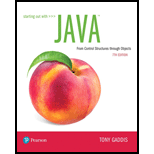
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 9, Problem 12PC
Program Plan Intro
Miscellaneous String Operations
Program Plan:
The detail of “StringOperationsDemo” class is shown below:
- In the “main()” method,
- Declare and initialize the variable and array variable.
- Call the wordCount() method to display the number of words in string.
- Call the mostFrequent() method to display the most frequently occurring character.
- Call replaceSubString() method to replace “the” with “that”.
- Call arrayToString() method to convert an array to a string.
- Display the converted string.
The detail of “StringOperations” class is shown below:
- In the “wordCount()” method,
- Separate the string using a space delimiter.
- Return the number of words in string.
- In the “arrayToString()” method,
- Convert the array value to string.
- Return the converted string.
- In the “mostFrequent()” method,
- Declare the variable.
- Convert the String to an array and store into “cArray” variable.
- Create an integer array variable to store the frequencies of the characters in “cArray” variable.
- The for loop executes until the frequencies length. If yes,
- Initialize frequencies elements to “0”.
- The for loop executes until the array length. If yes, loop executes until the array length. If yes,
- Check frequency of element and then calculate it.
- The for loop executes until the frequencies length. If yes,
- Check the frequencies of elements and determine the most frequent character.
- Return the most frequent character.
- In the “replaceSubString()” method,
- Check the “string2” and “string3” are the same. If yes,
- Return the string.
- Create an object for “string1”.
- Determine the first occurrence of “string2”.
- The while loop executes until the "index" is not equal to “-1”. If yes,
- Call the replace() method to replace this occurrence of the substring.
- Find the next occurrence of “string2”.
- Return the string.
- Check the “string2” and “string3” are the same. If yes,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Complete the class LeetMaker class using String methods. Remember that all the String methods are accessors. They do not change the original String. If you want to apply multiple methods to a String, you will need to save the return value in a variable.
Complete the class by doing the following:
Print the word in lowercase
Replace "e" with "3" (Use the unmodified variable word)
Print the changed word
In the changed word, replace: "t" with "7"
Print the changed word
In this newest changed word, replace: "L" with "1" (uppercase L with the number 1). Then, print the final changed word (with all the replacements)
Print the length of the word
The code to print the original word is already included for you. Do not change that statement. You will need to use the replace() method multiple times. Then print the final String. Remember that replace is an accessor method; It returns the changed version of the String. If you want to do something with the returned String - like use replace() on…
In some circumstances, a class is reliant on itself. That is, one class's object interacts with another class's object. To accomplish this, a method of the class may accept an object of the same class as a parameter.The String class's concat function is an example of this situation. The method is called by one String object and receives another String object as an argument. Here's an illustration:str3 is equal to str1.concat(str2);The method's String object (str1) appends its characters to those of the String supplied as a parameter (str2). As a consequence, a new String object is returned and saved as str3.
Create Java code to implement the conditions given.
Code in Java keep the code as simple as possible do not use arrays nor parseItnt StringBuilder etc keep it very simple.Proper setters, getters, constructors, and toString methods for all of the classes
Class Text
This is a text editing class. In its constructor it receives a string. The string will be stored in a field called initialValue using proper setter methods and proper documentation for Each of the methods .
The class also contains another String field called: ”mixedResult” which is only defined globally and will be initialized using a method in the future in the main class namely the mix method.
The public String toToken() method is a method that will use the initialValue field and it for the letter ’p’ or the letter ’A’. If it finds the letter, every element on the left side of the found letter will be stored in another string and returned. For example: If the initialValue was: ”This is a possible resource allocation”. The returning String will be: ”possible resource…
Chapter 9 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 9.2 - Prob. 9.1CPCh. 9.2 - Write an if statement that displays the word digit...Ch. 9.2 - Prob. 9.3CPCh. 9.2 - Write a loop that asks the user, Do you want to...Ch. 9.2 - Prob. 9.5CPCh. 9.2 - Write a loop that counts the number of uppercase...Ch. 9.3 - Prob. 9.7CPCh. 9.3 - Modify the method you wrote for Checkpoint 9.7 so...Ch. 9.3 - Look at the following declaration: String cafeName...Ch. 9.3 - Prob. 9.10CP
Ch. 9.3 - Prob. 9.11CPCh. 9.3 - Prob. 9.12CPCh. 9.3 - Prob. 9.13CPCh. 9.3 - Look at the following code: String str1 = To be,...Ch. 9.3 - Prob. 9.15CPCh. 9.3 - Assume that a program has the following...Ch. 9.4 - Prob. 9.17CPCh. 9.4 - Prob. 9.18CPCh. 9.4 - Prob. 9.19CPCh. 9.4 - Prob. 9.20CPCh. 9.4 - Prob. 9.21CPCh. 9.4 - Prob. 9.22CPCh. 9.4 - Prob. 9.23CPCh. 9.4 - Prob. 9.24CPCh. 9.5 - Prob. 9.25CPCh. 9.5 - Prob. 9.26CPCh. 9.5 - Look at the following string:...Ch. 9.5 - Prob. 9.28CPCh. 9.6 - Write a statement that converts the following...Ch. 9.6 - Prob. 9.30CPCh. 9.6 - Prob. 9.31CPCh. 9 - The isDigit, isLetter, and isLetterOrDigit methods...Ch. 9 - Prob. 2MCCh. 9 - The startsWith, endsWith, and regionMatches...Ch. 9 - The indexOf and lastIndexOf methods are members of...Ch. 9 - Prob. 5MCCh. 9 - Prob. 6MCCh. 9 - Prob. 7MCCh. 9 - Prob. 8MCCh. 9 - Prob. 9MCCh. 9 - Prob. 10MCCh. 9 - To delete a specific character in a StringBuilder...Ch. 9 - Prob. 12MCCh. 9 - This String method breaks a string into tokens. a....Ch. 9 - These static final variables are members of the...Ch. 9 - Prob. 15TFCh. 9 - Prob. 16TFCh. 9 - True or False: If toLowerCase methods argument is...Ch. 9 - True or False: The startsWith and endsWith methods...Ch. 9 - True or False: There are two versions of the...Ch. 9 - Prob. 20TFCh. 9 - Prob. 21TFCh. 9 - Prob. 22TFCh. 9 - Prob. 23TFCh. 9 - int number = 99; String str; // Convert number to...Ch. 9 - Prob. 2FTECh. 9 - Prob. 3FTECh. 9 - Prob. 4FTECh. 9 - The following if statement determines whether...Ch. 9 - Write a loop that counts the number of space...Ch. 9 - Prob. 3AWCh. 9 - Prob. 4AWCh. 9 - Prob. 5AWCh. 9 - Modify the method you wrote for Algorithm...Ch. 9 - Prob. 7AWCh. 9 - Look at the following string:...Ch. 9 - Assume that d is a double variable. Write an if...Ch. 9 - Write code that displays the contents of the int...Ch. 9 - Prob. 1SACh. 9 - Prob. 2SACh. 9 - Prob. 3SACh. 9 - How can you determine the minimum and maximum...Ch. 9 - Prob. 1PCCh. 9 - Prob. 2PCCh. 9 - Prob. 3PCCh. 9 - Prob. 4PCCh. 9 - Prob. 5PCCh. 9 - Prob. 6PCCh. 9 - Check Writer Write a program that displays a...Ch. 9 - Prob. 8PCCh. 9 - Prob. 9PCCh. 9 - Word Counter Write a program that asks the user...Ch. 9 - Sales Analysis The file SalesData.txt, in this...Ch. 9 - Prob. 12PCCh. 9 - Alphabetic Telephone Number Translator Many...Ch. 9 - Word Separator Write a program that accepts as...Ch. 9 - Pig Latin Write a program that reads a sentence as...Ch. 9 - Prob. 16PCCh. 9 - Lottery Statistics To play the PowerBall lottery,...Ch. 9 - Gas Prices In the student sample program files for...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java Constructor(String) This constructor will take a string of digits(no commas) and turn it into an UnboundedInt object (MUST BE STRING INPUT) UnboundedInt add (UnboundedInt ) A method that adds the current UnboundedInt with a passed in one. The return is a new UnboundedInt. UnboundedInt multiply (UnboundedInt ) - do this one last! A method that multiplies the current UnboundedInt with a passed in one. The return is a new UnboundedInt. void addEnd ( int ) -optional method (helpful) A method to add a new element at the end of the sequence , used for building up each higher term in a single sequence. (i.e. adding a new IntNode to the linked list) UnboundedInt clone( ) a method that returns a copy of the original structure boolean equals ( Object ) a method that returns true if linked list represents the same numerical number as the input parameter. False otherwise. Overrides method in Object class. String toString ( ) creates a string of all elements…arrow_forwardCode in Java keep the code as simple as possible do not use arrays nor parseItnt StringBuilder etc keep it very simple. Class TextEdit In text editing class. Its constructor it receives a string. The string will be stored in a field called initialValue using proper setter methods and proper documentation for Each of the methods. This class also contains another String field called: ”mixedResult” which is only defined globally and will be initialized using a method in the future in the main class namely the mix method. The public String toToken() method is a method that will use the initialValue field and it for the letter ’p’ or the letter ’A’. If it finds the letter, every element on the left side of the found letter will be stored in another string and returned. Example: If the initialValue was: ”This is a possible resource allocation”. The returning String will be: ”possible resource allocation”. Use control statements here iterate through the string using a counter control…arrow_forwardIn GO language. Create a struct that has student name, id, and GPA. Write functions to create a student, modify the student’s id, and modify the student's GPA, and print the student’s information. (This is like creating a class and methods). Now create an array of three students and test your functions. You may hardcode your values if using a web conpiler. (Please hardcode the values!)arrow_forward
- mport java.util.Scanner; public class ParkingCharges { // function to calculate the basic charge using the asked hours static double getBasicCharge(int hours) { if (hours >= 7 && hours <= 8) return 5.50; else if (hours >= 5 && hours <= 6) return 4.50; else if (hours >= 2 && hours <= 4) return 4.00; return 3.00; } // function to return the amount to subtract based on local living and OAP static double getDiscount(String isLocal, String isOAP) { if (isOAP.equals("Yes") && isLocal.equals("Yes")) return 2.0 + 1.0; else if (isOAP.equals("Yes")) return 2.0; else if (isLocal.equals("Yes")) return 1.0; return 0; } public static void main(String[] args) { // create a new Scanner object Scanner sc = new Scanner(System.in); // prompt the user to ask if they are disabled…arrow_forwardJava Program Fleet class- reading from a file called deltafleet.txt-Using file and Scanner Will be creating a file called newfleet.txt Instance Variables:o An array that stores Aircraft that represents Delta Airlines entire fleeto A variable that represents the count for the number of aircraft in the fleet• Methods:o Constructor- One constructor that instantiates the array and sets the count to zeroo readFile()- This method accepts a string that represents the name of the file to beread. It will then read the file. Once the data is read, it should create an aircraft andthen pass the vehicle to the addAircraft method. It should not allow any duplicationof records. Be sure to handle all exceptions.o writeFile()- This method accepts a string that represents the name of the file to bewritten to and then writes the contents of the array to a file. This method shouldcall a sort method to sort the array before writing to it.o sortArray()- This method returns a sorted array. The array is…arrow_forwardLANGUAGE: C++ This is good practice with classes. You are going to create a race class, and inside that class it has a list of entrants (so a list of entry objects) for that race. To test the program you will make an array of three races (so an array of race objects). Populate each race with time and list of entry objects. Print out the schedule. Create a race class that has The name of the race as a character string, ie “RACE 1” or “RACE 2”, The time of the race An array list of entries for that race Create a race array of 3 (or 4) of those race objects, For the list of entries for a particular race that you need above, make a horse entry class (the building block of your array list) you should have The horse name The jockey name The jockey weight Now you need to Populate the structure (ie set up the three races on the schedule, add 2-3 horses entries to each race. You can hardcode the entries if you want to, rather than getting them from the user, just to save the…arrow_forward
- JAVAA7-Write a class with a constructor that accepts a String object as its argument. The class should have a method that returns the number of vowels in the string, and another method that returns the number of consonants in the string. Demonstrate the class in a program by invoking the methods that return the number of vowels and consonants. Print the counts returned.arrow_forwardProject 1Learning objective:This project will help you to learn more about string processing and random class, to define data fields with appropriate getter and setter methods, to encapsulate data fields to make classes easy to maintain.Task:Generate email address for new employees.Create a program which will take inputs first name and last name of the employee.It should generate a new email based on the employee’s first name, last name. Generate a random string for their password. The employee should be able to change the password. Your program should have get and set methods to change the password. Have methods to display name, email, and mailbox capacity=1gb. Create a welcome email for the employee. Count the word count of the welcome email.Results: Sample of the outputEnter first name of the employee: Tom.Enter last name of the employee: Frank.Email generated: Tom_Frank@umsl.eduYour password is mjkfv#$Your mailbox capacity is 1 gbPlease change your password. Enter new…arrow_forward// LeftOrRight.cpp - This program calculates the total number of left-handed and right-handed // students in a class. // Input: L for left-handed; R for right handed; X to quit. // Output: Prints the number of left-handed students and the number of right-handed students. #include <iostream> #include <string> using namespace std; int main() { string leftOrRight = ""; // L or R for one student. int rightTotal = 0; // Number of right-handed students. int leftTotal = 0; // Number of left-handed students. // This is the work done in the housekeeping() function cout << "Enter an L if you are left-handed, a R if you are right-handed or X to quit: "; cin >> leftOrRight; // This is the work done in the detailLoop() function // Write your loop here. // This is the work done in the endOfJob() function // Output number of left or right-handed students. cout << "Number of left-handed students:…arrow_forward
- True or FalseWhen you call a string object’s Split method, the method divides the string into two substrings and returns them as an array of strings.arrow_forwardFlight assignment runs but there is no imput values for the user to interact when running the program. Kindly fix this issue.import java.util.*; class Flight { String airlineCode; int flightNumber; char type; // D for domestic, I for international String departureAirportCode; String departureGate; char departureDay; int departureTime; String arrivalAirportCode; String arrivalGate; char arrivalDay; int arrivalTime; char status; // S for scheduled, A for arrived, D for departed public Flight(String airlineCode, int flightNumber, char type, String departureAirportCode, String departureGate, char departureDay, int departureTime, String arrivalAirportCode, String arrivalGate, char arrivalDay, int arrivalTime) { this.airlineCode = airlineCode; this.flightNumber = flightNumber; this.type = type; this.departureAirportCode = departureAirportCode; this.departureGate = departureGate;…arrow_forwardDefine a class called Book. This class should store attributes such as the title, ISBN number, author, edition, publisher, and year of publication. Provide get/set methods in this class to access these attributes. Define a class called Bookshelf, which contains the main method. This class should create a few book objects with distinct names and store them in an ArrayList. This class should then list the names of all books in the ArrayList. Enhance the program by providing a sort function, which will sort the books in ascending order of their year of publication. Create a few more Bookobjects with the same names but with different edition numbers, ISBNs, and years of publication. Add these new Book objects to the ArrayList, and display the book list sorted by book name; for duplicate books of the same name, sort the list by year of publication. (Hint: You will need to define a comparator class that takes two Book objects as parameters of the compareTo This method should do a two-step…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
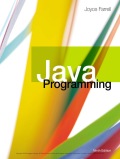
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
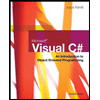
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,