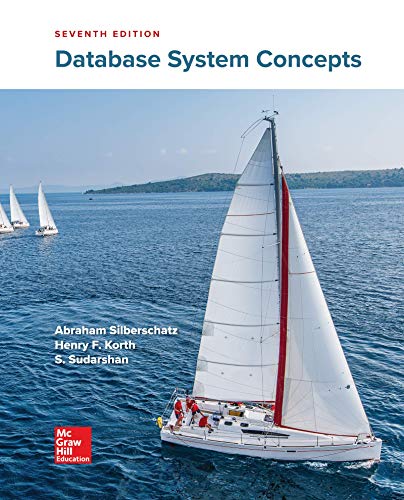
import java.util.Arrays;
import dsUtils.WordReader;
public class WordFrequencyAnalyzer {
/**********************************************************************************/
/* You are not allowed to add any fields to this class beyond the one given below */
/* You may only read in from the file once. This means you may only use a single */
/* word reader object. */
/**********************************************************************************/
// Currently, the field counters is not used.
// Your task is to make this class more efficent by storing the word counts
// as a symbol table / map / dictionary in the field counters.
private SequentialSearchST<String, Integer> counters;
private String filename;
/**
* Stores a count of the number of times any word appears in a file. The file is
* read in exactly once at the time time this object is constructed.
* (You need to modify the constructor to do this.)
*
* @param filename the name of the file on which to count all word occurrences.
*/
public WordFrequencyAnalyzer(String filename) {
this.filename = filename;
}
/**
* Returns the number of times a given word appears in the file from which this
* object was created.
* (Currently, it reads through the entire file to count the number of times
* the given word appears. Modify this to instead use the information stored
* in the counters field.)
*
* @param word the word to count
* @return the number of times <code>word</code> appears.
*/
public int getCount(char[] word) {
WordReader r = new WordReader(filename);
int count = 0;
for(char[] w : r) {
if (Arrays.equals(w, word))
count++;
}
return count;
}
/**
* Returns the maximum frequency over all words in the file from which this
* object was created.
* (Currently, this method is not implemented. You must implement it.)
*
* @return the maximum frequency of any word in the the file.
*/
public int maxCount() {
throw new RuntimeException("Not implemented.");
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

how/where do you insert the output?
how/where do you insert the output?
- Write code in Java: - Code must be recursive. import java.util.*; import java.lang.*; import java.io.*; class BinaryToDecimal { // *More methods can be added* public static int binaryToDecimal(String binaryString) { // *Code goes here* } } class DriverMain { public static void main(String args[]) { Scanner input = new Scanner(System.in); System.out.print(BinaryToDecimal.binaryToDecimal(input.nextLine())); } }arrow_forwardimport java.util.Arrays; import java.util.Random; public class Board { /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. That some of the possible moves * don't actually change what the board looks like. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { throw new RuntimeException("Not implemented"); } The board is 5 X 5. You can add classes and imports like rand.arrow_forwardpackage lab1; /** * A utility class containing several recursive methods * * <pre> * * For all methods in this API, you are forbidden to use any loops, * String or List based methods such as "contains", or methods that use regular expressions * </pre> * * */ public final class Lab1 { /** * This is empty by design, Lab class cannot be instantiated */ privateLab1(){ // empty by design } /** * Returns the product of a consecutive set of numbers from <code> start </code> * to <code> end </code>. * * @param start is an integer number * @param end is an integer number * @return the product of start * (start + 1) * ..*. + end * @pre. * <code> start </code> and <code> end </code> are small enough to let * this method return an int. This means the return value at most * requires 4 bytes and no overflow would happen. */ publicstaticintproduct(ints,inte){ if(s==e){ returne; }else{ returns*product(s+1,e); } }…arrow_forward
- *JAVA* complete method Delete the largest valueremoveMax(); Delete the smallest valueremoveMin(); class BinarHeap<T> { int root; static int[] arr; static int size; public BinarHeap() { arr = new int[50]; size = 0; } public void insert(int val) { arr[++size] = val; bubbleUP(size); } public void bubbleUP(int i) { int parent = (i) / 2; while (i > 1 && arr[parent] > arr[i]) { int temp = arr[parent]; arr[parent] = arr[i]; arr[i] = temp; i = parent; } } public int retMin() { return arr[1]; } public void removeMin() { } public void removeMax() { } public void print() { for (int i = 0; i <= size; i++) { System.out.print( arr[i] + " "); } }} public class BinarH { public static void main(String[] args) { BinarHeap Heap1 = new BinarHeap();…arrow_forwardimport java.util.Scanner; public class LabProgram { public static Roster getInput(){ /* Reads course title, creates a roster object with the input title. Note that */ /* the course title might have spaces as in "COP 3804" (i.e. use nextLine) */ /* reads input student information one by one, creates a student object */ /* with each input student and adds the student object to the roster object */ /* the input is formatted as in the sample input and is terminated with a "q" */ /* returns the created roster */ /* Type your code here */ } public static void main(String[] args) { Roster course = getInput(); course.display(); course.dislayScores(); }} public class Student { String id; int score; public Student(String id, int score) { /* Student Employee */ /* Type your code here */ } public String getID() { /* returns student's id */…arrow_forwardJava utilises the terms counter controlled loop, flag while loop, instance methods, inner classes, shallow copying, and deep copying.arrow_forward
- Convert uml to java code with main methodarrow_forwardHospital Employee //******************************************************************** // HospitalEmployee.java Authors: Lewis/Loftus // // Solution to Programming Project 9.2 //******************************************************************** public class HospitalEmployee { protected String name; protected int number; //----------------------------------------------------------------- // Sets up this hospital employee with the specified information. //----------------------------------------------------------------- public HospitalEmployee(String empName, int empNumber) { name = empName; number = empNumber; } //----------------------------------------------------------------- // Sets the name for this employee. //----------------------------------------------------------------- public void setName(String empName) { name = empName; } //-----------------------------------------------------------------…arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward
- Java. Code: public class Person{// PLEASE START YOUR CODE HERE// *********************************************************// *********************************************************// PLEASE END YOUR CODE HERE//constructorpublic Person(String firstName, String lastName){this.firstName = firstName;this.lastName = lastName;}public String toString(){return firstName + " " + lastName;}}arrow_forwardOn modern processors, what proportion of the time can a good compiler produce better code than an assembly language programmer? What is the purpose?arrow_forwardimport java.util.Scanner; public class LabProgram { /* Define your method here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); /* Type your code here. */ }}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
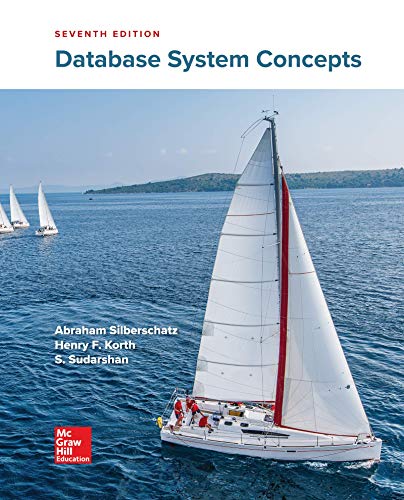
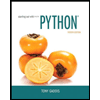
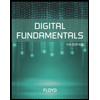
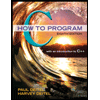
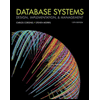
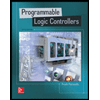