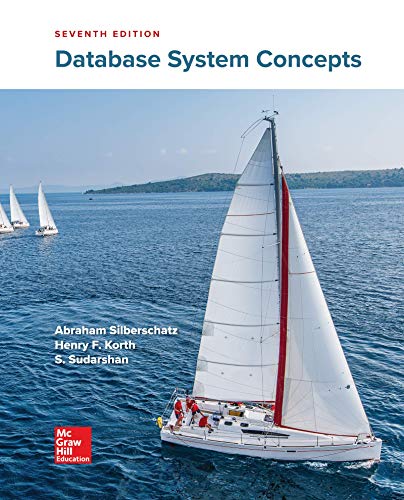
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Please complete the code and make sure it runs
package chapter04.queues;
public class LinkedQueue
{
protected PersonNode front;
protected PersonNode rear;
protected int numElements;
public LinkedQueue()
{
front = null;
rear = null;
numElements = 0;
}
public void enqueue(T fName, T lName, T sNum)
{
//Complete this method as required in the homework instructions.
}
public T dequeue()
{
//Complete this method as required in the homework instructions.
}
public void peekFront()
{
//Complete this method as required in the homework instructions.
}
public boolean contains(T lookFor)
{
//Complete this method as required in the homework instructions.
}
public void display()
{
//Complete this method as required in the homework instructions.
}
public int size()
{
return numElements;
}
public boolean isFull()
{
return false;
}
public boolean isEmpty()
{
return numElements == 0;
}
}
package chapter04.queues;
public class TestLinkedQueue
{
public static void main(String[] args)
{
LinkedQueue queue = new LinkedQueue();
System.out.println(queue.isEmpty());
/*
queue.enqueue("George", "Smith", "1901");
queue.enqueue("Eve", "Connor", "1783");
queue.enqueue("Else", "George", "1932");
queue.enqueue("Lina", "Wanner", "1245");
System.out.println();
queue.display();
System.out.println();
System.out.println("Empty? " + queue.isEmpty());
System.out.println();
System.out.println("Size: " + queue.size());
System.out.println();
String r = queue.dequeue();
System.out.println(r + " removed");
System.out.println();
queue.display();
*/
}
}
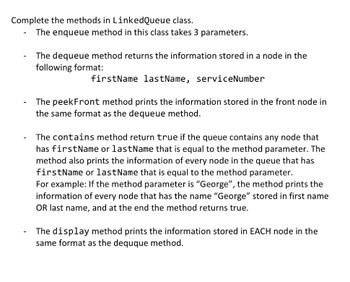
Transcribed Image Text:Complete the methods in LinkedQueue class.
-
The enqueue method in this class takes 3 parameters.
The dequeue method returns the information stored in a node in the
following format:
firstName lastName, serviceNumber
The peekFront method prints the information stored in the front node in
the same format as the dequeue method.
The contains method return true if the queue contains any node that
has firstName or lastName that is equal to the method parameter. The
method also prints the information of every node in the queue that has
firstName or lastName that is equal to the method parameter.
For example: If the method parameter is "George", the method prints the
information of every node that has the name "George" stored in first name
OR last name, and at the end the method returns true.
The display method prints the information stored in EACH node in the
same format as the dequque method.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- please fix code to match "enter patients name in lbs" thank you import java.util.LinkedList;import java.util.Queue;import java.util.Scanner; interface Patient { public String displayBMICategory(double bmi); public String displayInsuranceCategory(double bmi);} public class BMI implements Patient { public static void main(String[] args) { /* * local variable for saving the patient information */ double weight = 0; String birthDate = "", name = ""; int height = 0; Scanner scan = new Scanner(System.in); String cont = ""; Queue<String> patients = new LinkedList<>(); // do while loop for keep running program till user not entered q do { System.out.print("Press Y for continue (Press q for exit!) "); cont = scan.nextLine(); if (cont.equalsIgnoreCase("q")) { System.out.println("Thank you for using BMI calculator"); break;…arrow_forwardpackage lab1; /** * A utility class containing several recursive methods * * <pre> * * For all methods in this API, you are forbidden to use any loops, * String or List based methods such as "contains", or methods that use regular expressions * </pre> * * */ public final class Lab1 { /** * This is empty by design, Lab class cannot be instantiated */ privateLab1(){ // empty by design } /** * Returns the product of a consecutive set of numbers from <code> start </code> * to <code> end </code>. * * @param start is an integer number * @param end is an integer number * @return the product of start * (start + 1) * ..*. + end * @pre. * <code> start </code> and <code> end </code> are small enough to let * this method return an int. This means the return value at most * requires 4 bytes and no overflow would happen. */ publicstaticintproduct(ints,inte){ if(s==e){ returne; }else{ returns*product(s+1,e); } }…arrow_forwardpublic class OfferedCourse extends Course { // TODO: Declare private fields // TODO: Define mutator methods - // setInstructorName(), setLocation(), setClassTime() // TODO: Define accessor methods - // getInstructorName(), getLocation(), getClassTime() } import java.util.Scanner; public class CourseInformation { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Course myCourse = new Course(); OfferedCourse myOfferedCourse = new OfferedCourse(); String courseNumber, courseTitle; String oCourseNumber, oCourseTitle, instructorName, location, classTime; courseNumber = scnr.nextLine(); courseTitle = scnr.nextLine(); oCourseNumber = scnr.nextLine(); oCourseTitle = scnr.nextLine(); instructorName = scnr.nextLine(); location = scnr.nextLine(); classTime = scnr.nextLine(); myCourse.setCourseNumber(courseNumber); myCourse.setCourseTitle(courseTitle);…arrow_forward
- public class Ex08FanTest { public static void main (String[] args){ Ex08Fan fan1= new Ex08Fan(); Ex08Fan fan2= new Ex08Fan(); fan1.setOn(true); fan1.setSpeed(3); fan1.setRadius(10); fan1.setColor("yellow"); fan2.setOn(true); fan2.setSpeed(2); fan2.setRadius(5); fan2.setColor("blue"); fan2.setOn(false); System.out.println("Fan 1:\n" + fan1.toString()); System.out.println("\nFan 2:\n" + fan2.toString()); }}arrow_forwardRedesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardclass Queue { private static int front, rear, capacity; private static int queue[]; Queue(int c) { front = rear = 0; capacity = c; queue = new int[capacity]; } static void queueEnqueue(int data) { if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } else { queue[rear] = data; rear++; } return; } static void queueDequeue() { if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } else { for (int i = 0; i < rear - 1; i++) { queue[i] = queue[i + 1]; } if (rear < capacity) queue[rear] = 0; rear--; } return; } static void queueDisplay() { int i; if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } for (i = front; i < rear; i++) { System.out.printf(" %d <-- ", queue[i]); } return; } static void queueFront() { if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } System.out.printf("\nFront Element is: %d", queue[front]);…arrow_forward
- import java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forwardJava Given main(), complete the SongNode class to include the printSongInfo() method. Then write the Playlist class' printPlaylist() method to print all songs in the playlist. DO NOT print the dummy head node.arrow_forwardStarter code for ShoppingList.java import java.util.*;import java.util.LinkedList; public class ShoppingList{ public static void main(String[] args) { Scanner scnr=new Scanner(System.in); LinkedList<ListItem>shoppingList=new LinkedList<ListItem>();//declare LinkedList String item; int i=0,n=0;//declare variables item=scnr.nextLine();//get input from user while(item.equals("-1")!=true)//get inputuntil user not enter -1 { shoppingList.add(new ListItem(item));//add into shoppingList LinkedList n++;//increment n item=scnr.nextLine();//get item from user } for(i=0;i<n;i++) { shoppingList.get(i).printNodeData();//call printNodeData()for each object } }} class ListItem{ String item; //constructor ListItem(String item) { this.item=item; } void printNodeData() { System.out.println(item); }}arrow_forward
- import java.util.Scanner; public class Playlist { // TODO: Write method to ouptut list of songs public static void main (String[] args) { Scanner scnr = new Scanner(System.in); SongNode headNode; SongNode currNode; SongNode lastNode; String songTitle; int songLength; String songArtist; // Front of nodes list headNode = new SongNode(); lastNode = headNode; // Read user input until -1 entered songTitle = scnr.nextLine(); while (!songTitle.equals("-1")) { songLength = scnr.nextInt(); scnr.nextLine(); songArtist = scnr.nextLine(); currNode = new SongNode(songTitle, songLength, songArtist); lastNode.insertAfter(currNode); lastNode = currNode; songTitle = scnr.nextLine(); } // Print linked list…arrow_forwardPlease fill in all the code gaps if possible: (java) public class LinkedList { privateLinkedListNode head; **Creates a new instance of LinkedList** public LinkedList () { } public LinkedListNode getHead() public void setHead (LinkedListNode head) **Add item at the front of the linked list** public void insertFront (Object item) **Remove item at the front of linked list and return the object variable** public Object removeFront() }arrow_forwardExplain the following code : public static void printLeafNodes(TreeNode node) { // base case if (node == null) { return; } if (node.left == null && node.right == null) { System.out.printf("%d ", node.value); } printLeafNodes(node.left); printLeafNodes(node.right); }arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
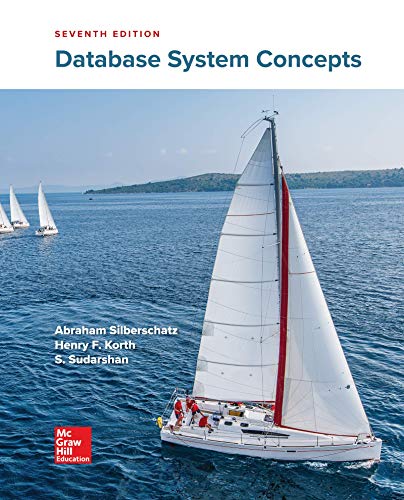
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
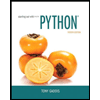
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
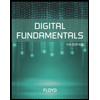
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
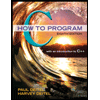
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
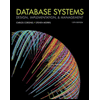
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
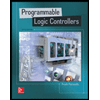
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education