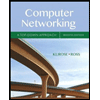
Hello , I'm attempting complete my Java code but I'm getting an error
import java.util.Scanner;
public class GradeCalculatorFinal.java{
public static void main(String[] args) {
// Total points / total credits
// points for a class = grade value * credits
// A = 4, B = 3, C = 2, D = 1....
Scanner scanner = new Scanner(System.in);
System.out.println("Enter grade:");
String grade = scanner.nextLine();
Integer points = 0;
Integer gradeValue = 0;
if (grade.equalsIgnoreCase("A")) {
gradeValue = 4;
} else if (grade.equalsIgnoreCase("B")) {
gradeValue = 3;
} else if (grade.equalsIgnoreCase("C")) {
gradeValue = 2;
} else if (grade.equalsIgnoreCase("D")) {
gradeValue = 1;
} else if (grade.equalsIgnoreCase("F")) {
gradeValue = 0;
} else {
System.out.println("You didn't enter a valid grade :(");
}
Integer points = gradeValue * Integer.parseInt(credits);
Integer gpa = points / Integer.parseInt(credits);
System.out.println("Credits: " + credits);
System.out.println("Grades: " + grades);
System.out.println("Points: " + points);
System.out.println("GPA: " + gpa);
scanner.close();
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- import java.util.Scanner; public class LabProgram { public static Roster getInput(){ /* Reads course title, creates a roster object with the input title. Note that */ /* the course title might have spaces as in "COP 3804" (i.e. use nextLine) */ /* reads input student information one by one, creates a student object */ /* with each input student and adds the student object to the roster object */ /* the input is formatted as in the sample input and is terminated with a "q" */ /* returns the created roster */ /* Type your code here */ } public static void main(String[] args) { Roster course = getInput(); course.display(); course.dislayScores(); }} public class Student { String id; int score; public Student(String id, int score) { /* Student Employee */ /* Type your code here */ } public String getID() { /* returns student's id */…arrow_forwardHelp me fix the code make it run out like the EXPEarrow_forwardGiven string inputStr on one line and integers idx1 and idx2 on a second line, output "Match found" if the character at index idx1 of inputStr is equal to the character at index idx2. Otherwise, output "Match not found". End with a newline. Ex: If the input is: eerie 4 1 then the output is: Match found Note: Assume the length of string inputStr is greater than or equal to both idx1 and idx2.arrow_forward
- import java.util.Scanner; public class CircleAndSphereWhileLoop{ public static final double MAX_RADIUS = 500.0; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Read a double value as radius using prompt // "Enter the radius (between 0.0 and 500.0, exclusive): " // Step 3: While the input radius is not in the ragne (0.0, 500.0) // Display a message on one line (ssuming input value -1) // "The input number -1.00 is out of range." // Read a double value as radius using the same promt double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 * Math.PI * Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); // Step 4: Display the radius, circle circumference, circle area, // sphere surface area, and…arrow_forwardJava - How can I output the following statement as a single line with a newline? Program below. import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); if(n>=11 && n<=100) { while(n>=11 && n<=100) { System.out.print(n+ " "); (the problem line. When I use System.out.println, it makes the line vertical when I want it horizontal.) if(n ==11||n==22||n==33||n==44||n==55||n==66||n==77||n==88||n==99) break; n--; } } else System.out.println("Input must be 11-100"); }}arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward
- import java.util.Scanner; public class AutoBidder { public static void main (String [] args) { Scanner scnr = new Scanner (System.in); char keepGoing; int nextBid; nextBid = 0; keepGoing 'y'; * Your solution goes here */ while { nextBid = nextBid + 3; System.out.println ("I'll bid $" + nextBid + "!") ; System.out.print ("Continue bidding? (y/n) "); keepGoing = scnr.next ().charAt (0); } System.out.println (""); }arrow_forward1 import java.util.Scanner; 2 3 public class RedBalloon { public static void main (String 0 args) { Scanner scnr = new Scanner(System.in); boolean isRed; boolean isBalloon; 4 5 6 7 8 isRed = scnr.nextBoolean(); isBalloon = scnr.nextBoolean(); 9 10 11 12 13 } 15 } 14arrow_forwardimport java.util.Scanner;public class main{public static void main(String[] args){Scanner sc = new Scanner(System.in):int year, day, weekday;String month;String outday = "";System.out.printf("Enter the month%n");month = sc.nextLine();System.out.printf("Enter the day%n");month = sc.nextInt();weekay = find_day(month, day);outday = out_weekday(weekday);System.out.printf("The day is: %s%n", outday);}} Change the program from the previous example to take command line arguments instead of using the Scanner. For example, running the program like this:> java OutDays March 14will output the weekday string (which is Thursday) on the console for March 14, 2019). public static void main(String[] args){ int year, day, weekday; String month; String outday = ""; // #### your code here for accessing the command line arguments weekday = find_day(month, day); outday = out_weekday(weekday); System.out.printf("The day is: %s%n", outday);}arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
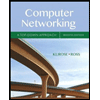
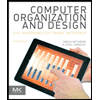
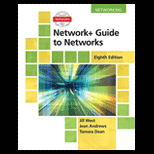
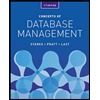
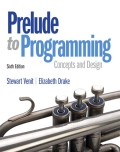
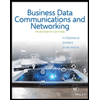