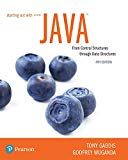
Concept explainers
Roulette Wheel Colors
On a roulette wheel, the pockets are numbered from 0 to 36. The colors of the pockets are as follows:
- Pocket 0 is green.
- For pockets 1 through 10, the odd numbered pockets are red and the even numbered pockets are black.
- For pockets 11 through 18, the odd numbered pockets are black and the even numbered pockets are red.
- For pockets 19 through 28, the odd numbered pockets are red and the even numbered pockets are black.
- For pockets 29 through 36, the odd numbered pockets are black and the even numbered pockets are red.
Write a class named RoulettePocket. The class’s constructor should accept a pocket number. The class should have a method named getPocketColor that returns the pocket’s color, as a string.
Demonstrate the class in a

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Absolute Java (6th Edition)
Problem Solving with C++ (10th Edition)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
C++ How to Program (10th Edition)
C How to Program (8th Edition)
Modern Database Management (12th Edition)
- Card guessing Design a strategy that minimizes the expected number of questions asked in the following game You have a deck of cards that consists of one ace of spades, two deuces of spades, three threes, and on up to nine nines, making 45 cards in all. Someone draws a card from the shuffled deck, which you have to identify by asking questions answerable with yes or no.arrow_forwardTests that look inside the box There are a lot of different ways to test a black box.arrow_forward(Scissor-Rock-Paper game). (A scissor can cut a paper, a rock can knock a scissor, and a paper can wrap a rock.)The program randomly generates a number 0, 1, or 2 representing scissor, rock, and paper. The program prompts the user to enter a number 0, 1, or 2 and displays a message indicating whether the user or the computer wins, loses, or draws.arrow_forward
- START OF PLAY The player starts with 100 points. They have ten attempts (or rounds) to reach 500 points to win. Each round, the player "bets" some amount of points. See gameplay as follows: A HIGH/LOW ROUND (1) The player is shown an initial random card.Cards have a value from 2-14; however, these values are converted to a string as follows: 2-9 are converted to their string equivalent "2", "3", ... "9"10 is "T", 11 is "J", 12 is "Q", 13 is "K" and 14 is "A" (2) After the play sees the first card, they should guess if the next card will be higher (i.e., greater than the current card) or lower (i.e., a lower value than the first card).They will do this by inputting "H" or "L" (lowercase "h" and "l" will also be accepted as valid input). (3) After they input their "high" or "low" guess, the player bets a number of points that their guess will be right. The bet must be between 1 and the total amount of points the player has.1 (4) After the bet amount is entered, a second card will be…arrow_forward9. Trivia Game In this programming exercise, you will create a simple trivia game for two players. The program will work like this: Starting with player 1, each player gets a turn at answering 5 trivia questions. (There should be a total of 10 questions.) When a question is displayed, 4 possible answers are also displayed. Only one of the answers is correct, and if the player selects the correct answer, he or she earns a point. After answers have been selected for all the questions, the program displays the number of points earned by each player and declares the player with the highest number of points the winner. To create this program, write a Question class to hold the data for a trivia question. The Question class should have attributes for the following data: A trivia question Possible answer 1 Possible answer 2 Possible answer 3 Possible answer 4 The number of the correct answer (1, 2, 3, or 4) The Question class also should have an appropriate _…arrow_forward9. Trivia Game In this programming exercise, you will create a simple trivia game for two players. The program will work like this: Starting with player 1, each player gets a turn at answering 5 trivia questions. (There should be a total of 10 questions.) When a question is displayed, 4 possible answers are also displayed. Only one of the answers is correct, and if the player selects the correct answer, he or she earns a point. After answers have been selected for all the questions, the program displays the number of points earned by each player and declares the player with the highest number of points the winner. To create this program, write a Question class to hold the data for a trivia question. The Question class should have attributes for the following data: A trivia question Possible answer 1 Possible answer 2 Possible answer 3 Possible answer 4 The number of the correct answer (1, 2, 3, or 4) The Question class also should have an appropriate _…arrow_forward
- Summary When you borrow money to buy a house, a car, or for some other purposes, then you typically repay it by making periodic payments. Suppose that the loan amount is L, r is the interest rate per year, m is the number of payments in a year, and the loan is for t years. Suppose that i = (r / m) and r is in decimal. Then the periodic payment is (first image which R=....): You can also calculate the unpaid loan balance after making certain payments. For example, the unpaid balance after making k payments is (second image which L'=....) : where R is the periodic payment. (Note that if the payments are monthly, then m = 12.) Instructions Write a program that prompts the user to input the values of L, r, m, t, and k. The program then outputs the appropriate values. Your program must contain at least two functions, with appropriate parameters, to calculate the periodic payments and the unpaid balance after certain payments. Make the program menu driven and use a loop so that the…arrow_forwardAssignment Description This program will simulate part of the game of Yahtzee! This is a dice game that involves rolling five dice and scoring points based on what show up on those five dice. The players would record their scores on a score card, and then total them up, and the player with the larger total wins the game. A Yahtzee score card has two portions: The upper portion has spaces for six scores, obtained by adding up all of the 1's, 2's, 3's, etc. The lower portion has special scores for various combinations: Three of a kind -- at least 3 dice are the same number;the score is the sum of all five dice Four of a kind -- at least 4 dice are the same number;the score is the sum of all five dice Small straight -- four consecutive numbers are represented, e.g. 2345;the score is 25 points Large straight -- five consecutive numbers are represented, e.g. 23456;the score is 30 points Full House -- three of one kind, two of another; the score is 30 points Yahtzee! -- five of a kind; the…arrow_forwardVisual Basic For tax purposes an item may be depreciated over a period of several years, n.With the straight line method of depreciation, each year the item depreciates by 1/nth of itsoriginal value. With the double declining balance method of depreciation, each year the itemdepreciates by 2/nths of its value at the beginning of that year. (In the final year it is depreciatedby its value at the beginning of the year.)Write a program that performs the following tasks:1.1 Request a depreciation of the item, the year of purchase, the cost of the item, the number ofyears to be depreciated (estimated life), and the method of depreciation. The method ofdepreciation should be chosen by clicking on one of two buttons.1.2 Display a year by year description of the depreciation.arrow_forward
- Game Description: Pig is a game that has two players (in our case one human and one computer) that alternate turns. Each player’s goal is to get 100 points rolled on a normal six-sided die first. Each turn consists of the rolling the die repeatedly until you get a 1 or decide to stop. As long as you roll a 2-6, you will add this amount to your total for that turn. But if you roll a 1 during your turn, your turn ends and you receive zero points for that entire turn (erasing all of the progress you made since you last agreed to stop). If you decide to stop rolling at any point in your turn, your points for that turn are then added to the overall score. The overall score is then safe from future rolls. The trick is knowing how long to push it before we should stop and save our gains. See end of this document for an example of a game of pig we should write coding as below In order to explore what is the best strategy for the computer player (i.e. at what score do we stop each computer…arrow_forwardSports exercise advisor algorithm. In this algorithm you will start out with a temperature value in Celsius, so you do not need to ask the user for it. First, convert the temperature to Fahrenheit. Then display a recommended sports exercise based on the Fahrenheit temperature as follows: for temperatures between 65 and 80° display tennis, for colder temperatures display ping pong, and for warmer temperatures display canoeing. thanks in advancearrow_forwardassign(self, assignment:Assignment) -> AssignmentResult: """ This function is to simulate the process of the student receiving an assignment, then working on the assignment, then submitting the assignment and finally receiving grade for the assignment. This function will receive an assignment then a grade should be calculated using the following formula: grade = 1 - (Student's current energy X Assignment difficulty level). The min grade a student may receive is 0% (0) After the grade is calculated the student's energy should be decreased by percentage difficulty. Example if the student has 80% (.8) energy and the assignment is a difficultly level .2 there final energy should be 64% (.64) = .8 - (.8 * .2). The min energy a student may have is 0% (0) Finally the grade calculated should be stored internally with in this class so it can be retrieved later. Then an Assignment Result object should be created with the student's ID, the assignment…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
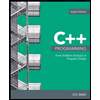
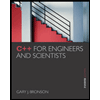