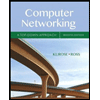
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Using a conditional expression, write a statement that increments numUsers if updateDirection is 1, otherwise decrements numUsers. Ex: if numUsers is 8 and updateDirection is 1, numUsers becomes 9; if updateDirection is 0, numUsers becomes 7
Hint: Start with "numUsers = ...".
#include <iostream>
using namespace std;
int main() {
int numUsers;
int updateDirection;
cin >> numUsers;
cin >> updateDirection;
/* Your solution goes here */
cout << "New value is: " << numUsers << endl;
return 0;
}
Please help me with this problem with C++.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- #include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is the work done in the detailLoop() function // Use switch statement to…arrow_forward// SumAndProduct.cpp - This program computes sums and products // Input: Interactive// Output: Computed sum and product #include <iostream>#include <string>void sums(int);void products(int);using namespace std; int main() { int number; cout << "Enter a positive integer or 0 to quit: "; cin >> number; while(number != 0) { // Call sums function here // Call products function here cout << "Enter a positive integer or 0 to quit: "; cin >> number; } return 0;} // End of main function// Write sums function here// Write products function herearrow_forward#include <iostream> #include <iomanip> #include <string> using namespace std; int main() { constdouble MONSTERA_PRICE =11.50; constdouble PHILODENDRON_PRICE =13.75; constdouble HOYA_PRICE =10.99; constint MAX_POTS =20; constdouble POINTS_PER_DOLLAR =1.0/0.75; char plantType; int quantity; double totalAmount =0.0; int totalPoints =0; int availablePots =0; double plantPrice =0.0; cout << "Welcome to Tom's Plant Shop!" << endl; cout << "******************************" << endl; cout << "Enter your full name: "; string fullName; getline(cin, fullName); output: compiling code... Welcome to Tom's Plant Shop!----------------------------------------Enter your full name: ______ Available plants:M - Monstera ($ 11.5)P - Philodendron ($13.75)H - Hoya ($10.99)Q - Quit shoppingEnter the plant type (M/P/H/Q): ________ Part 1 Tom has recently started a plant-selling business, and he offers three different types of plants, namely Monstera,…arrow_forward
- // EmployeeBonus2.cpp - This program calculates an employee's yearly bonus. #include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is…arrow_forwardmain.cc file #include #include #include #include "cup.h" int main() { std::string drink_name; double amount = 0.0; std::cout << "What kind of drink can I get you?: "; std::getline(std::cin, drink_name); while (std::cout << "How much do you want to fill?: " && !(std::cin >> amount)) { // If the input is invalid, clear it, and ask again std::cin.clear(); std::cin.ignore(); std::cout << "Invalid input; please re-enter.\n"; } //================== YOUR CODE HERE ================== // Instantiate a `Cup` object named `mug`, with // the drink_name and amount given by the user above. //==================================================== while (true) { char menu_input = 'X'; std::cout << "\n=== Your cup currently has " << mug.GetFluidOz() << " oz. of " << mug.GetDrinkType() << " === \n\n"; std::cout << "Please select what you want to do with…arrow_forwardFind errors / syntax error. Write line numberarrow_forward
- // LargeSmall.cpp - This program calculates the largest and smallest of three integer values. #include <iostream> using namespace std; int main() { // This is the work done in the housekeeping() function // Declare and initialize variables here int largest; // Largest of the three values int smallest; // Smallest of the three values // Prompt the user to enter 3 integer values // Write assignment, add conditional statements here as appropriate // This is the work done in the endOfJob() function // Output largest and smallest number. cout << "The largest value is " << largest << endl; cout << "The smallest value is " << smallest << endl; return 0; }arrow_forwardPart 4: Working with Logic Errors: Working with Logic Errors // This program takes two values from the user and then swaps them// before printing the values. The user will be prompted to enter// both numbers.// Place your name here#include <iostream>using namespace std; int main(){float firstNumber;float secondNumber;// Prompt user to enter the first number.cout << "Enter the first number" << endl;cout << "Then hit enter" << endl;cin >> firstNumber;// Prompt user to enter the second number.cout << "Enter the second number" << endl;cout << "Then hit enter" << endl;cin >> secondNumber;// Echo print the input.cout << endl << "You input the numbers as " << firstNumber<< " and " << secondNumber << endl;// Now we will swap the values.firstNumber = secondNumber;secondNumber = firstNumber;// Output the values.cout << "After swapping, the values of the two numbers are "<<…arrow_forward// Airline.cpp - This program determines if an airline passenger is // eligible for a 25% discount. #include <iostream> #include <string> using namespace std; int main() { string passengerFirstName = ""; // Passenger's first name string passengerLastName = ""; // Passenger's last name int passengerAge = 0; // Passenger's age // This is the work done in the housekeeping() function cout << "Enter passenger's first name: "; cin >> passengerFirstName; cout << "Enter passenger's last name: "; cin >> passengerLastName; cout << "Enter passenger's age: "; cin >> passengerAge; // This is the work done in the detailLoop() function // Test to see if this customer is eligible for a 25% discount // This is the work done in the endOfJob() function return 0; }arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
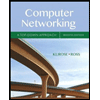
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
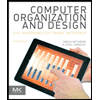
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
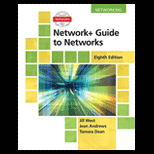
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
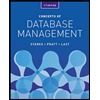
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
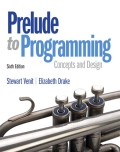
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
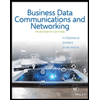
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY