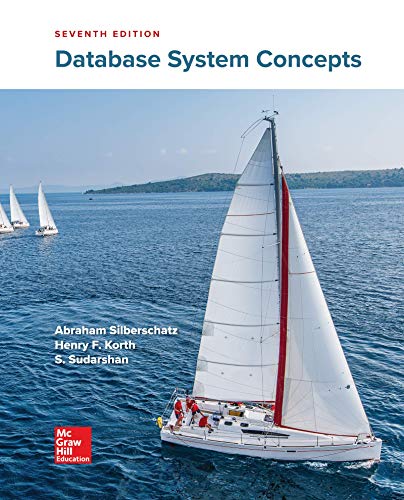
- stdout is not correct
- In some test cases: exception in thread "main" java.util.NoSuchElementException
please fix this code and add a counter. The desired output is given in the picture. Also please add spaces where it belongs
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Scanner;
class Name {
public Name(String last, String first) {
this.last = last;
this.first = first;
}
public Name(String first) {
this("", first);
}
public String getFirst() {
return first;
}
public String getLast() {
return last;
}
public String getFormal() {
return first + " " + last;
}
public String getOfficial() {
return last + " " + first;
}
public String getInitials() {
return first.charAt(0) + "_" + last.charAt(0) + "_";
}
public boolean equals(Name other) {
return first.equals(other.first) && last.equals(other.last);
}
public String toString() {
return first + " " + last;
}
public static Name read(Scanner scanner) {
if (!scanner.hasNext())
return null;
String last = scanner.next();
String first = scanner.next();
return new Name(last, first);
}
private String first, last;
}
class PhoneNumber {
public PhoneNumber(String number) {
this.number = number;
}
public String getAreaCode() {
return number.substring(1, number.indexOf(")"));
}
public String getExchange() {
return number.substring(number.indexOf(")") + 1, number.indexOf("-"));
}
public String getLineNumber() {
return number.substring(number.lastIndexOf("-") + 1);
}
public boolean isTollFree() {
return number.charAt(0) == '8';
}
public boolean equals(PhoneNumber other) {
return number.equals(other.number);
}
public String toString() {
return number;
}
private String number;
public static PhoneNumber read(Scanner scanner) {
if (!scanner.hasNext())
return null;
String number = scanner.next();
return new PhoneNumber(number);
}
}
class PhonebookEntry {
private Name name;
private PhoneNumber phoneNumber;
public PhonebookEntry(Name name, PhoneNumber phoneNumber) {
this.name = name;
this.phoneNumber = phoneNumber;
}
public Name getName() {
return name;
}
public PhoneNumber getPhoneNumber() {
return phoneNumber;
}
public void Display() {
if (phoneNumber.isTollFree()) {
System.out.println("Dialing (toll-free) " + name.getFormal() + ": "
+ phoneNumber.toString());
} else {
System.out.println("Dialing " + name.getFormal() + ": "
+ phoneNumber.toString());
}
}
public String toString() {
return name.toString() + ": " + phoneNumber.toString();
}
public boolean equals(PhonebookEntry other) {
return name.equals(other.name) && phoneNumber.equals(other.phoneNumber);
}
public static PhonebookEntry read(Scanner scanner) {
Name name = Name.read(scanner);
PhoneNumber phoneNumber = PhoneNumber.read(scanner);
if (name == null || phoneNumber == null)
return null;
else
return new PhonebookEntry(name, phoneNumber);
}
}
public class Phonebook {
public static void main(String[] args) {
char choice;
PhonebookEntry entries[]=new PhonebookEntry[100];
Scanner sc = new Scanner(System.in);
int count=0;
Scanner readFile=null;
try {
readFile = new Scanner(new File("phonebook.text"));
PhonebookEntry pe=PhonebookEntry.read(readFile);
while(pe!=null)
{
if(count<entries.length)
{
entries[count]=pe;
count++;
}
else
{
System.out.println("*** Exception *** Phonebook capacity exceded - increase size of underlying array");
PhonebookEntry entriesNew[]=new PhonebookEntry[entries.length*2];
for(int i=0;i<count;i++)
{
entriesNew[i]=entries[i];
}
entries=entriesNew;
entries[count]=pe;
count++;
}
pe=PhonebookEntry.read(readFile);
}
readFile.close();
while(true)
{
System.out.print("lookup, reverse-lookup, quit (l/r/q)? ");
choice=sc.next().charAt(0);
switch(choice)
{
case 'L':
case 'l':{
System.out.print("last name? ");
String lastName=sc.next();
System.out.print("first name? ");
String firstName=sc.next();
int index=searchByName(lastName,firstName,entries,count);
if(index!=-1)
{
System.out.println(entries[index].getName().getFormal()+"'s phone number is "+entries[index].getPhoneNumber().toString());
}
else
{
System.out.println("Name not found");
}
continue;
}
case 'R':
case 'r':{
System.out.print("phone number (nnn-nnn-nnnn)? ");
String number=sc.next();
int index=searchByNumber(number,entries,count);
if(index==-1)
{
System.out.println("** Phone number not found **");
}
else
{
System.out.println(number+" belongs to "+entries[index].getName().getFormal());
}
continue;
}
case 'Q':
case 'q':{
break;
}
default:{
System.out.println("** Invalid Choice **");
continue;
}
}
break;
}
} catch (FileNotFoundException e) {
System.out.println("*** IOException *** phonebook.text not found");
}
}
private static int searchByNumber(String number, PhonebookEntry[] entries,
int count) {
for(int i=0;i<count;i++)
{
if(entries[i].getPhoneNumber().toString().equalsIgnoreCase(number))
{
return i;
}
}
return -1;
}
private static int searchByName(String lastName, String firstName,
PhonebookEntry[] entries, int count) {
for(int i=0;i<count;i++)
{
if(entries[i].getName().getLast().equalsIgnoreCase(lastName) && entries[i].getName().getFirst().equalsIgnoreCase(firstName))
{
return i;
}
}
return -1;
}
}
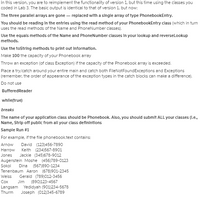
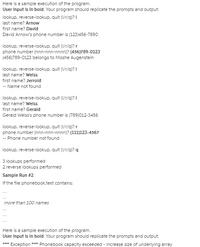

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Change the java code below to handle interrupted exceptions, and also to input the integer to multiply by sleeptime. public class SleepUtilities { /** * Nap between zero and NAP_TIME seconds. */ public static void nap() { nap(NAP_TIME); } /** * Nap between zero and duration seconds. */ public static void nap(int duration) { int sleeptime = (int) (NAP_TIME * Math.random() ); try { Thread.sleep(sleeptime*1000); } catch (InterruptedException e) {} } private static final int NAP_TIME = 5; }arrow_forwardI am writing a method to remove the first element in a doubly linked list. However I am supposed to throw an exception when the list is empty. When I test my class I keep getting "testRemoveFirst caused an ERROR: Uncompilable source code - exception Exceptions.EmptyCollectionException is never thrown in body of corresponding try statement" What does that mean and why is it not able to run?(I'm coding in Java)arrow_forwardWrite a class TimeOfDay that uses the exception classes defined in the previous exercise. Give it a method setTimeTo(timeString) that changes the time if timeString corresponds to a valid time of day. If not, it should throw an exception of the appropriate type. [Java]arrow_forward
- Java Program ASAP Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. Also change the program so that for test cases 2 and 3 the numbers are in there correct places as shown in the input files import java.io.*;import java.util.Scanner;public class FileSorting { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.equalsIgnoreCase("QUIT")) { break; // Exit the program } else { String filePath = new File("").getAbsolutePath() + "/" + input; File file = new File(filePath); if (file.exists() && !file.isDirectory()) { try (BufferedReader br = new BufferedReader(new FileReader(file))) { String st;…arrow_forwardI need help with this Java code, I have already found all the errors and I am getting the correct output. However; in regards to the next part, I have a hard time with loops. I need help creating a Do-while loop to validate all user input and handle exceptions. Please no advanced code, as I am new to Java.arrow_forwardJava Program ASAP Modify this program so it passes the test cases in Hypergrade. Also change the public class to FileSorting import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.equalsIgnoreCase("QUIT")) { break; // Exit the program } else { String filePath = new File("").getAbsolutePath() + "/" + input; File file = new File(filePath); if (file.exists() && !file.isDirectory()) { try (BufferedReader br = new BufferedReader(new FileReader(file))) { String st; StringBuilder formattedText = new StringBuilder(); while ((st = br.readLine()) != null)…arrow_forward
- for some reason I keep getting an error Exception in thread "main" java.lang.Error: Unresolved compilation problem: The method append(char) is undefined for the type StringBuilder at StringBuilder.main(StringBuilder.java:19)arrow_forwardJAVA PROGRAM MODIFY THIS PROGRAM SO IT READS THE TEXT FILES IN HYPERGRADE. I HAVE PROVIDED THE INPUTS AND THE FAILED TEST CASE AS A SCREENSHOT. HERE IS THE WORKING CODE TO MODIFY: import java.io.*;import java.util.*;public class NameSearcher { private static List<String> loadFileToList(String filename) throws FileNotFoundException { List<String> namesList = new ArrayList<>(); File file = new File(filename); if (!file.exists()) { throw new FileNotFoundException(filename); } try (Scanner scanner = new Scanner(file)) { while (scanner.hasNextLine()) { String line = scanner.nextLine().trim(); String[] names = line.split("\\s+"); for (String name : names) { namesList.add(name.toLowerCase()); } } } return namesList; } private static Integer searchNameInList(String name, List<String> namesList) {…arrow_forward1. ArrayIndexOutOfBoundsException Given the following code: public class ExceptionTest{ public static void main( String[] args ){ String nums[] = {“one”, “two”, “three”}; for( int i=0; i<=3; i++ ){ System.out.println(nums[i]); } } } Compile and run the TestExceptions program. The output should look like this: one two three Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 3 at ExceptionTest.main(1.java:4) Modify the TestExceptions program to handle the exception. The output of the program after catching the exception should look like this: one two three Exception caught: Array Index 3 is out of boundsarrow_forward
- I am trying to write a spell checker class from scratch in java, and a spell checker test program in main but I got stuck because public int quadraticProbing(String[] hashArray, int initial_index, int collision) beginning from line 256 of SpellCheckHashTable.java is throwing a NullPointerException in 3 places as shown below. Exception in thread "main" java.lang.NullPointerException at SpellCheckHashTable.quadraticProbing(SpellCheckHashTable.java:265) at SpellCheckHashTable.insert(SpellCheckHashTable.java:209) at SpellCheckHashTableDemo.main(SpellCheckHashTableDemo.java:48) I am trying to have the class use OOP principles of encapsulation, private variables, and getter and setter methods. Apart from hashcode() my program would not use predefined hashing methods from java. The program will: Read a set of words W, from a words.txt file and store them in a hash table; Implement a spellCheck function that performs a spell check on a string s, entered by user at the…arrow_forwardStart with the code below and complete the getInt method. The method should prompt the user to enter an integer. Scan the input the user types. If the input is not an int, throw an IOException; otherwise, return the int. In the main program, invoke the getInt method, use try-catch block to catch the IOException. import java.util.*; import java.io.*; public class ReadInteger{ public static void main() { // your code goes here } public static int getInt() throws IOException { // your code goes here } }arrow_forwardReview the following code. What will be printed to the console when we run the program? Explain why. Be sure to include a discussion of exceptions in your explanation. import java.util.LinkedList; public class Main { public static void main(String[] args) { LinkedList heroes = new LinkedList(); heroes.addLast("Jubilee"); heroes.addLast("Jean Grey"); heroes.addLast("Wolverine"); try { System.out.println(heroes.get(0)); } catch (IndexOutOfBoundsException e) { System.out.println("Exception caught!"); } } }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
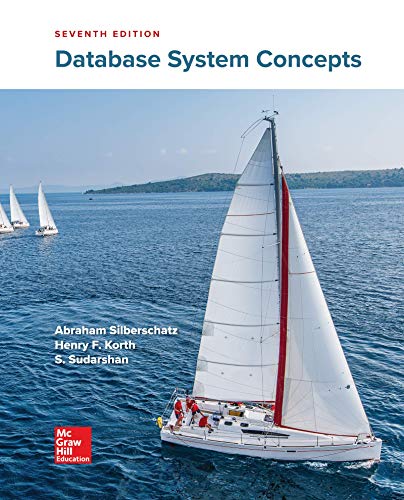
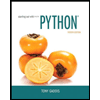
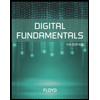
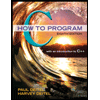
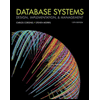
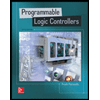