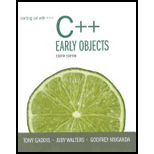
Concept explainers
Bin Manager Class
Design and write an object -oriented
class InvBin
{
private:
string description; //Item name
int qty; // Quantity of items
// in this bin
public:
InvBin (string d = “empty”, int q = 0) // 2-parameter constructor
{ description = d: qty = q: } // with default values
// It will also have the following public member functions. They
// will be used by the BinManager class not the client program.
void setDescription(string d)
string getDescription()
void setQty(1nt q)
int getQty( )
};
class BinManager
{
Private:
InvBin bm[30]: // Array of InvBin objects
int numBins; // Number of bins
// currently 1n use
public:
BinManager() // Default constructor
{ numBins = 0; )
BinManager(int size, string d[], int q[]) //3-parameter constructor
{
//Receives number of bins in use and parallel arrays of item names
//and quantities. Uses this info. to store values in the elements
//of the bin array. Remember, these elements are InvBin objects.
}
// The class will also have the following public member functions:
string getDescription(int index) // Returns name of one item
int getQuantity{int index) // Returns qty of one item
bool addParts{int binlndex, int q) //These return true if the
bool removeParts(int binlndex , int q) //action was done and false
// if it could not be done
//see validation information
Client Program
Once you have created these two classes, write a menu-driven client program that uses a BinManager object to manage its warehouse bins. It should initialize it to use nine of the bins, holding the following item descriptions and quantities. The bin index where the item will be stored is also shown here.
1. regular pliers 25 | 2. n. nose pliers 5 | 3. screwdriver 25 |
4. p. head screw driver 6 | 5. wrench-large 7 | 6. wrench-small 18 |
7. drill 51 | 8. cordless drill 16 | 9. hand saw 12 |
The modular client program should have functions to display a menu, get and validate the user's choice, and carry out the necessary activities to handle that choice. This includes adding items to a bin, removing items from a bin, and displaying a report of all bins. Think about what calls the displayReport client function will need to make to the BinManager object to create this report. When the user chooses the “Quit” option from the menu, the program should call its displayReport function one last time to display the final bin information. All 1/0 should be done in the client class. The BinManager class only accepts information, keeps the array of InvBin objects up to date, and returns information to the client program.
Input Validation: The BinManager class functions should not accept numbers less than 1 for the number of parts being added or removed from a bin. They should also not allow the user to remove more items from a bin than it currently holds.

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Starting Out With C++, Early Objects - With Access Package
Additional Engineering Textbook Solutions
Artificial Intelligence: A Modern Approach
Java: An Introduction to Problem Solving and Programming (7th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
C How to Program (8th Edition)
Problem Solving with C++ (10th Edition)
Digital Fundamentals (11th Edition)
- Double Bubble For this exercise you need to create a Bubble class and construct two instances of the Bubble object. You will then take the two Bubble objects and combine them to create a new, larger combined Bubble object. This will be done using functions that take in these Bubble objects as parameters. The Bubble class contains one data member, radius_, and the corresponding accessor and mutator methods for radius_, GetRadius and SetRadius. Create a member function called CalculateVolume that computes for the volume of a bubble (sphere). Use the value 3.1415 for PI. Your main function has some skeleton code that asks the user for the radius of two bubbles. You will use this to create the two Bubble objects. You will create a CombineBubbles function that receives two references (two Bubble objects) and returns a Bubble object. Combining bubbles simply means creating a new Bubble object whose radius is the sum of the two Bubble objects' radii. Take note that the CombineBubbles function…arrow_forwardProblem: Employee and ProductionWorker Classes Write a python class named ProductionWorker that is a subclass of the Employee class. The ProductionWorker class should keep data attributes for the following information: • Shift number (an integer, such as 1, 2, or 3)• Hourly pay rateThe workday is divided into two shifts: day and night. The shift attribute will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Write the appropriate accessor and mutator methods for this class. Once you have written the class, write a program that creates an object of the ProductionWorker class, and prompts the user to enter data for each of the object’s data attributes. Store the data in the object, then use the object’s accessor methods to retrieve it and display it on the screen Note: The program should be written in python. Sample Input/Output: Enter the name: Ahmed Al-AliEnter the ID number: 12345Enter the department:…arrow_forwardLocalResource(String date, String sector)- Actions of the constructor include accepting a date in the format “dd/mm/yyyy”, initializing the base class, storing the sector and storing an id as a consecutively increasing integer. Note that the constructor must ensure that the date of birth information is recorded. b. getId():Integer - returns the ID of the current instance of LocalResource c. getSector():String – returns the sector associated with the current instance of localResource d. getTRN():String – returns the trn number of the current instance of LocalResource. The process used to determine the TRN is to add the id to the number 100000000 and then returning the string equivalent. e. Update the NineToFiver class to ensure it properly extends the LocalResource class f. In method getContact() of NineToFiver, remove the comments so that the method returns "Local Employee #"+and the id of the contact. Write a concrete class LocalConsultant that extends LocalResource, and implements…arrow_forward
- In C++ Syntax for arrays of objects classGrades allCS[15]; //the default constructor is applied to //all elements of this array //to set the number of students in each class to 25 for (i = 0; i < 15; i++) { allCS[i].setNumStudents(25); } //Assume values in all classes. Write the code to print all class averages?arrow_forwardarray of Payroll ObjectsDesign a PayRoll class that has data members for an employee’shourly pay rate and number of hours worked. Write a program withan array of seven PayRoll objects. The program should read thenumber of hours each employee worked and their hourly pay ratefrom a file and call class functions to store this information in theappropriate objects. It should then call a class function, once foreach object, to return the employee’s gross pay, so this informationcan be displayed.arrow_forward(Polynomial Class) Develop class Polynomial. The internal representation of a Polynomialis an array of terms. Each term contains a coefficient and an exponent, e.g., the term2x4has the coefficient 2 and the exponent 4. Develop a complete class containing proper constructorand destructor functions as well as set and get functions. The class should also provide the followingoverloaded operator capabilities:a) Overload the addition operator (+) to add two Polynomials.b) Overload the subtraction operator (-) to subtract two Polynomials.c) Overload the assignment operator to assign one Polynomial to another.d) Overload the multiplication operator (*) to multiply two Polynomials.e) Overload the addition assignment operator (+=), subtraction assignment operator (-=),and multiplication assignment operator (*=).arrow_forward
- Calculator Class In the file Calculator.java, write a class called Calculator that emulates basic functions of a calculator: add, subtract, multiply, divide, and clear. The class has one private member field called value for the calculator's current value. Implement the following Constructor and instance methods as listed below: public Calculator() - Constructor method to set the member field to 0.0 public void add(double val) - add the parameter to the member field public void subtract(double val) - subtract the parameter from the member field public void multiply(double val) - multiply the member field by the parameter public void divide(double val) - divide the member field by the parameter public void clear( ) - set the member field to 0.0 public double getValue( ) - return the member field Given two double input values num1 and num2, the program outputs the following values: The initial value of the instance field, value The value after adding num1 The value after…arrow_forwardC++ Pet Class You are going to organize and show information about pets in a Pet Store using a Pet class. The Pet class You need to make a Pet class that holds the following information as private data members: the name of the pet (e.g. "Spot", "Fluffy", or any word a user enters) the type of pet (e.g. dog, cat, snake, hamster, or any word a user enters) level of hungriness of the pet (2 means hungry, 1 means content, 0 means full) Your class also needs to have the following functions: a default constructor that sets the pet to be a dog named Buddy, with level of hungriness being “content” a parameterized constructor to allow having any type of pet the order of the parameters is: name of the pet, type of pet, hungriness level a PrintInfo function that prints the info for the pet according to the sample output below a TimePasses function that increases the hungry level of the pet by one (unless its hungry level is already 2, meaning hungry). a FeedPet function that changes the…arrow_forwardC++ Pet Class You are going to organize and show information about pets in a Pet Store using a Pet class. The Pet class You need to make a Pet class that holds the following information as private data members: the name of the pet (e.g. "Spot", "Fluffy", or any word a user enters) the type of pet (e.g. dog, cat, snake, hamster, or any word a user enters) level of hungriness of the pet (2 means hungry, 1 means content, 0 means full) Your class also needs to have the following functions: a default constructor that sets the pet to be a dog named Buddy, with level of hungriness being “content” a parameterized constructor to allow having any type of pet the order of the parameters is: name of the pet, type of pet, hungriness level a PrintInfo function that prints the info for the pet according to the sample output below a TimePasses function that increases the hungry level of the pet by one (unless its hungry level is already 2, meaning hungry). a FeedPet function that changes the…arrow_forward
- (Invoice Class) Create a class called Invoice that a hardware store might use to representan invoice for an item sold at the store. An Invoice should include four data members—a part number (type string), a part description (type string), a quantity of the item being purchased (typeint) and a price per item (type int). Your class should have a constructor that initializes the fourdata members. A constructor that receives multiple arguments is defined with the form:ClassName( TypeName1 parameterName1, TypeName2 parameterName2, ... )Provide a set and a get function for each data member. In addition, provide a member functionnamed getInvoiceAmount that calculates the invoice amount (i.e., multiplies the quantity by theprice per item), then returns the amount as an int value. If the quantity is not positive, it should beset to 0. If the price per item is not positive, it should be set to 0. Write a test program that demonstrates class Invoice’s capabilitiesarrow_forwardNutritional information (classes/constructors) PYTHON ONLY Complete the FoodItem class by adding a constructor to initialize a food item. The constructor should initialize the name (a string) to "None" and all other instance attributes to 0.0 by default. If the constructor is called with a food name, grams of fat, grams of carbohydrates, and grams of protein, the constructor should assign each instance attribute with the appropriate parameter value. The given program accepts as input a food item name, fat, carbs, and protein and the number of servings. The program creates a food item using the constructor parameters' default values and a food item using the input values. The program outputs the nutritional information and calories per serving for both food items. Ex: If the input is: M&M's10.034.02.01.0 where M&M's is the food name, 10.0 is the grams of fat, 34.0 is the grams of carbohydrates, 2.0 is the grams of protein, and 1.0 is the number of servings, the output is:…arrow_forwardProgramming Language C++ Task 1: Write a program to calculate the volume of various containers. A base class, Cylinder, will be created, with its derived classes, also called child classes or sub-classes. First, create a parent class, Cylinder. Create a constant for pi since you will need this for any non-square containers. Use protected for the members. Finally, create a public function that sets the volume. // The formula is: V = pi * (r^2) * h Task 2: Create a derived, or child class for Cylinder, that is, a Cone class. The same function, with the same parameters, is used. However, the formula is different for a cone. // The formula is: V = (1/3) * pi * (r^2) * h Task 3: Test your classes in the main function by creating an instance of Cone and an instance of Cylinder. In each case, call the set_volume function, passing the same parameters. Task 4: Create a derived class for Cone called PartialCone. Add a second radius variable with scope specific to this class (because the top and…arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
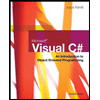