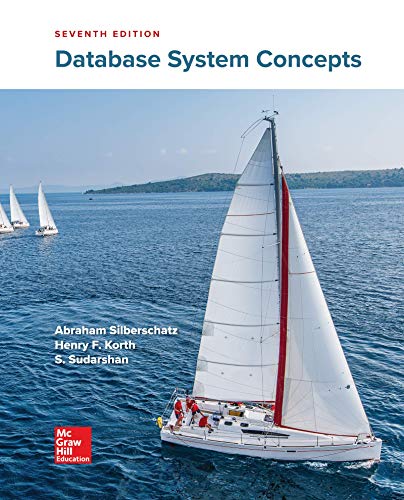
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write an efficient java code (not insert method) to insert x in a binary search tree (BST) with root t.
Please write proper comments in your java code. Your java code should not read or print anything. Assume BST node is defined as follow:
private class node{
int data;
node ll, rl; // left and right link
// node constructor
node(int k) {
data=k; ll=rl=null;
} // end constructor
} // end class node
I have written the first line of code:
node tmp = new node(x); // Allocating space for a new node with x.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java - class IntBTNode { private int data; private IntBTNode left; private IntBTNode right; } Write a new static method of the IntBTNode class to meet the following specification. public static boolean all10(IntBTNode root) // Precondition: root is the root reference of a binary tree (but // NOT NECESSARILY a search tree). // Postcondition: The return value is true if every node in the tree // contains 10. NOTE: If the tree is empty, the method returns true.arrow_forwardVERY EASY RPN PROBLEM. read the directions please answer thank you public class Node <T>{ private T data; private Node<T> next; public Node(T data, Node<T> next) { this.data = data; this.next = next; } public T getData() { return data; } public Node<T> getNext() { return next; } } import java.util.Stack; public class StackArray<T> implements Stack<T> { private T [] elements; private int top; private int size; public StackArray (int size) { elements = (T[]) new Object[size]; top = -1; this.size = size; } public boolean empty() { return top == -1; } public boolean full() { return top == size - 1; } public boolean push(T el) { if (full()) return false; else { top++; elements[top] = el; return true; } }…arrow_forward1. Write code to define LinkedQueue<T> and CircularArrayQueue<T> classes which willimplement QueueADT<T> interface. Note that LinkedQueue<T> and CircularArrayQueue<T>will use linked structure and circular array structure, respectively, to define the followingmethods.(i) public void enqueue(T element); //Add an element at the rear of a queue(ii) public T dequeue(); //Remove and return the front element of the queue(iii) public T first(); //return the front element of the queue without removing it(iv) public boolean isEmpty(); //Return True if the queue is empty(v) public int size(); //Return the number of elements in the queue(vi) public String toString();//Print all the elements of the queuea. Create an object of LinkedQueue<String> class and then add some names of your friendsin the queue and perform some dequeue operations. At the end, check the queue sizeand print the entire queue.b. Create an object from CircularArrayQueue<T> class and…arrow_forward
- 7. Given a Queue Q, write a non-generic method that finds the maximum element in the Queue. You may use only queue operations. No other data structure can be used other than queues. The Queue must remain intact after finding the maximum value. Assume the class that has the findMax method implements Comparable. The header of the findMax method: public Comparable findMax(Queue q)arrow_forwardConsider the following structure of a node for a linked list. struct Node { int key; Node *next; }; What will be the output of the following pseudo-code? Assume, head is a pointer to the first node of the following linked list. 5->2->8->2->9->2->11-> NULL Node *pres head; 0; while(pres!= NULL && Count key : int count 2) { count = count + 1; } pres = pres->next; } cout key « endl; 11 Error 2.arrow_forwardGiven Class:- import java.util.*; // Iterator, Comparatorpublic class BinarySearchTree<T> implements BSTInterface<T>{protected BSTNode<T> root; // reference to the root of this BSTprotected Comparator<T> comp; // used for all comparisonsprotected boolean found; // used by removepublic BinarySearchTree() // Precondition: T implements Comparable// Creates an empty BST object - uses the natural order of elements.{root = null;comp = new Comparator<T>(){public int compare(T element1, T element2){return ((Comparable)element1).compareTo(element2);}};}public BinarySearchTree(Comparator<T> comp) // Creates an empty BST object - uses Comparator comp for order// of elements.{root = null;this.comp = comp;}public boolean isFull()// Returns false; this link-based BST is never full.{return false;}public boolean isEmpty()// Returns true if this BST is empty; otherwise, returns false.{return (root == null);}public T min()// If this BST is empty, returns null;//…arrow_forward
- JAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forwardImplement a __setitem__ function that also supports negative indices. For example: W = L2(Node(10, Node(20, Node(30))))print W[ 10, 20, 30 ] W[1]=25print W[ 10, 25, 30 ] W[-1]=35print W[ 10, 25, 35 ] Complete the code: def L2(*args,**kwargs): class L2_class(L): def __getitem__(self, idx): <... YOUR CODE HERE ...> def __setitem__(self, idx, value): <... YOUR CODE HERE ...> return L2_class(*args,**kwargs) W = L2(Node(10, Node(20, Node(30))))print(W)W[1]=25print(W)W[-1]=35print(W)arrow_forwardPlease fill in the code gaps if possible. This problem has been giving me trouble. Any help is appreciated. public class Node { private String element; // we assume elements are character strings private Node next; /* Creates a node with the given element and next node. */ public Node(String s, Node n) { //fill in body } /* Returns the element of this node. */ public String getElement() { //fill in body } /* Returns the next node of this node. */ public Node getNext() { //fill in body } // Modifier methods: /* Sets the element of this node. */ public void setElement(String newElem) { //fill in body } /* Sets the next node of this node. */ public void setNext(Node newNext) { //fill in body } }arrow_forward
- I want convert the code from singly-linked list to doubly-linked list package Problem; class Node{ private Object value; // Holds the data of the node private Node next; // Holds the follwoing node's address Node(Object v, Node n){ this.value =v; this.next = n; } public Node getNext(){ return this.next; } public Object getValue(){ return this.value; } public void setNext(Node n){ this.next=n; } public void setValue(Object v) { this.value=v; } } class SLL{ public Node head; SLL(){ this.head=null; } public void display(){ Node curr = this.head; while(curr != null) { System.out.print(curr.getValue()); if(curr.getNext()!=null)…arrow_forwardCan you help me with a C++ programming task I am trying to complete for myself please: Write a program (in main.cpp) that: Prompts the user for a filename containing node data. Outputs the minimal spanning tree for a given graph. You will need to implement the createSpanningGraph method in minimalSpanTreeType.h to create the graph and the weight matrix. There are a few tabs: main.cpp, graphType.h, linkedList.h, linkedQueue.h, queueADT.h, minimalSpanTreeType.h, and then two data files labeled: CH20_Ex21Data.txt, CH20Ex4Data.txtarrow_forwardI want convert the code from singly-linked list to doubly-linked list package Problem; class Node{ private Object value; // Holds the data of the node private Node next; // Holds the follwoing node's address Node(Object v, Node n){ this.value =v; this.next = n; } public Node getNext(){ return this.next; } public Object getValue(){ return this.value; } public void setNext(Node n){ this.next=n; } public void setValue(Object v) { this.value=v; } } class SLL{ public Node head; SLL(){ this.head=null; } public void display(){ Node curr = this.head; while(curr != null) { System.out.print(curr.getValue()); if(curr.getNext()!=null)…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
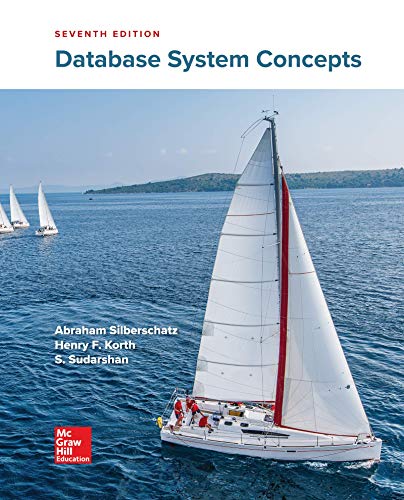
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
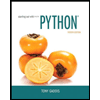
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
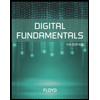
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
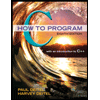
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
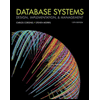
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
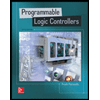
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education