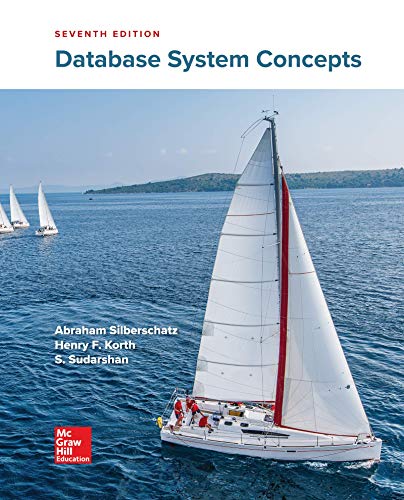
Concept explainers
Expand the example code to have some simple functionality and then write unit tests that integrate with Guice for their behavior.
import com.google.inject.AbstractModule;
public class BillingModule extends AbstractModule {
@Override
protected void configure() {
/*
* This tells Guice that whenever it sees a dependency on a TransactionLog,
* it should satisfy the dependency using a DatabaseTransactionLog.
*/
bind(TransactionLog.class).to(DatabaseTransactionLog.class);
/*
* Similarly, this binding tells Guice that when CreditCardProcessor is used in
* a dependency, that should be satisfied with a PaypalCreditCardProcessor.
*/
bind(CreditCardProcessor.class).to(PaypalCreditCardProcessor.class);
}
}

Step by stepSolved in 2 steps

- Given the code: import java.util.HashMap; import java.util.LinkedList; import java.util.Scanner; public class Controller { privateHashMap<String,LinkedList<Stock>>stockMap; publicController(){ stockMap=newHashMap<>(); Scannerinput=newScanner(System.in); do{ // Prompt for stock name or option to quit System.out.print("Enter stock name or 3 to quit: "); StringstockName=input.next(); if(stockName.equals("3")){ break;// Exit if user inputs '3' } // Get or create a list for the specified stock LinkedList<Stock>stockList=stockMap.computeIfAbsent(stockName,k->newLinkedList<>()); // Prompt to buy or sell System.out.print("Input 1 to buy, 2 to sell: "); intcontrolNum=input.nextInt(); System.out.print("How many stocks: "); intquantity=input.nextInt(); if(controlNum==1){ // Buying stocks System.out.print("At what price: "); doubleprice=input.nextDouble(); buyStock(stockList,stockName,quantity,price); }else{ // Selling stocks System.out.print("Press…arrow_forwardImplement a generic priority queue in Java and test it with an abstract Cryptid class and two concrete Cryptid classes, Yeti and Bigfoot. Create a code package called pq and put all the code for this assignment in it. Write the abstract class Cryptid. A Cryptid has a name (a String) and a public abstract void attack() method. Name should be protected. The class implements Comparable<Cryptid>. The compareTo method returns a negative int if the instance Cryptid's name (that is, the name of the Cryptid you called the method on) is earlier in lexicographic order than the name of the other Cryptid (the one passed in as an argument; it returns a positive int if the other Cryptid's name is earlier in lex order, and returns 0 if they are equal (that is, if they have all the same characters.) This part is easy; just have the method call String's compareTo() method on the instance Cryptid's name, with the other Cryptid's name as the argument, and return the result. Extend Cryptid…arrow_forwardComplete the following class with the appropriate methods shown in the rubric import java.util.HashMap; import java.util.Set; /** * Stores and manages a map of users. * * @author Java Foundations * @version 4.0 */ public class Users { privateHashMap<String, User> userMap; /** * Creates a user map to track users. */ publicUsers() { userMap = newHashMap<String, User>(); } /** * Adds a new user to the user map. * * @param user the user to add */ publicvoidaddUser(Useruser) { userMap.put(user.getUserId(), user); } /** * Retrieves and returns the specified user. * * @param userId the user id of the target user * @return the target user, or null if not found */ publicUsergetUser(StringuserId) { returnuserMap.get(userId); } /** * Returns a set of all user ids. * * @return a set of all user ids in the map */ publicSet<String> getUserIds() { returnuserMap.keySet(); } }arrow_forward
- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardModify the simulate() method in the Simulator class to work with the revised interface of ParkingLot and the new peek() method in Queue. In addition, implement the getIncomingQueueSize() method of Simulator using the size() method of Queue. The getIncomingQueueSize() is going to be used in the CapacityOptimizer class (next task) to determine the size of the incoming queue after a simulation run. CODE TO MODIFY IN JAVA: public class Simulator { /** * Length of car plate numbers */ public static final int PLATE_NUM_LENGTH = 3; /** * Number of seconds in one hour */ public static final int NUM_SECONDS_IN_1H = 3600; /** * Maximum duration a car can be parked in the lot */ public static final int MAX_PARKING_DURATION = 8 * NUM_SECONDS_IN_1H; /** * Total duration of the simulation in (simulated) seconds */ public static final int SIMULATION_DURATION = 24 * NUM_SECONDS_IN_1H; /** * The probability distribution for a car leaving the lot based on the duration * that the car has been parked in…arrow_forwardThe Java classes GenericServlet and HttpServlet may be differentiated from one another with the assistance of an example.arrow_forward
- Explain this code detail by detail like putting comments private void table_update() { int CC; try { // It is for SOMETHING ETC.*** Class.forName("com.mysql.jdbc.Driver"); con1 = DriverManager.getConnection("jdbc:mysql://localhost/linda","root",""); insert = con1.prepareStatement("SELECT * FROM record"); ResultSet Rs = insert.executeQuery(); ResultSetMetaData RSMD = Rs.getMetaData(); CC = RSMD.getColumnCount(); DefaultTableModel DFT = (DefaultTableModel) jTable1.getModel(); DFT.setRowCount(0); while (Rs.next()) { Vector v2 = new Vector(); for (int ii = 1; ii <= CC; ii++) { v2.add(Rs.getString("id")); v2.add(Rs.getString("name")); v2.add(Rs.getString("mobile")); v2.add(Rs.getString("course")); }…arrow_forwardThe map-reduce framework is quite useful for creating inverted indices on a setof documents. An inverted index stores for each word a list of all documentIDs that it appears in (offsets in the documents are also normally stored, butwe shall ignore them in this question).For example, if the input document IDs and contents are as follows:1: data clean2: data base3: clean basethen the inverted lists woulddata: 1, 2clean: 1, 3base: 2, 3Give pseudocode for map and reduce functions to create inverted indices on agiven set of files (each file is a document).Assume the document IDis availableusing a function context.getDocumentID(), and the map function is invokedonce per line of the document. The output inverted list for each word should bea list of document IDs separated by commas. The document IDs are normallysorted, but for the purpose of this question you do not need to bother to sortthem.arrow_forwardDefine the abstract base class LinkedSQD_Base using a linked implementation. Indicate whether each field and method should be public, protected, or private, and explain why. Implement each of the ADTs stack, queue, and deque as a class that extends your base class. Repeat parts a and b, but instead define and use the abstract base class ArraySQD_Base using an array-based implementation. Java programarrow_forward
- Create an implementation of each LinkedList, Queue Stack interface provided For each implementation create a tester to verify the implementation of thatdata structure performs as expected Your task is to: Implement the LinkedList interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in the test harness Create a client ( a class with a main ) ‘StagBusClient’ which builds a bus route by performing the following operations on your linked list: Create (insert) 4 stations List the stations Check if a station is in the list (print result) Check for a station that exists, and one that doesn’t Remove a station List the stations Add a station before another station. List the stations Add a station after another station. Print the stations StagBusClient.java package app; import linkedList.LinkedList; import linkedList.LinkedListImpl; public class StagBusClient { public static void main(String[] args) { // create…arrow_forwardimport java.util.Scanner; public class LabProgram { public static Roster getInput(){ /* Reads course title, creates a roster object with the input title. Note that */ /* the course title might have spaces as in "COP 3804" (i.e. use nextLine) */ /* reads input student information one by one, creates a student object */ /* with each input student and adds the student object to the roster object */ /* the input is formatted as in the sample input and is terminated with a "q" */ /* returns the created roster */ /* Type your code here */ } public static void main(String[] args) { Roster course = getInput(); course.display(); course.dislayScores(); }} public class Student { String id; int score; public Student(String id, int score) { /* Student Employee */ /* Type your code here */ } public String getID() { /* returns student's id */…arrow_forwardDeduce a Java program for the concept of Mutual Exclusion by using the following options. ▪ Synchronized method. ▪ Synchronized block. ▪ Static Synchronizationarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
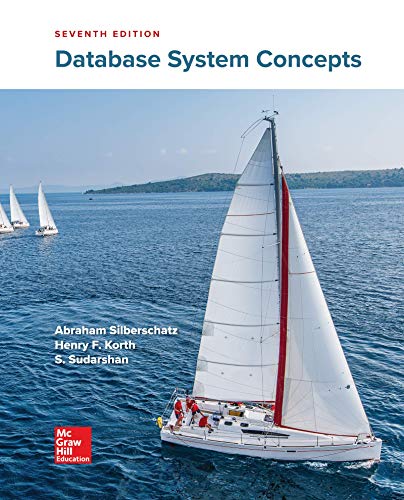
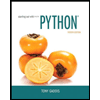
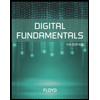
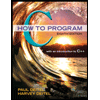
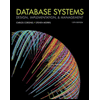
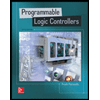