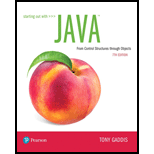
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 9, Problem 8AW
Look at the following string:
“cookies>milk>fudge:cake:ice cream”
Write code using the String class’s split method that can be used to extract the following tokens from the string: cookies, milk, fudge, cake, and ice cream.
Expert Solution & Answer

Learn your wayIncludes step-by-step video

schedule07:09
Students have asked these similar questions
Java Questions - (Has 2 Parts). Based on each code, which answer out of the choices "A, B, C, D, E" is correct. Each question has one correct answer. Thank you.
Part 1 - 9. In Java, composition is said to use __ relationship.
A. is-aB. part-ofC. has-aD. both is-a and part-ofE. both has-a and part-of
Part 2 - 10. Given the following code, which statement is true?
class Water() { ... }class Juice() extends Water { ... }class Apple() { ... }class ApplePie() { Apple a1 = new Apple(); }class AppleJuice() { Apple a1 = new Apple(); Water w1 = newWater(); }
A. Juice is a superclass and Water is a subclass.B. ApplePie is a superclass of Apple.C. ApplePie is a composite class that contains an instance of Apple class.D. AppleJuice is a superclass that contains an instance of Apple class and an instance of Water.E. Water is a composite class that contains an instance of Juice.
Term
by CodeChum Admin (JAVA CODE)
Construct a class called Term. It is going to represent a term in polynomial expression. It has an integer coefficient and an exponent. In this case, there is only 1 independent variable that is 'x'.
There should be two operations for the Term:
public Term times(Term t) - multiplies the term with another term and returns the result
public String toString() - prints the coefficient followed by "x^" and appended by the exponent. But with the following additional rules:
if the coefficient is 1, then it is not printed.
if the exponent is 1, then it is not printed ( the caret is not printed as well)
if the exponent is 0, then only the coefficient is printed.
Input
The first line contains the coefficient and the exponent of the first term. The second line contains the coefficient and the exponent of the second term.
1·1 4·3
Output
Display the resulting product for each of the test case.
4x^4
Java
Constructor(String)
This constructor will take a string of digits(no commas) and turn it into an UnboundedInt object (MUST BE STRING INPUT)
UnboundedInt add (UnboundedInt )
A method that adds the current UnboundedInt with a passed in one. The return is a new UnboundedInt.
UnboundedInt multiply (UnboundedInt ) - do this one last!
A method that multiplies the current UnboundedInt with a passed in one. The return is a new UnboundedInt.
void addEnd ( int ) -optional method (helpful)
A method to add a new element at the end of the sequence , used for building up each higher term in a single sequence. (i.e. adding a new IntNode to the linked list)
UnboundedInt clone( )
a method that returns a copy of the original structure
boolean equals ( Object )
a method that returns true if linked list represents the same numerical number as the input parameter. False otherwise. Overrides method in Object class.
String toString ( )
creates a string of all elements…
Chapter 9 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 9.2 - Prob. 9.1CPCh. 9.2 - Write an if statement that displays the word digit...Ch. 9.2 - Prob. 9.3CPCh. 9.2 - Write a loop that asks the user, Do you want to...Ch. 9.2 - Prob. 9.5CPCh. 9.2 - Write a loop that counts the number of uppercase...Ch. 9.3 - Prob. 9.7CPCh. 9.3 - Modify the method you wrote for Checkpoint 9.7 so...Ch. 9.3 - Look at the following declaration: String cafeName...Ch. 9.3 - Prob. 9.10CP
Ch. 9.3 - Prob. 9.11CPCh. 9.3 - Prob. 9.12CPCh. 9.3 - Prob. 9.13CPCh. 9.3 - Look at the following code: String str1 = To be,...Ch. 9.3 - Prob. 9.15CPCh. 9.3 - Assume that a program has the following...Ch. 9.4 - Prob. 9.17CPCh. 9.4 - Prob. 9.18CPCh. 9.4 - Prob. 9.19CPCh. 9.4 - Prob. 9.20CPCh. 9.4 - Prob. 9.21CPCh. 9.4 - Prob. 9.22CPCh. 9.4 - Prob. 9.23CPCh. 9.4 - Prob. 9.24CPCh. 9.5 - Prob. 9.25CPCh. 9.5 - Prob. 9.26CPCh. 9.5 - Look at the following string:...Ch. 9.5 - Prob. 9.28CPCh. 9.6 - Write a statement that converts the following...Ch. 9.6 - Prob. 9.30CPCh. 9.6 - Prob. 9.31CPCh. 9 - The isDigit, isLetter, and isLetterOrDigit methods...Ch. 9 - Prob. 2MCCh. 9 - The startsWith, endsWith, and regionMatches...Ch. 9 - The indexOf and lastIndexOf methods are members of...Ch. 9 - Prob. 5MCCh. 9 - Prob. 6MCCh. 9 - Prob. 7MCCh. 9 - Prob. 8MCCh. 9 - Prob. 9MCCh. 9 - Prob. 10MCCh. 9 - To delete a specific character in a StringBuilder...Ch. 9 - Prob. 12MCCh. 9 - This String method breaks a string into tokens. a....Ch. 9 - These static final variables are members of the...Ch. 9 - Prob. 15TFCh. 9 - Prob. 16TFCh. 9 - True or False: If toLowerCase methods argument is...Ch. 9 - True or False: The startsWith and endsWith methods...Ch. 9 - True or False: There are two versions of the...Ch. 9 - Prob. 20TFCh. 9 - Prob. 21TFCh. 9 - Prob. 22TFCh. 9 - Prob. 23TFCh. 9 - int number = 99; String str; // Convert number to...Ch. 9 - Prob. 2FTECh. 9 - Prob. 3FTECh. 9 - Prob. 4FTECh. 9 - The following if statement determines whether...Ch. 9 - Write a loop that counts the number of space...Ch. 9 - Prob. 3AWCh. 9 - Prob. 4AWCh. 9 - Prob. 5AWCh. 9 - Modify the method you wrote for Algorithm...Ch. 9 - Prob. 7AWCh. 9 - Look at the following string:...Ch. 9 - Assume that d is a double variable. Write an if...Ch. 9 - Write code that displays the contents of the int...Ch. 9 - Prob. 1SACh. 9 - Prob. 2SACh. 9 - Prob. 3SACh. 9 - How can you determine the minimum and maximum...Ch. 9 - Prob. 1PCCh. 9 - Prob. 2PCCh. 9 - Prob. 3PCCh. 9 - Prob. 4PCCh. 9 - Prob. 5PCCh. 9 - Prob. 6PCCh. 9 - Check Writer Write a program that displays a...Ch. 9 - Prob. 8PCCh. 9 - Prob. 9PCCh. 9 - Word Counter Write a program that asks the user...Ch. 9 - Sales Analysis The file SalesData.txt, in this...Ch. 9 - Prob. 12PCCh. 9 - Alphabetic Telephone Number Translator Many...Ch. 9 - Word Separator Write a program that accepts as...Ch. 9 - Pig Latin Write a program that reads a sentence as...Ch. 9 - Prob. 16PCCh. 9 - Lottery Statistics To play the PowerBall lottery,...Ch. 9 - Gas Prices In the student sample program files for...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
For each of the following activities, give a PEAS description of the task environment and characterize it in te...
Artificial Intelligence: A Modern Approach
What output will be produced by the following code, when embedded in a complete program? int extra = 2; if (ext...
Problem Solving with C++ (9th Edition)
Write a line of Java code that will give the name ANSWER to the int value 42. In other words, make answer a nam...
Absolute Java (6th Edition)
What is an infinite loop?
Starting out with Visual C# (4th Edition)
This is a number that identifies an item in a list. a. element b. index c. bookmark d. identifier
Starting Out with Python (4th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C++ Stars This lab exercise will practice creating objects with constructors and destructors and demonstrate when constructors and destructors are called. Star Class Create a class, Star. A Star object has two member variables: its name, and a solar radius. This class should have a constructor which takes a std::string, the name of the star, and a double, the solar radius of the star. In the constructor, the Star class should print to the terminal that the star was born. For example, if you create a Star as follows: Star my_star("Saiph", 22.2); Then the constructor should print: The star Saiph was born. In the destructor, the Star class should print to the terminal that the star was destroyed, along with the number of times the volume of the sun that that star was, formatted to two decimal places. Hint: use the following line to set the precision to 2 decimal places: std::cout << std::fixed << std::setprecision(2); For example, when my_star above has its destructor called,…arrow_forwardT/F 3) The parameter (String[ ] variable) is used in a Java key method such that a user may run the software and supply "command-line" parameters. The operator, on the other hand, does not need to have any parameters since the parameter is a String sequence.arrow_forwardReadme.md: Stars(C++) This lab exercise will practice creating objects with constructors and destructors and demonstrate when constructors and destructors are called. Star Class Create a class, Star. A Star object has two member variables: its name, and a solar radius. This class should have a constructor which takes a std::string, the name of the star, and a double, the solar radius of the star. In the constructor, the Star class should print to the terminal that the star was born. For example, if you create a Star as follows: Star my_star("Saiph", 22.2); Then the constructor should print: The star Saiph was born. In the destructor, the Star class should print to the terminal that the star was destroyed, along with the number of times the volume of the sun that that star was, formatted to two decimal places. Hint: use the following line to set the precision to 2 decimal places: std::cout << std::fixed << std::setprecision(2); For example, when my_star above has its…arrow_forward
- Complete the class LeetMaker class using String methods. Remember that all the String methods are accessors. They do not change the original String. If you want to apply multiple methods to a String, you will need to save the return value in a variable. Complete the class by doing the following: Print the word in lowercase Replace "e" with "3" (Use the unmodified variable word) Print the changed word In the changed word, replace: "t" with "7" Print the changed word In this newest changed word, replace: "L" with "1" (uppercase L with the number 1). Then, print the final changed word (with all the replacements) Print the length of the word The code to print the original word is already included for you. Do not change that statement. You will need to use the replace() method multiple times. Then print the final String. Remember that replace is an accessor method; It returns the changed version of the String. If you want to do something with the returned String - like use replace() on…arrow_forwardBulgarian solitaire def bulgarian_solitaire(piles, k): You are given a row of piles of pebbles, all identical, and a positive integer k so that the total number of pebbles in these piles equals k*(k+1)//2, the arithmetic sum formula that equals the sum of positive integers from 1 to k. Almost as if intended as a metaphor for the bleak daily life behind the Iron Curtain, all pebbles are identical and you don't have any choice in your moves. Each move picks up one pebble from each pile (even if doing so makes that pile vanish), and creates a new pile from the resulting handful. For example, starting with[7, 4, 2, 1, 1], the first move turns this into [6, 3, 1, 5]. The next move turns that into [5, 2, 4, 4], which then turns into the five-pile configuration [4, 1, 3, 3, 4], and so on. This function should count how many moves are needed to convert the given initial piles into the goal state where each number from 1 to k appears as the size of exactly one pile, and return that count. These…arrow_forwardmport java.util.Scanner; public class ParkingCharges { // function to calculate the basic charge using the asked hours static double getBasicCharge(int hours) { if (hours >= 7 && hours <= 8) return 5.50; else if (hours >= 5 && hours <= 6) return 4.50; else if (hours >= 2 && hours <= 4) return 4.00; return 3.00; } // function to return the amount to subtract based on local living and OAP static double getDiscount(String isLocal, String isOAP) { if (isOAP.equals("Yes") && isLocal.equals("Yes")) return 2.0 + 1.0; else if (isOAP.equals("Yes")) return 2.0; else if (isLocal.equals("Yes")) return 1.0; return 0; } public static void main(String[] args) { // create a new Scanner object Scanner sc = new Scanner(System.in); // prompt the user to ask if they are disabled…arrow_forward
- True or FalseIn structs, all fields and methods are public i.e. all the data can be accessed and changed and all the methods can be called by those who use it.arrow_forwardTreasure Hunter description You are in front of a cave that has treasures. The cave canrepresented in a grid which has rows numbered from 1to , and of the columns numbered from 1 to . For this problem, define (?, )is the tile that is in the -th row and -column.There is a character in each tile, which indicates the type of that tile.Tiles can be floors, walls, or treasures that are sequentially representedwith the characters '.' (period), '#' (hashmark), and '*' (asterisk). You can passfloor and treasure tiles, but can't get past wall tiles.Initially, you are in a tile (??, ). You want to visit all the treasure squares, andtake the treasure. If you visit the treasure chest, then treasurewill be instantly taken, then the tile turns into a floor.In a move, if you are in a tile (?, ), then you can move tosquares immediately above (? 1, ), right (?, + 1), bottom (? + 1, ), and left (?, 1) of thecurrent plot. The tile you visit must not be off the grid, and must not be awall patch.Determine…arrow_forwardQuestion Language: JAVA Keep Guessing Write a program that plays a number-guessing game to guess a secret number randomly generated within the range 1 and 10. The user will get as many numbers of tries as he/she needs to guess the number. Tell the users if their guess is right or wrong and if their guess is wrong let them try to guess the number again. You must generate the secret number using the Java Random class. When the user guesses a number he/she will have to take an input of his guessed number from the keyboard. The user will have to take as many inputs as needed to guess the correct secret-number. That means the code will keep looping as long as the guess is different from the secret number. Use a while loop to prompt the user to guess again if the guess is wrong. Create a class named NumberGuessingGame which will contain the main method, write all your code in the main method. Sample input and output: Suppose the random number generated is 6. I have chosen a…arrow_forward
- C# (Odd or Even) Write an app that reads an integer, then determines and displays whether it’s odd or even. (Hints: use the remainder operator) (Multiples) Write an app that reads two integers, determines whether the first is a multiple of the second, and displays the result. (Hints: use the remainder operator). Create a class called Date that includes three pieces of information as auto-implemented properties: a month (type int), a day (type int), and a year (type int). Your class should have a constructor that initializes the three fields. Provide a method DisplayDate() that displays the three fields (separated by '/'). Write a test app named DateDemo that demonstrates class Date’s capabilities.arrow_forwardScanner method --------------- reads characters until it encounters a newline character, then returns those characters as a String.arrow_forwardIf the new operator cannot allocate the amount of memory requested, it throws_____________arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
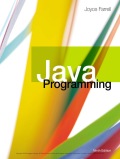
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
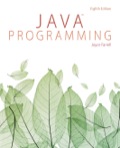
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Program to find HCF & LCM of two numbers in C | #6 Coding Bytes; Author: FACE Prep;https://www.youtube.com/watch?v=mZA3cdalYN4;License: Standard YouTube License, CC-BY