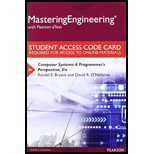
Computer Systems: Program... -Access
3rd Edition
ISBN: 9780134071923
Author: Bryant
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 4, Problem 4.47HW
A.
Program Plan Intro
Given C code:
void bubble_a(long *data, long count)
{
long i, last;
for(last = count – 1; last >0; last--)
{
for(i=0;i<last;i++)
if(data[i+1]<data[i])
{
long t = data[i+1];
data[i+1] = data[i];
data[i] = t;
}
}
}
Data movement instructions:
- The different instructions are been grouped as “instruction classes”.
- The instructions in a class performs same operation but with different sizes of operand.
- The “Mov” class denotes data movement instructions that copy data from a source location to a destination.
- The class has 4 instructions that includes:
- movb:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 1 byte data size.
- movw:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 2 bytes data size.
- movl:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 4 bytes data size.
- movq:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 8 bytes data size.
- movb:
Unary and Binary Operations:
- The details of unary operations includes:
- The single operand functions as both source as well as destination.
- It can either be a memory location or a register.
- The instruction “incq” causes 8 byte element on stack top to be incremented.
- The instruction “decq” causes 8 byte element on stack top to be decremented.
- The details of binary operations includes:
- The first operand denotes the source.
- The second operand works as both source as well as destination.
- The first operand can either be an immediate value, memory location or register.
- The second operand can either be a register or a memory location.
Jump Instruction:
- The “jump” instruction causes execution to switch to an entirely new position in program.
- The “label” indicates jump destinations in assembly code.
- The “je” instruction denotes “jump if equal” or “jump if zero”.
- The comparison operation is performed.
- If result of comparison is either equal or zero, then jump operation takes place.
- The “ja” instruction denotes “jump if above”.
- The comparison operation is performed.
- If result of comparison is greater, then jump operation takes place.
- The “pop” instruction resumes execution of jump instruction.
- The “jmpq” instruction jumps to given address. It denotes a direct jump.
B.
Program Plan Intro
Given C code:
void bubble_p(long* data, long count)
{
long *i, *last;
for (last = data+count-1; last > data; last--)
{
for (i = data; i < last; i++)
{
if (*(i+1) < *i)
{
long t = *(i+1);
*(i+1) = *i;
*i = t;
}
}
}
}
Data movement instructions:
- The different instructions are been grouped as “instruction classes”.
- The instructions in a class performs same operation but with different sizes of operand.
- The “Mov” class denotes data movement instructions that copy data from a source location to a destination.
- The class has 4 instructions that includes:
- movb:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 1 byte data size.
- movw:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 2 bytes data size.
- movl:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 4 bytes data size.
- movq:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 8 bytes data size.
- movb:
Unary and Binary Operations:
- The details of unary operations includes:
- The single operand functions as both source as well as destination.
- It can either be a memory location or a register.
- The instruction “incq” causes 8 byte element on stack top to be incremented.
- The instruction “decq” causes 8 byte element on stack top to be decremented.
- The details of binary operations includes:
- The first operand denotes the source.
- The second operand works as both source as well as destination.
- The first operand can either be an immediate value, memory location or register.
- The second operand can either be a register or a memory location.
Jump Instruction:
- The “jump” instruction causes execution to switch to an entirely new position in program.
- The “label” indicates jump destinations in assembly code.
- The “je” instruction denotes “jump if equal” or “jump if zero”.
- The comparison operation is performed.
- If result of comparison is either equal or zero, then jump operation takes place.
- The “ja” instruction denotes “jump if above”.
- The comparison operation is performed.
- If result of comparison is greater, then jump operation takes place.
- The “pop” instruction resumes execution of jump instruction.
- The “jmpq” instruction jumps to given address. It denotes a direct jump.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a C++ program that makes many references to elements of two-dimensionalarrays using only subscripts. Write a second program that does the same operation butuses pointers and pointer arithmetic to do the array references for the storage-mappingfunction. Compare the time efficiency of the two programs. Which of the two programs islikely to be more reliable? Why?
Consider the following C++ Code
- Write a C++ code that outputs the contents of the array to which strPtr Points and deallocates the memory space occupied by the array to which strPtr points.
- Can you modify the code to use a different array size for strPtr? How would this affect the output of the program?
Someone tries to fix the stack based buffer overflow problem as follows, is it secure now? why? Are there any other issues within the function's implementation below? Explain your reasoning.
int bof(char *str, int size)
{
char *buffer = (char *) malloc(size);
strcpy(buffer, str);
return 1;
}
Chapter 4 Solutions
Computer Systems: Program... -Access
Ch. 4.1 - Prob. 4.1PPCh. 4.1 - Prob. 4.2PPCh. 4.1 - Prob. 4.3PPCh. 4.1 - Prob. 4.4PPCh. 4.1 - Prob. 4.5PPCh. 4.1 - Prob. 4.6PPCh. 4.1 - Prob. 4.7PPCh. 4.1 - Prob. 4.8PPCh. 4.2 - Practice Problem 4.9 (solution page 484) Write an...Ch. 4.2 - Prob. 4.10PP
Ch. 4.2 - Prob. 4.11PPCh. 4.2 - Prob. 4.12PPCh. 4.3 - Prob. 4.13PPCh. 4.3 - Prob. 4.14PPCh. 4.3 - Prob. 4.15PPCh. 4.3 - Prob. 4.16PPCh. 4.3 - Prob. 4.17PPCh. 4.3 - Prob. 4.18PPCh. 4.3 - Prob. 4.19PPCh. 4.3 - Prob. 4.20PPCh. 4.3 - Prob. 4.21PPCh. 4.3 - Prob. 4.22PPCh. 4.3 - Prob. 4.23PPCh. 4.3 - Prob. 4.24PPCh. 4.3 - Prob. 4.25PPCh. 4.3 - Prob. 4.26PPCh. 4.3 - Prob. 4.27PPCh. 4.4 - Prob. 4.28PPCh. 4.4 - Prob. 4.29PPCh. 4.5 - Prob. 4.30PPCh. 4.5 - Prob. 4.31PPCh. 4.5 - Prob. 4.32PPCh. 4.5 - Prob. 4.33PPCh. 4.5 - Prob. 4.34PPCh. 4.5 - Prob. 4.35PPCh. 4.5 - Prob. 4.36PPCh. 4.5 - Prob. 4.37PPCh. 4.5 - Prob. 4.38PPCh. 4.5 - Prob. 4.39PPCh. 4.5 - Prob. 4.40PPCh. 4.5 - Prob. 4.41PPCh. 4.5 - Prob. 4.42PPCh. 4.5 - Prob. 4.43PPCh. 4.5 - Prob. 4.44PPCh. 4 - Prob. 4.45HWCh. 4 - Prob. 4.46HWCh. 4 - Prob. 4.47HWCh. 4 - Prob. 4.48HWCh. 4 - Modify the code you wrote for Problem 4.47 to...Ch. 4 - In Section 3.6.8, we saw that a common way to...Ch. 4 - Prob. 4.51HWCh. 4 - The file seq-full.hcl contains the HCL description...Ch. 4 - Prob. 4.53HWCh. 4 - The file pie=full. hcl contains a copy of the PIPE...Ch. 4 - Prob. 4.55HWCh. 4 - Prob. 4.56HWCh. 4 - Prob. 4.57HWCh. 4 - Our pipelined design is a bit unrealistic in that...Ch. 4 - Prob. 4.59HW
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a C++ program that ask user to enter a choice between 0 and 2 to do different tasks. 0 for add, 1 for subtract and 2 for multiply. Use arrays of function pointer to work on this problem. Like: void (*fun_ptr_arr[])(int, int) = {add, subtract, multiply};arrow_forwardWrite a C++ program that will implement and test the five functions described below that use pointers and dynamic memory allocation. The Functions: You will write the five functions described below. Then you will call them from the main function, to demonstrate their correctness. findLast: takes an array of integers, its size and a target integer value. Returns an index of the last occurrence of the target element in the array. If the target value doesn't exist, returns -1. For this function only: Do not use square brackets anywhere in the function, not even the parameter list (use pointer notation instead). nextFibonacci. Modify the following function to use pointers as parameters (instead of reference parameters). The Fibonacci sequence is a series of numbers where each number is the addition of the previous two numbers, starting with 0, and 1 (specifically: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55…). This function updates the given i-th and (i+1)-st Fibonacci numbers to (i+1)-st and…arrow_forwardI'm having a hard time seeing the problem with this buffer overflow problem can someone please elaborate if its secure or not, and if there are any other issues with the implementation in the problem? Someone tries to fix the stack based buffer overflow problem as follows, is it secure now? why? Are there any other issues within the function's implementation below? Explain your reasoning. int bof(char *str, int size) { char *buffer = (char *) malloc(size); strcpy(buffer, str); return 1; }arrow_forward
- In C++, how simple is it to duplicate an array of shared pointers into a new array? Create a list of potential solutions to the issue at hand. Do the objects controlled by a shared pointer also get copied when it's copied? Explainarrow_forwardUsing only getchar from stdio.h along with the standard operators, create a program in c language where: -Initialize a multidimensional array words, where the size of the first dimension is MAXWORDS and the size of the second dimension is MAXLEN. -Write lines of code which takes an input stream of characters (max of 1000 characteres) from the user using getchar, until the user triggers the end of file.-Create three arrays of pointers p1[], p2[], p3[], where each element of these arrays point to a string.arrow_forwardwrite a c++ program for the following Write a function called mirror_add that takes two 2D arrays as parameters and adds the“mirrored” version of the second one to the first one, for example :first[1,2,3][2,0,1]+second[4,3,1] ----------->[2,1,3]first[2,5,7][5,1,3]You only need to write the mirror_add function, not the main function. Assume that thecolumn size of both arrays is 3.arrow_forward
- Show code in C++ to print the element of arr2 that corresponds to *p (from arr1) -- use no variables beyond what are given here. When given two parallel arrays and a pointer into one of them, use pointer math to access the coinciding element of the second array. Here are the declarations we are dealing with: double arr1[MAX]; char arr2[MAX]; double * p; // points into arr1 at a particular elementarrow_forwardWrite a program to sort the elements of 1D array in descending order and also implement linear search on the same array. Both sorting and searching operations should be done inside one user defined function: “Task”. So, for achieving the required functionality, pass array into the function: Task () from main () using Pass by reference/ or address approach.arrow_forwardWrite a C program that uses the following: a main() to read two integer values from the user, val1 and val2, and prints the returned value from swap().a swap() that uses call by reference (takes the addresses into pointers) to swap values, and prints their values after the swap "num1 = # and num2 = #". This function returns the largest of the two values. If these are equal, it returns their sum.arrow_forward
- Talk about the difference of accessing elements in an array VS access the memory address of an array What will happen with the following code: 1: cout << array<<endl; 2: cout << array[0] << endl; Does C++ support Index Out of bound Checking? If so, please explain how that works, if not, explain what will happen if Index Out of bound happens.arrow_forwardWrite a C function and program that calculates the array size after deleting a certain element of a 10-element integer array from the array. Sample: Series: 1 2 2 3 4 4 5 5 6 6 Array size after 4 deleted: 8 Note: A CALL BY REFERENCE function will be written. The element we want to delete will be entered by the user. The entered number can be repeated more than once in the array, the asking array size will be calculated after all duplicates are deleted.arrow_forwardWrite a program to sort the elements in 1D array in descending order and also implement linear search on the same array. Both sorting and searching operations should be done inside user-defined function: "Task". So,for achieving the required functionality,pass,array into the function: Task () from main () using pass by reference/or address approach.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
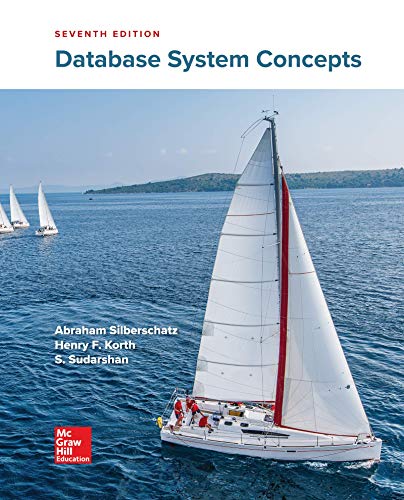
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
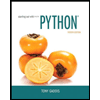
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
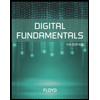
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
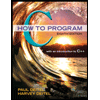
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
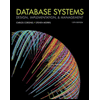
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
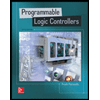
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education