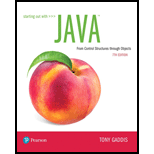
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 9, Problem 5PC
Program Plan Intro
Password Verifier
Program Plan:
The detail of “PasswordDemo” class is shown below:
- In the “main()” method,
- Declare the required variable.
- Read the password input from user.
- Check whether the password is valid or not. If yes,
- Display the error message to user.
- Otherwise, display the valid password message to user.
- Exit the system.
The detail of “PasswordVerifier” class is shown below:
- Declare and initialize the minimum password length.
- In the isValid() method,
- Declare the variable.
- Check whether the password is valid or not. If yes,
- Set the status as “true”.
- Otherwise, set the status as “false”.
- Return the status.
- In the hasUpperCase() method,
- Declare the variable.
- The for loop executes until length of string. If yes,
- Check the string has at least one uppercase character. If yes, set the status as “true”.
- Return the status.
- In the hasLowerCase() method,
- Declare the variable.
- The for loop executes until length of string. If yes,
- Check the string has at least one lowercase character. If yes, set the status as “true”.
- Return the status.
- In the hasDigit() method,
- Declare the variable.
- The for loop executes until length of string. If yes,
- Check the string contains numeric digit. If yes, set the status as “true”.
- Return the status.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Readme.md:
Stars(C++)
This lab exercise will practice creating objects with constructors and destructors and demonstrate when constructors and destructors are called.
Star Class
Create a class, Star. A Star object has two member variables: its name, and a solar radius. This class should have a constructor which takes a std::string, the name of the star, and a double, the solar radius of the star.
In the constructor, the Star class should print to the terminal that the star was born. For example, if you create a Star as follows:
Star my_star("Saiph", 22.2);
Then the constructor should print:
The star Saiph was born.
In the destructor, the Star class should print to the terminal that the star was destroyed, along with the number of times the volume of the sun that that star was, formatted to two decimal places. Hint: use the following line to set the precision to 2 decimal places:
std::cout << std::fixed << std::setprecision(2);
For example, when my_star above has its…
C++
Stars
This lab exercise will practice creating objects with constructors and destructors and demonstrate when constructors and destructors are called.
Star Class
Create a class, Star. A Star object has two member variables: its name, and a solar radius. This class should have a constructor which takes a std::string, the name of the star, and a double, the solar radius of the star.
In the constructor, the Star class should print to the terminal that the star was born. For example, if you create a Star as follows:
Star my_star("Saiph", 22.2);
Then the constructor should print:
The star Saiph was born.
In the destructor, the Star class should print to the terminal that the star was destroyed, along with the number of times the volume of the sun that that star was, formatted to two decimal places. Hint: use the following line to set the precision to 2 decimal places:
std::cout << std::fixed << std::setprecision(2);
For example, when my_star above has its destructor called,…
Grade 12 computer science about inherritence
Please write in Java language
Here is the question
Create an Essay class that inherits the Document class. The Essay class should include member constants MIN_WORDS and MAX_WORDS. An essay should be between 1500 and 3000 words. The Essay class should also contain a member method validLength(), which returns true when the Essay object contains between MIN_WORDS and MAX_WORDS and false otherwise.
Thank you very much
Chapter 9 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 9.2 - Prob. 9.1CPCh. 9.2 - Write an if statement that displays the word digit...Ch. 9.2 - Prob. 9.3CPCh. 9.2 - Write a loop that asks the user, Do you want to...Ch. 9.2 - Prob. 9.5CPCh. 9.2 - Write a loop that counts the number of uppercase...Ch. 9.3 - Prob. 9.7CPCh. 9.3 - Modify the method you wrote for Checkpoint 9.7 so...Ch. 9.3 - Look at the following declaration: String cafeName...Ch. 9.3 - Prob. 9.10CP
Ch. 9.3 - Prob. 9.11CPCh. 9.3 - Prob. 9.12CPCh. 9.3 - Prob. 9.13CPCh. 9.3 - Look at the following code: String str1 = To be,...Ch. 9.3 - Prob. 9.15CPCh. 9.3 - Assume that a program has the following...Ch. 9.4 - Prob. 9.17CPCh. 9.4 - Prob. 9.18CPCh. 9.4 - Prob. 9.19CPCh. 9.4 - Prob. 9.20CPCh. 9.4 - Prob. 9.21CPCh. 9.4 - Prob. 9.22CPCh. 9.4 - Prob. 9.23CPCh. 9.4 - Prob. 9.24CPCh. 9.5 - Prob. 9.25CPCh. 9.5 - Prob. 9.26CPCh. 9.5 - Look at the following string:...Ch. 9.5 - Prob. 9.28CPCh. 9.6 - Write a statement that converts the following...Ch. 9.6 - Prob. 9.30CPCh. 9.6 - Prob. 9.31CPCh. 9 - The isDigit, isLetter, and isLetterOrDigit methods...Ch. 9 - Prob. 2MCCh. 9 - The startsWith, endsWith, and regionMatches...Ch. 9 - The indexOf and lastIndexOf methods are members of...Ch. 9 - Prob. 5MCCh. 9 - Prob. 6MCCh. 9 - Prob. 7MCCh. 9 - Prob. 8MCCh. 9 - Prob. 9MCCh. 9 - Prob. 10MCCh. 9 - To delete a specific character in a StringBuilder...Ch. 9 - Prob. 12MCCh. 9 - This String method breaks a string into tokens. a....Ch. 9 - These static final variables are members of the...Ch. 9 - Prob. 15TFCh. 9 - Prob. 16TFCh. 9 - True or False: If toLowerCase methods argument is...Ch. 9 - True or False: The startsWith and endsWith methods...Ch. 9 - True or False: There are two versions of the...Ch. 9 - Prob. 20TFCh. 9 - Prob. 21TFCh. 9 - Prob. 22TFCh. 9 - Prob. 23TFCh. 9 - int number = 99; String str; // Convert number to...Ch. 9 - Prob. 2FTECh. 9 - Prob. 3FTECh. 9 - Prob. 4FTECh. 9 - The following if statement determines whether...Ch. 9 - Write a loop that counts the number of space...Ch. 9 - Prob. 3AWCh. 9 - Prob. 4AWCh. 9 - Prob. 5AWCh. 9 - Modify the method you wrote for Algorithm...Ch. 9 - Prob. 7AWCh. 9 - Look at the following string:...Ch. 9 - Assume that d is a double variable. Write an if...Ch. 9 - Write code that displays the contents of the int...Ch. 9 - Prob. 1SACh. 9 - Prob. 2SACh. 9 - Prob. 3SACh. 9 - How can you determine the minimum and maximum...Ch. 9 - Prob. 1PCCh. 9 - Prob. 2PCCh. 9 - Prob. 3PCCh. 9 - Prob. 4PCCh. 9 - Prob. 5PCCh. 9 - Prob. 6PCCh. 9 - Check Writer Write a program that displays a...Ch. 9 - Prob. 8PCCh. 9 - Prob. 9PCCh. 9 - Word Counter Write a program that asks the user...Ch. 9 - Sales Analysis The file SalesData.txt, in this...Ch. 9 - Prob. 12PCCh. 9 - Alphabetic Telephone Number Translator Many...Ch. 9 - Word Separator Write a program that accepts as...Ch. 9 - Pig Latin Write a program that reads a sentence as...Ch. 9 - Prob. 16PCCh. 9 - Lottery Statistics To play the PowerBall lottery,...Ch. 9 - Gas Prices In the student sample program files for...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C++ Create a class Rectangle. The class has attributes length and width, each of which defaults to 1. It has member functions that calculate the perimeter and the area of the rectangle. It has setter and getter functions for both length and width. The program should ASK THE USER TO INPUT length and width value. The set functions should verify that length and width are each floating-point numbers larger than 0.0 and less than 20.0. A sample output of your program should look like follows: length = 1.0; width = 1.0; perimeter = 4.0; area = 1.0 another example: length = 5.0; width = 4.0; perimeter = 18.0; area = 20.0 There should be three files and ONE class only. .h file contains class definition, .cpp file contains the implementation of the class in .h file and main.cpp should contain the driver code.arrow_forwardCourse Title: Modern Programming Language Please Java Language Code Question : Create a class called with your 18Arid2891, as Invoice18Arid2891, that a hardware store might use to represent an invoice for an item sold at the store. An Invoice should include four pieces of information as instance variables a part number (type String), a part description (type String),a quantity of the item being purchased (type int) and a price per item (double). Your class should have a constructor that initializes the four instance variables. Provide a set and a get method for each instance variable. In addition, provide a method named getInvoice Amount that calculates the invoice amount (i.e., multiplies the quantity by the price per item), then returns the amount as a double value. If the quantity is not positive, it should be set to 0. If the price per item is not positive, it should be set to 0.0. Write a test application named InvoiceTestMJibranAkram that demonstrates class Invoice’s…arrow_forwardJAVA CODE PLEASE 3. Term by CodeChum Admin Construct a class called Term. It is going to represent a term in polynomial expression. It has an integer coefficient and an exponent. In this case, there is only 1 independent variable that is 'x'. There should be two operations for the Term: public Term times(Term t) - multiplies the term with another term and returns the result public String toString() - prints the coefficient followed by "x^" and appended by the exponent. But with the following additional rules: if the coefficient is 1, then it is not printed. if the exponent is 1, then it is not printed ( the caret is not printed as well) if the exponent is 0, then only the coefficient is printed. Input The first line contains the coefficient and the exponent of the first term. The second line contains the coefficient and the exponent of the second term. 1·1 4·3 Output Display the resulting product for each of the test case. 4x^4arrow_forward
- Bank Accounts (Use Python) Write a program that accepts bank transactions and prints out the balance of an account afterwards. PROGRAM DESIGN Create a class named bankAccount. A bank account should have the following attributes: accNumber, balance, and dateOpened. This class should also have the option to do the following transactions: deposit, withdraw and drop. Initially there is only one bankAccount active in the program, with a balance amount of 1515, and was opened back in 10/01/1987. Depositing adds to a current bankAccount’s balance, while withdrawing – does otherwise. Dropping would equate the current balance to zero. Refer to the following class diagram for more details about the current bankAccount: bankAccountaccNumber = 1balance = 1515dateOpened = "10/27/1987" deposit(dep)withdraw(wd)drop(accNumber) INPUTThe input would be a string that contains the following data: the transaction to be done (dep – for deposit, wd – for withdraw and drop – for drop), the accNumber, and…arrow_forward(Date Class) Create a class called Date that includes three pieces of information as datamembers—a month (type int), a day (type int) and a year (type int). Your class should have a constructor with three parameters that uses the parameters to initialize the three data members. For thepurpose of this exercise, assume that the values provided for the year and day are correct, but ensurethat the month value is in the range 1–12; if it isn’t, set the month to 1. Provide a set and a get function for each data member. Provide a member function displayDate that displays the month, dayand year separated by forward slashes (/). Write a test program that demonstrates class Date’s capabilities.arrow_forwardC# (Odd or Even) Write an app that reads an integer, then determines and displays whether it’s odd or even. (Hints: use the remainder operator) (Multiples) Write an app that reads two integers, determines whether the first is a multiple of the second, and displays the result. (Hints: use the remainder operator). Create a class called Date that includes three pieces of information as auto-implemented properties: a month (type int), a day (type int), and a year (type int). Your class should have a constructor that initializes the three fields. Provide a method DisplayDate() that displays the three fields (separated by '/'). Write a test app named DateDemo that demonstrates class Date’s capabilities.arrow_forward
- (( C# object oriented programming )) Define a rectangle class with length,witdh, coor (x), coor (y). Then write the following methods: (Create a border named rectangle with variables length ,width, color (x), color (y) and do the following operations.)a) Itstores four global variables for length, witdh,coorx and coory. (create four integer variables.)b) Twoconstructors: default and user defined (overloaded).(Create a class called rectangle with variables and do the following.))c) move()method that moves rectangle in x and y directions.(Create a method named move() that takes parameters and move the rectangle in the x and y directions.Annotation: motion operation takes the parX and parY parameters given to the method, increasing or decreasing the coorx and coory variableswill be)d) writetwo get methods which return perimeter and area. (calculate the area and perimeter of the rectangleWrite two methods that return)e) writemain method to test these two constructors by…arrow_forwardIn previous chapters, you have created programs for the Greenville Idol competition. Now create a Contestant class with the following characteristics: The Contestant class contains public static arrays that hold talent codes and descriptions. Recall that the talent categories are Singing Dancing, Musical instrument, and Other. The class contains an auto-implemented property that holds a contestants name. The class contains fields for a talent code and description. The set accessor for the code assigns a code only if it is valid. Otherwise, it assigns I for Invalid. The talent description is a read-only property that is assigned a value when the code is set. Modify the GreenvilleRevenue program so that it uses the Contestant class and performs the following tasks: The program prompts the user for the number of contestants in this years competition; the number must be between 0 and 30. The program continues to prompt the user until a valid value is entered. The expected revenue is calculated and displayed. The revenue is $25 per contestant. The program prompts the user for names and talent codes for each contestant entered. Along with the prompt for a talent code, display a list of the valid categories. After data entry is complete, the program displays the valid talent categories and then continuously prompts the user for talent codes and displays the names of all contestants in the category. Appropriate messages are displayed if the entered code is not a character or a valid code.arrow_forwardUsing classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Your program should be able to handle a maximum of 500 entries. Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables. Define a class extPersonType using the class personType (as defined in Example 10-10, Chapter 10), the class dateType (as designed in this chapters Programming Exercise 2), and the class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information. Use constructors to automatically initialize the member variables. Define the class addressBookType using the previously defined classes. An object of the type addressBookType should be able to process a maximum of 500 entries. The program should perform the following operations: Load the data into the address book from a disk. Sort the address book by last name. Search for a person by last name. Print the address, phone number, and date of birth (if it exists) of a given person. Print the names of the people whose birthdays are in a given month. Print the names of all the people between two last names. Depending on the users request, print the names of all family members, friends, or business associates.arrow_forward
- C PROGRAMMING HELP I need help fixing an old assignment of mine. The point of the script is to read the pasted/attached Number_new.txt file's contents, meant to represent a classes' final grades out of 100 percent, and print an .exe screen as well as another .txt file that repeats the grade number with a letter grade and a PASS or FAIL next to it. At the end it needs to say how many students passed and how many failed. Nothing too complicated with the grading, just 90.0 and up is an A, 80.0 to 89.99 is a B, etc. And of course, anything below 60.0 is an F/FAIL. Please NO IOSTREAM.H OR CACIO.H! I cant use those header files. Below is the script: #include<stdio.h>#include<math.h>#define THEFILE "Number_new.txt" int main(){ float r0[10]; float rr[10]; FILE *fp; char line[81]; fp = fopen(THEFILE, "Number_new.txt"); if (fp != NULL) { /* The file is open. */ printf("%7s %7s %7s %7s %7s %7s %7s %7s %7s %7s\n", "0", "0.01",…arrow_forwardJAVA CODE Class Description: Your need to create an instantiable class that represents a parcel of raw land that can be sold by a real estate company. This class should be named Land. It will contain three fields: a string named location and a width and length, measured in feet, each of which can accept numbers with decimal points. You may assume that every parcel of land is rectangular and that 'width' describes the size of the property along the road and 'length' is how far back it goes from the road. The class needs one constructor that sets the values all the fields. The class needs the following public methods: • Getters and setters for all fields. • A method called getArea that calculates and returns the area of the parcel of land in square feet. (Area is calculated as length x width.) • Standard methods: toString, equals, and hashCode where equals and hashCode define two equivalent parcels of land as each having the same length and each having the same width. • Implementation…arrow_forwardPersonal Information ProgramDevelop a program, in which you’ll design a class that holds the following personal data: name, address, age, and phone number. As part of the program, you’ll then create appropriate accessor and mutator methods. Also, set up three instances of the class. One instance should hold your information, and the other two should hold your friends’ or family members’ information.Input: data for the following attributes of 3 instances of the Personal Information Class: name, address, age, and phone number NOTE: The input data do not need to be real data; they need to be entered at the keyboard, when program is run.Processing: Develop the following: Personal Information Class A program that creates three instances of the above class Output:Set up a loop and display data within each object: name, address, age, and phone numberarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
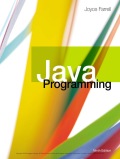
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
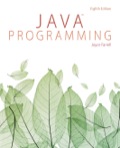
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
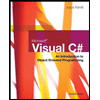
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
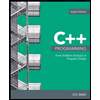
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning