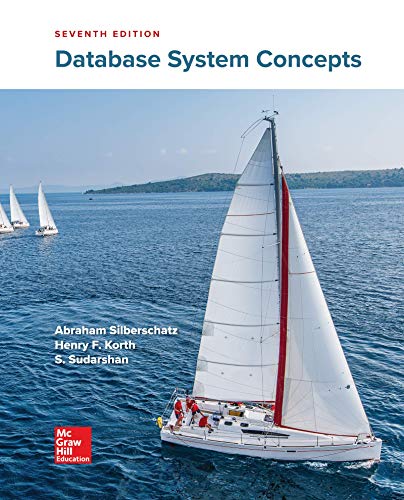
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import org.json.JSONObject;
public class Myclass {
public static void main(String[] args) throws Exception {
try {
URL url = new URL("https://parseapi.back4app.com/classes/City?count=1&limit=10&keys=name,population");
HttpURLConnection urlConnection = (HttpURLConnection)url.openConnection();
urlConnection.setRequestProperty("X-Parse-Application-Id", "mxsebv4KoWIGkRntXwyzg6c6DhKWQuit8Ry9sHja"); // This is the fake app's application id
urlConnection.setRequestProperty("X-Parse-Master-Key", "TpO0j3lG2PmEVMXlKYQACoOXKQrL3lwM0HwR9dbH"); // This is the fake app's readonly master key
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
StringBuilder stringBuilder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
}
JSONObject data = new JSONObject(stringBuilder.toString()); // Here you have the data that you need
System.out.println(data.toString(2));
} finally {
urlConnection.disconnect();
}
} catch (Exception e) {
System.out.println("Error: " + e.toString());
}
}
}
---------------------------------------------
This is the code I have so far for part 2. Am I doing this correctly?
public class city
{
private String name;
private int population;
public City (String cityName, int cityPop)
{
name = cityName;
population = cityPop;
}
}
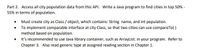

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Complete the following class with the appropriate methods shown in the rubric import java.util.HashMap; import java.util.Set; /** * Stores and manages a map of users. * * @author Java Foundations * @version 4.0 */ public class Users { privateHashMap<String, User> userMap; /** * Creates a user map to track users. */ publicUsers() { userMap = newHashMap<String, User>(); } /** * Adds a new user to the user map. * * @param user the user to add */ publicvoidaddUser(Useruser) { userMap.put(user.getUserId(), user); } /** * Retrieves and returns the specified user. * * @param userId the user id of the target user * @return the target user, or null if not found */ publicUsergetUser(StringuserId) { returnuserMap.get(userId); } /** * Returns a set of all user ids. * * @return a set of all user ids in the map */ publicSet<String> getUserIds() { returnuserMap.keySet(); } }arrow_forwardAssign isTeenager with true if kidAge is 13 to 19 inclusive. Otherwise, assign isTeenager with false. 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 public class TeenagerDetector { publicstaticvoidmain (String [] args) { Scannerscnr=newScanner(System.in); booleanisTeenager; intkidAge; kidAge=scnr.nextInt(); if ( (kidsAge>13) && (kidsAge<19), { isTeenager=true; } elseif { isTeenager=false; } if (isTeenager) { System.out.println("Teen"); } else { System.out.println("Not teen"); }arrow_forwardjavaarrow_forward
- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardin java please.Create a method from the implementation perspective. Create a method where it takes in a linked chain of values and adds them in order to the front. method header:public void addToFront(ANode<T> first)arrow_forwardBuiltInFunctionDefinitionNode.java has an error so make sure to fix it. BuiltInFunctionDefinitionNode.java import java.util.HashMap; import java.util.function.Function; public class BuiltInFunctionDefinitionNode extends FunctionDefinitionNode { private Function<HashMap<String, InterpreterDataType>, String> execute; private boolean isVariadic; public BuiltInFunctionDefinitionNode(Function<HashMap<String, InterpreterDataType>, String> execute, boolean isVariadic) { this.execute = execute; this.isVariadic = isVariadic; } public String execute(HashMap<String, InterpreterDataType> parameters) { return this.execute.apply(parameters); } }arrow_forward
- The main question is the entire page labeled Program #1, the little screenshot is for extra steps. The program has to be written in Java.arrow_forwardDescription: In this project, you are required to make a java code to build a blockchain including multiple blocks. In each block, it contains a list of transactions, previous hash - the digital signature of the previous block, and the hash of the current block which is based on the list of transactions and the previous hash. If anyone would change anything with the previous block, the digital signature of the current block will change. When this changes, the digital signature of the next block will change. Detailed Requirements: 1. Define your block class which contains three private members and four public methods. a. Members: previousHash (int), transactions (String[]), and blockHash (int); b. Methods: i. Block( int previousHash, String[] transactions){} ii. getPreviousHash(){} iii. getTransaction(){} iv. getBlockHash(){} 2. Using ArrayList to design your Blockchain. 3. Your blockchain must contain genesis block and make it to be the first block. 4. You can add a block to the end of…arrow_forwardimport javax.swing.*; import java.util.ArrayList; import java.util.Collections; import java.util.Random; import java.awt.*; import java.awt.event.*; public class memory extends JFrame implements ActionListener { private JButton[] cards; private ImageIcon[] icons; private int[] iconIDs; private JButton firstButton; private ImageIcon firstIcon; private int numMatches; public memory() { setTitle("Memory Matching Game"); setSize(800, 600); setLayout(new BorderLayout()); JPanel boardPanel = new JPanel(new GridLayout(4, 4)); add(boardPanel, BorderLayout.CENTER); icons = new ImageIcon[8]; for (int i = 1; i <= 8; i++) { icons[i-1] = new ImageIcon("image" + i + ".png"); } iconIDs = new int[16]; for (int i = 0; i < 8; i++) { iconIDs[2*i] = i; iconIDs[2*i+1] = i; } Random rand = new Random(); for (int i = 0; i < 16;…arrow_forward
- Here is my code in Java: import java.util.Scanner; import java.util.HashMap; public class Inventory { /** This class demonstrates the inventory */ //Declarations Of inventory static final int LightBulb60W = 1; static final int LightBulb100W = 2; static final int BoltM5 = 3; static final int BoltM8 = 4; static final int Hose25 = 5; static final int Hose50 = 6; public static void main (String [] args) { //Scanner Object @SuppressWarnings("resource") Scanner keyboard = new Scanner(System.in); //Variables int month; int day; int year; double units; double cost = 0; //Declarations int total; HashMap<Integer, String> productMap = new HashMap<>(); productMap.put(1,"Light Bulb 60W"); productMap.put(2,"Light Bulb 100W"); productMap.put(3,"Bolt M5"); productMap.put(4,"Bolt M8"); productMap.put(5,"Hose 25 feet"); productMap.put(6,"Hose 50 feet 60W"); while(true){ //Prints out inventory System.out.println("The Inventory: "); System.out.println("1…arrow_forwardIn netbeans using Java create a class Palindrome2 which replaces the while loop of problem 13 with a for loop.arrow_forwardRun the program to tell me there is an error, BSTChecker.java:3: error: duplicate class: Node class Node { ^ BSTChecker.java:63: error: class LabProgram is public, should be declared in a file named LabProgram.java public class LabProgram { ^ 2 errors This is my program, please fix it. import java.util.*; class Node { int key; Node left, right; public Node(int key) { this.key = key; left = right = null; } } class BSTChecker { public static Node checkBSTValidity(Node root) { return checkBSTValidityHelper(root, null, null); } private static Node checkBSTValidityHelper(Node node, Integer min, Integer max) { if (node == null) { return null; } if ((min != null && node.key <= min) || (max != null && node.key >= max)) { return node; } Node leftResult = checkBSTValidityHelper(node.left, min, node.key); if (leftResult != null) { return leftResult; } Node rightResult = checkBSTValidityHelper(node.right, node.key, max); if (rightResult != null) { return rightResult; }…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
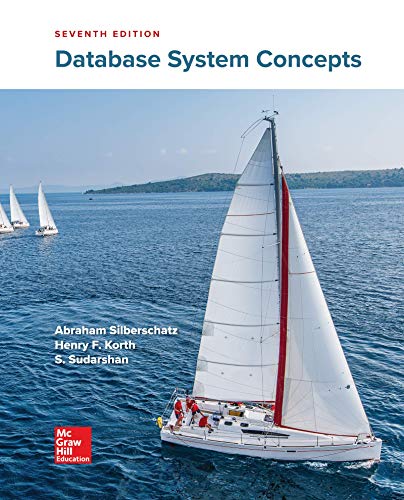
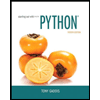
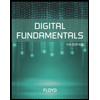
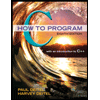
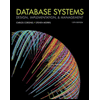
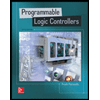