\n
I44S3hCp9 ydranfield80\n
IE749MAQu dhouseman9a\n....
TJyeWJWUv bneamesmm\n
TLONniHHE aaltyok\n
tpXu8iQGW bwillimotcu\n
tQ8JiPSD5 ecurrorbu\n
however. it is off one line and producing an extra line in the beginning and lacks a line at the end and instead displays:
Enter a starting point and ending point\n
493 562ENTER
fMTEc9nPe smcconway7w\n
fpCqTjEfk cjacmardpr\n
gAmfepkQX cbidgodc4\n
GheSmEhte pianillipm\n
GMHoz13Ko lmcamishdl\n
Gr3yzaUYQ sheeranc8\n
Gv2zAbRx6 tcraddy8c\n
h7FUxSdUz rrosingb\n
HDLFE0tEN rfryd1l\n
HIlCcbD87 htongo8\n
hXB6xBgNW bfogdeneg\n
HzqustkgH mhansardpv\n
I44S3hCp9 ydranfield80\n
IE749MAQu dhouseman9a\n
IWA54iHTY ddongallp2\n...
TJyeWJWUv bneamesmm\n
TLONniHHE aaltyok\n
tpXu8iQGW bwillimotcu\n
i have tried changing
for(int i=start;i<end;i++){
User u= l.get(i);
System.out.println(u);
}
to
for(int i=start;i<=end;i++){
User u= l.get(i);
System.out.println(u);
}
and
for(int i=start-1;i<end;i++){
User u= l.get(i);
System.out.println(u);
}
and i have tried changing loop iterations
if (end > l.size()) {
end = l.size();
}
for (int i = start; i < end; i++) {
User u = l.get(i);
System.out.println(u);
}
but those solutions do not work, can another solution be provided to help fix my code, can an expert help fix my code so that it removes the first line in bold and adds the last line in bold to display correctly, I am just one line off

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- The main question is the entire page labeled Program #1, the little screenshot is for extra steps. The program has to be written in Java.arrow_forwardYou have to use comment function to describe what each line does import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class PreferenceData { private final List<Student> students; private final List<Project> projects; private int[][] preferences; private static enum ReadState { STUDENT_MODE, PROJECT_MODE, PREFERENCE_MODE, UNKNOWN; }; public PreferenceData() { super(); this.students = new ArrayList<Student>(); this.projects = new ArrayList<Project>(); } public void addStudent(Student s) { this.students.add(s); } public void addStudent(String s) { this.addStudent(Student.createStudent(s)); } public void addProject(Project p) { this.projects.add(p); } public void addProject(String p) { this.addProject(Project.createProject(p)); } public void createPreferenceMatrix() { this.preferences = new…arrow_forwardSolution question number 2arrow_forward
- StringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardimport org.firmata4j.IODevice;import org.firmata4j.Pin;import org.firmata4j.firmata.FirmataDevice;import org.firmata4j.ssd1306.SSD1306;import java.io.IOException;import java.util.HashMap;import java.util.TimerTask;public class minorproj extends TimerTask {static String recLog = "\"/dev/cu.usbserial-0001\"";static IODevice myGroveBoard;private final SSD1306 theOledObject;private Pin MoistureSensor;private Pin WaterPump;private Pin Button;private int sampleCount;public minorproj(SSD1306 theOledObject, Pin Button, Pin MoistureSensor, Pin WaterPump) {this.theOledObject = theOledObject;this.Button = Button;this.MoistureSensor = MoistureSensor;this.WaterPump = WaterPump;this.sampleCount = 0;}double startTime = System.currentTimeMillis();@Overridepublic void run() {while (true) {{// check if button is pressedif (this.Button.getValue() == 1) {break; // exit loop and stop program}String VolValue = String.valueOf(MoistureSensor.getValue());System.out.println("Moisture Sensor Value:" +…arrow_forwardimport java.io.BufferedReader;import java.io.File;import java.io.FileReader;import java.util.Scanner;public class romeoJuliet {public static void main(String[]args) throws Exception{Scanner keyboard=new Scanner(System.in);System.out.println("Enter a filename");String fN=keyboard.nextLine();int wLC=0, lC=0;String sW= keyboard.nextLine();File file=new File(fN);String [] wordSent=null;FileReader fO=new FileReader(fN);BufferedReader buff= new BufferedReader(fO);String sent;while((sent=buff.readLine())!=null){if(sent.contains(sW)){wLC++;}lC++;}System.out.println(fN+" has "+lC+" lines");System.out.println("Enter some text");System.out.println(wLC+" line(s) contain \""+sW+"\"");fO.close();}} Need to adjust code so that it reads all of the words that it was tasked to find. for some reason it isn't finding all of the word locations in a text. for example the first question is to find "the" in romeo and juliet which should have 1137, but it's only picking up 1032?arrow_forward
- import javax.swing.*; import java.util.ArrayList; import java.util.Collections; import java.util.Random; import java.awt.*; import java.awt.event.*; public class memory extends JFrame implements ActionListener { private JButton[] cards; private ImageIcon[] icons; private int[] iconIDs; private JButton firstButton; private ImageIcon firstIcon; private int numMatches; public memory() { setTitle("Memory Matching Game"); setSize(800, 600); setLayout(new BorderLayout()); JPanel boardPanel = new JPanel(new GridLayout(4, 4)); add(boardPanel, BorderLayout.CENTER); icons = new ImageIcon[8]; for (int i = 1; i <= 8; i++) { icons[i-1] = new ImageIcon("image" + i + ".png"); } iconIDs = new int[16]; for (int i = 0; i < 8; i++) { iconIDs[2*i] = i; iconIDs[2*i+1] = i; } Random rand = new Random(); for (int i = 0; i < 16;…arrow_forwardI need this in JAVAarrow_forwardCAN AN EXPERT HELP FIX MY CODE here is my code import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.ArrayList; import java.util.Collections; import java.util.List; public class User implements Comparable<User> { private String username; private String password; public User(String username, String password) { this.username = username; this.password = password; } @Override public int compareTo(User other) { int cmp = this.password.length() - other.password.length(); if (cmp != 0) { return cmp; } cmp = this.password.toLowerCase().compareTo(other.password.toLowerCase()); if (cmp != 0) { return cmp; } return this.username.toLowerCase().compareTo(other.username.toLowerCase()); } @Override public String toString() { return String.format("%20s%20s", password, username); } public static void main(String args[]){ List<User> l= new ArrayList<>(); try{ BufferedReader br= new…arrow_forward
- Run the program to tell me there is an error, BSTChecker.java:3: error: duplicate class: Node class Node { ^ BSTChecker.java:63: error: class LabProgram is public, should be declared in a file named LabProgram.java public class LabProgram { ^ 2 errors This is my program, please fix it. import java.util.*; class Node { int key; Node left, right; public Node(int key) { this.key = key; left = right = null; } } class BSTChecker { public static Node checkBSTValidity(Node root) { return checkBSTValidityHelper(root, null, null); } private static Node checkBSTValidityHelper(Node node, Integer min, Integer max) { if (node == null) { return null; } if ((min != null && node.key <= min) || (max != null && node.key >= max)) { return node; } Node leftResult = checkBSTValidityHelper(node.left, min, node.key); if (leftResult != null) { return leftResult; } Node rightResult = checkBSTValidityHelper(node.right, node.key, max); if (rightResult != null) { return rightResult; }…arrow_forwardMy problem is my code does not recognize the file path. CODE: Author.java package libraryDatabase; import java.util.Objects; public class Author { String firstName; public Author() { } String LastName; public Author(String firstName, String lastName) { this.firstName = firstName; LastName = lastName; } @java.lang.Override public java.lang.String toString() { return "Author{" + "firstName='" + firstName + '\'' + ", LastName='" + LastName + '\'' + '}'; } //this will be used for comparing two authors @Override public boolean equals(Object o) { if (this == o) return true; if (o == null || getClass() != o.getClass()) return false; Author author = (Author) o; return Objects.equals(firstName, author.firstName) && Objects.equals(LastName, author.LastName); } //this will be used when we compare two authors in search method @Override public int hashCode() { return Objects.hash(firstName, LastName); } } Book.java package libraryDatabase; public class Book { Author author; String…arrow_forwardIn Java, we can create an array of class objects. True O Falsearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
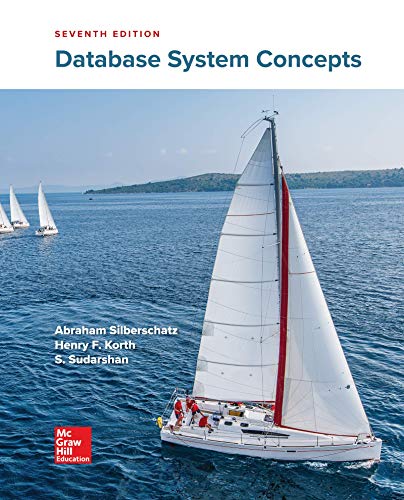
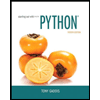
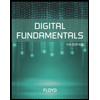
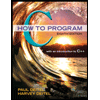
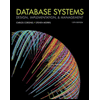
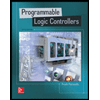