// JumpinJava.cpp - This program looks up and prints the names and prices of coffee orders. // Input: Interactive // Output: Name and price of coffee orders or error message if add-in is not found #include #include using namespace std; int main() { // Declare variables. string addIn; // Add-in ordered const int NUM_ITEMS = 5; // Named constant // Initialized array of add-ins string addIns[] = {"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"}; // Initialized array of add-in prices double addInPrices[] = {.89, .25, .59, 1.50, 1.75}; bool foundIt = false; // Flag variable int x; // Loop control variable double orderTotal = 2.00; // All orders start with a 2.00 charge int findItem(); // Get user input cout << "Enter coffee add-in or XXX to quit: "; cin >> addIn; // Write the rest of the program here. findItem(); foundIt = false x = 0 while(x < NUM_ITEMS) if addIn = addIns[x] foundIt = true orderTotal = addInPrices[x] endif x = x + 1 endwhile if(foundIt = true) { cout << "The price of" << addIns[x] << "is" << addInPrices[x] << "." << endl; } else { cout << "Sorry, we do not carry that." << endl; } return 0; } // End of main()
// JumpinJava.cpp - This program looks up and prints the names and prices of coffee orders.
// Input: Interactive
// Output: Name and price of coffee orders or error message if add-in is not found
#include <iostream>
#include <string>
using namespace std;
int main()
{
// Declare variables.
string addIn; // Add-in ordered
const int NUM_ITEMS = 5; // Named constant
// Initialized array of add-ins
string addIns[] = {"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"};
// Initialized array of add-in prices
double addInPrices[] = {.89, .25, .59, 1.50, 1.75};
bool foundIt = false; // Flag variable
int x; // Loop control variable
double orderTotal = 2.00; // All orders start with a 2.00 charge
int findItem();
// Get user input
cout << "Enter coffee add-in or XXX to quit: ";
cin >> addIn;
// Write the rest of the program here.
findItem();
foundIt = false
x = 0
while(x < NUM_ITEMS)
if addIn = addIns[x]
foundIt = true
orderTotal = addInPrices[x]
endif
x = x + 1
endwhile
if(foundIt = true)
{
cout << "The price of" << addIns[x] << "is" << addInPrices[x] << "." << endl;
}
else
{
cout << "Sorry, we do not carry that." << endl;
}
return 0;
} // End of main()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

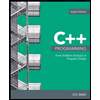
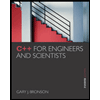
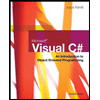
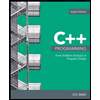
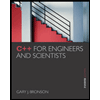
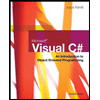