Below is method signature class:
package hW7;
import java.util.Arrays;
public class MergeSort {
public static void mergeSort(int[] arr) {
}
}
and below is from homework 4 merge function:
public static void mergeSort(int [] arr) {
mergeSortRecurse(arr,0,arr.length-1);
}
private static void mergeSortRecurse(int[] arr, int start, int end) {
if(start>=end)
return;
int mid = start + ((end-start)/2);
mergeSortRecurse(arr,start,mid);
mergeSortRecurse(arr,mid+1,end);
merge(arr,start,mid,end);
}
private static void merge(int[] arr, int start, int mid, int end) {
int leftSize = mid - start +1;
int rightSize = end - mid;
int[] left = new int[leftSize+1];
int[] right = new int[rightSize+1];
int leftIndex;
int rightIndex;
for(leftIndex = 0; leftIndex<leftSize;leftIndex++)
left[leftIndex] = arr[start+leftIndex];
for(rightIndex = 0; rightIndex<rightSize;rightIndex++)
right[rightIndex] = arr[mid+rightIndex+1];
left[leftIndex] = Integer.MAX_VALUE;
right[rightIndex] = Integer.MAX_VALUE;
leftIndex = 0;
rightIndex = 0;
for(int mergeIndex = start; mergeIndex<=end;mergeIndex++) {
if(left[leftIndex] <= right[rightIndex]) {
arr[mergeIndex] = left[leftIndex];
leftIndex++;
}
else {
arr[mergeIndex] = right[rightIndex];
rightIndex++;
}
}
}
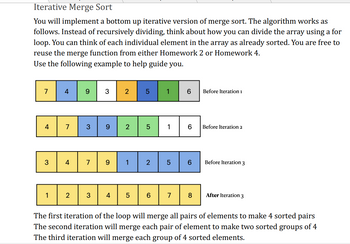

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 2 images

- *JAVA* complete method Delete the largest valueremoveMax(); Delete the smallest valueremoveMin(); class BinarHeap<T> { int root; static int[] arr; static int size; public BinarHeap() { arr = new int[50]; size = 0; } public void insert(int val) { arr[++size] = val; bubbleUP(size); } public void bubbleUP(int i) { int parent = (i) / 2; while (i > 1 && arr[parent] > arr[i]) { int temp = arr[parent]; arr[parent] = arr[i]; arr[i] = temp; i = parent; } } public int retMin() { return arr[1]; } public void removeMin() { } public void removeMax() { } public void print() { for (int i = 0; i <= size; i++) { System.out.print( arr[i] + " "); } }} public class BinarH { public static void main(String[] args) { BinarHeap Heap1 = new BinarHeap();…arrow_forwardimport java.util.Scanner; public class LabProgram { public static Roster getInput(){ /* Reads course title, creates a roster object with the input title. Note that */ /* the course title might have spaces as in "COP 3804" (i.e. use nextLine) */ /* reads input student information one by one, creates a student object */ /* with each input student and adds the student object to the roster object */ /* the input is formatted as in the sample input and is terminated with a "q" */ /* returns the created roster */ /* Type your code here */ } public static void main(String[] args) { Roster course = getInput(); course.display(); course.dislayScores(); }} public class Student { String id; int score; public Student(String id, int score) { /* Student Employee */ /* Type your code here */ } public String getID() { /* returns student's id */…arrow_forwardWrite a java class named First_Last_Recursive_Merge_Sort that implements the recursive algorithm for Merge Sort. You can use the structure below for your implementation. public class First_Last_Recursive_Merge_Sort { //This can be used to test your implementation. public static void main(String[] args) { final String[] items = {"Zeke", "Bob", "Ali", "John", "Jody", "Jamie", "Bill", "Rob", "Zeke", "Clayton"}; display(items, items.length - 1); mergeSort(items, 0, items.length - 1); display(items, items.length - 1); } private static <T extends Comparable<? super T>> void mergeSort(T[] a, int first, int last) { //<Your implementation of the recursive algorithm for Merge Sort should go here> } // end mergeSort private static <T extends Comparable<? super T>> void merge(T[] a, T[] tempArray, int first, int mid, int last) { //<Your implementation of the merge algorithm should go here> } // end merge //Just a quick method to display the whole array. public…arrow_forward
- StringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardpublic class arrayOutput ( public static void main (String [] args) { final int NUM ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; } Type the program's output userVals [0] = 2; userVals [1] = 6; userVals [2] = 8; for (i = userVals.length - 1; i >= 0; −−1) { System.out.println(userVals [1]); } C.C. ? ? ??arrow_forwardJavaTimer.java: import java.util.Arrays;import java.util.Random; public class JavaTimer { // Please expand method main() to meet the requirements.// You have the following sorting methods available:// insertionSort(int[] a);// selectionSort(int[] a);// mergeSort(int[] a);// quickSort(int[] a);// The array will be in sorted order after the routines are called!// Be sure to re-randomize the array after each sort.public static void main(String[] args) {// Create and initialize arraysint[] a = {1, 3, 5}, b, c, d;// Check the time to sort array along startTime = System.nanoTime();quickSort(a);long endTime = System.nanoTime();long duration = (endTime - startTime) / 1000l;// Output resultsSystem.out.println("Working on an array of length " + a.length + ".");System.out.println("Quick sort: " + duration + "us.");}// Thanks to https://www.javatpoint.com/insertion-sort-in-javapublic static void insertionSort(int array[]) {int n = array.length;for (int j = 1; j < n; j++) {int key = array[j];int…arrow_forward
- Exercise 2: Consider the following class: public class Sequence { private ArrayList values; public Sequence() { values = new ArrayList(); } public void add(int n) { values.add(n); } public String toString() { return values.toString(); } } Add a method public Sequence merge(Sequence other) that merges two sequences, alternating ele- ments from both sequences. If one sequence is shorter than the other, then alternate as long as you can and then append the remaining elements from the longer sequence. For example, if a is 1 4 9 16 and b is 9 7 4 9 11 then a.merge(b) returns the sequence 1 9 4 7 9 4 16 9 11 without modifying a or b.arrow_forwardimport java.util.* ; public class Averager { public static void main(String[] args) { //a two dimensional array int[][] a = {{5, 9, 3, 2, 14}, {77, 44, 22, 15, 99}, {14, 2, 3, 9, 5}, {88, 15, 17, 121, 33}} ; System.out.printf("Average = %.2f\n", average(a)) ; System.out.println("EXPECTED:") ; System.out.println("Average = 29.85") ; System.out.printf("Average of evens = %.2f\n", averageEvens(a)) ; System.out.println("EXPECTED:") ; System.out.println("Average of evens = 26.57") ; Random random = new Random(1) ; a = new int[100][1] ; for (int i = 0 ; i < 100 ; i++) a[i][0] = random.nextInt(1000) ; System.out.println("For an array of random values in range 0 to 999:") ; System.out.printf("Average = %.2f\n", average(a)) ; System.out.printf("Average of evens = %.2f\n", averageEvens(a)) ; } /** Find the average of all elements of a two-dimensional array @param aa the two…arrow_forward