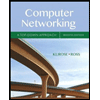
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
// interface method ==================================================
public Node get(int val) {
/*See BST.java for method specification */
/* Hint: Make sure you return a Node, not an int */
/* Your code here */
return null; // Dummy return statement. Remove when you implement!
}
FILL OUT METHOD AND ALL DESCRIPTIONS AND INFORMATION IS IN PICTURES PROVIDED!
IN JAVA LANGUAGE
PLS AND THANK YOU!!!!!!
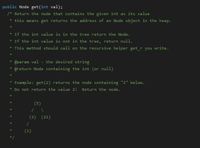
Transcribed Image Text:public Node get(int val);
/* Return the node that contains the given int as its value
* this means get returns the address of an Node object in the heap.
* If the int value is in the tree return the Node.
* If the int value is not in the tree, return null.
* This method should call on the recursive helper get_r you write.
@param val
the desired string
@return Node containing the int (or null)
* Example: get(2) returns the node containing "2" below.
Do not return the value 2!
Return the node.
(5)
(2) (21)
(1)
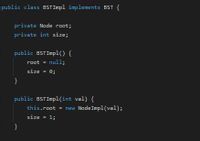
Transcribed Image Text:public class BSTIMP1 implements BST {
private Node root;
private int size;
public BSTImpl() {
root = null;
size = 0;
public BSTImpl(int val) {
this.root - new NodeImpl(val);
size = 1;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- VERY EASY RPN PROBLEM. read the directions please answer thank you public class Node <T>{ private T data; private Node<T> next; public Node(T data, Node<T> next) { this.data = data; this.next = next; } public T getData() { return data; } public Node<T> getNext() { return next; } } import java.util.Stack; public class StackArray<T> implements Stack<T> { private T [] elements; private int top; private int size; public StackArray (int size) { elements = (T[]) new Object[size]; top = -1; this.size = size; } public boolean empty() { return top == -1; } public boolean full() { return top == size - 1; } public boolean push(T el) { if (full()) return false; else { top++; elements[top] = el; return true; } }…arrow_forward@Override// interface method ==================================================public int merge(BST nbt) {/*See BST.java for method specification */// Hint: traverse bst using pre-order// as each node is visited, take the value there// and do this.insert(value)// have to somehow count when an add is successful// so we can return the number of nodes added/* Your code here */return 0; // Dummy return statement. Remove when you implement!} in java fill out method should merge two bst and not add duplicatesarrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forward
- I need an explanation for this program. class Directory { Node head,tail; Directory() { head=null; tail=null; } public void insert(Node node) // method that insers node into list if phone number is new { Node temp=head; while(temp!=null && !temp.phone_number.equals(node.phone_number)) temp=temp.next; if(temp!=null) { System.out.println("Entered phone number already exists"); return; } if(head==null) { head=node; tail=node; } else { tail.next=node; tail=node; } System.out.println("Inserted Successfully"); } public void remove(String ph_no) // method that removes node with given phone number { Node prev=null; Node node=head; while(node!=null && !node.phone_number.equals(ph_no)) { prev=node;…arrow_forwardPlease help in Python 3. Given code for BST.py ## Node classclass Node():def __init__(self, val):self.__value = valself.__right = Noneself.__left = Nonedef setLeft(self, n):self.__left = ndef setRight(self, n):self.__right = ndef getLeft(self):return self.__leftdef getRight(self):return self.__rightdef getValue(self):return self.__value # BST classclass BST():def __init__(self):self.__root = Nonedef append(self, val):node = Node(val)if self.__root == None:self.__root = nodereturncurrent = self.__rootwhile True:if val <= current.getValue():if current.getLeft() == None:current.setLeft(node)returnelse:current = current.getLeft()else:if current.getRight() == None:current.setRight(node)returnelse:current = current.getRight()def searchLength(self, val):if self.__root == None:return -1current = self.__rootlength = 0while current != None:if current.getValue() == val:return length + 1elif val < current.getValue():length += 1current = current.getLeft()else:length += 1current =…arrow_forwardi need it ASAP pleasearrow_forward
- write this code below as algorithim to determine the leaf node reclusively ? public static void printLeafNodes(TreeNode node) { // base case if (node == null) { return; } if (node.left == null && node.right == null) { System.out.printf("%d ", node.value); } printLeafNodes(node.left); printLeafNodes(node.right); }arrow_forwardPlease complete the code and make sure it runs package chapter04.queues; public class LinkedQueue { protected PersonNode front; protected PersonNode rear; protected int numElements; public LinkedQueue() { front = null; rear = null; numElements = 0; } public void enqueue(T fName, T lName, T sNum) { //Complete this method as required in the homework instructions. } public T dequeue() { //Complete this method as required in the homework instructions. } public void peekFront() { //Complete this method as required in the homework instructions. } public boolean contains(T lookFor) { //Complete this method as required in the homework instructions. } public void display() { //Complete this method as required in the homework instructions. } public int size() { return numElements; } public boolean isFull() { return false;…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
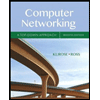
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
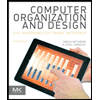
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
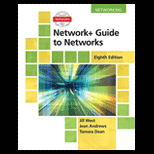
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
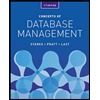
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
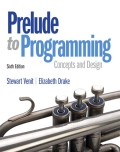
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
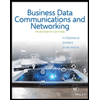
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY