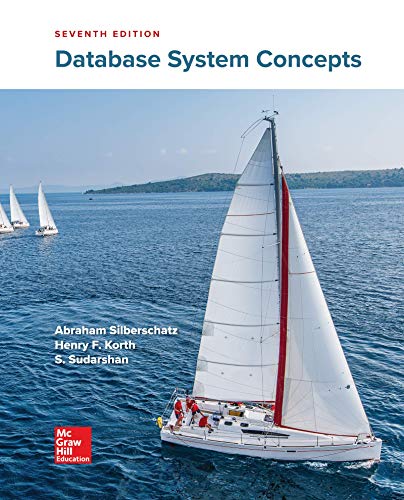
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Redo project 6 using ArrayList.
import java.util.Scanner;
import java.util.*;
public class CardGame {
static Scanner scanner = new Scanner(System.in);
static Random random = new Random();
public static void main(String[] args) {
System.out.println("Welcome to the card game! Here are the instructions:");
System.out.println("- You will randomly draw a card from a deck of 52 cards.");
System.out.println("- The computer will also draw a card from the same deck.");
System.out.println("- If your card has a higher face value, you win. Otherwise, you lose.");
System.out.println("- If your card has the same face value as the computer's card, the suit determines the order.");
int gamesPlayed = 0;
int userWins = 0;
int computerWins = 0;
boolean[] cards = new boolean[52]; // false means the card is available, true means it's been drawn
while (true) {
System.out.println("\nNew game!");
Card userCard = drawCard(cards);
Card computerCard = drawCard(cards);
System.out.println("Your card is " + userCard);
System.out.println("Computer's card is " + computerCard);
int userValue = userCard.getValue();
int computerValue = computerCard.getValue();
if (userValue > computerValue) {
System.out.println("You win!");
userWins++;
} else if (userValue < computerValue) {
System.out.println("You lose!");
computerWins++;
} else {
// same face value, compare suits
int userSuit = userCard.getSuit();
int computerSuit = computerCard.getSuit();
if (userSuit > computerSuit) {
System.out.println("You win!");
userWins++;
} else {
System.out.println("You lose!");
computerWins++;
}
}
gamesPlayed++;
if (gamesPlayed == 26) {
System.out.println("\nYou have played 26 games, which is half of the deck.");
System.out.println("Do you want to shuffle the deck and start a new game? (y/n)");
String answer = scanner.next();
if (answer.equals("y")) {
Arrays.fill(cards, false);
gamesPlayed = 0;
userWins = 0;
computerWins = 0;
} else {
break;
}
} else {
System.out.println("\nDo you want to play another game? (y/n)");
String answer = scanner.next();
if (!answer.equals("y")) {
break;
}
}
}
System.out.println("\nThanks for playing!");
System.out.println("You played " + gamesPlayed + " games.");
System.out.println("You won " + userWins + " times.");
System.out.println("The computer won " + computerWins + " times.");
}
public static Card drawCard(boolean[] cards) {
while (true) {
int suit = random.nextInt(4) + 1;
int value = random.nextInt(13) + 2;
int index = (suit - 1) * 13 + (value - 2);
if (!cards[index]) {
cards[index] = true;
return new Card(suit, value);
}
}
}
public static class Card {
private int suit;
private int value;
public Card(int suit, int value) {
this.suit = suit;
this.value = value;
}
public int getSuit() {
return suit;
}
public int getValue() {
return value;
}
public String toString() {
String suitStr = "";
switch (suit) {
case 1: suitStr = "club"; break;
case 2: suitStr = "diamond"; break;
case 3: suitStr = "heart"; break;
case 4: suitStr = "spade"; break;
}
String valueStr;
switch (value) {
case 11: valueStr = "Jack"; break;
case 12: valueStr = "Queen"; break;
case 13: valueStr = "King"; break;
case 14: valueStr = "Ace"; break;
default: valueStr = Integer.toString(value); break;
}
return valueStr + " of " + suitStr + "s";
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- import java.util.Scanner; public class AverageWithSentinel{ public static final int END_OF_INPUT = -500; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Declare an int variable with an initial value // as the count of input integers // Step 3: Declare a double variable with an initial value // as the total of all input integers // Step 4: Display an input prompt // "Enter an integer, -500 to stop: " // Step 5: Read an integer and store it in an int variable // Step 6: Use a while loop to update count and total as long as // the input value is not -500. // Then display the same prompt and read the next integer // Step 7: If count is zero // Display the following message // "No integers were…arrow_forwardimport java.util.Scanner; public class leapYearLab { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int userYear; boolean LeapYear; System.out.println("Enter the year"); userYear = scnr.nextInt(); isLeapYear(userYear); //if leap year if (isLeapYear){ System.out.println(userYear + " - leap year"); } else{ System.out.println(userYear + " - not a leap year"); } scnr.close(); } public static boolean isLeapYear(int userYear){ boolean LeapYear; /* Type your code here. */ if ( userYear % 4 == 0) { // checking if year is divisible by 100 if ( userYear % 100 == 0) { // checking if year is divisible by 400 // then it is a leap year if ( userYear % 400 == 0) LeapYear = true; else LeapYear = false; } // if the year is not divisible by 100 else…arrow_forwardimport java.util.Scanner; public class ParkingFinder {/* Your code goes here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int numVisits; int duration; numVisits = scnr.nextInt(); duration = scnr.nextInt(); System.out.println(findParkingPrice(numVisits, duration)); }}arrow_forward
- import java.util.Scanner; public class RomanNumerals { public static void main(String[] args) { Scanner in = new Scanner("I C X D M L"); char romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 1") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 100") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 10") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 500") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 1000") ; romanNumeral = in.next().charAt(0); System.out.println("Value: " + valueOf(romanNumeral) + " Expected: 50") ; } /** Gives the value…arrow_forwardimport java.util.Scanner; public class CircleAndSphereWhileLoop{ public static final double MAX_RADIUS = 500.0; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Read a double value as radius using prompt // "Enter the radius (between 0.0 and 500.0, exclusive): " // Step 3: While the input radius is not in the ragne (0.0, 500.0) // Display a message on one line (ssuming input value -1) // "The input number -1.00 is out of range." // Read a double value as radius using the same promt double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 * Math.PI * Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); // Step 4: Display the radius, circle circumference, circle area, // sphere surface area, and…arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward
- import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print(" "); String s1 = sc.nextLine(); System.out.print(""); String s2 = sc.nextLine(); int minLen = Math.min(s1.length(), s2.length()); int matchCount = 0; for (int i = 0; i < minLen; i++) { if (s1.charAt(i) == s2.charAt(i)) { matchCount++; } } if (matchCount == 1) { System.out.println("1 character matches"); } else { System.out.println(matchCount + " characters match"); } sc.close(); }}arrow_forwardimport java.util.Scanner; public class AutoBidder { public static void main (String [] args) { Scanner scnr = new Scanner (System.in); char keepGoing; int nextBid; nextBid = 0; keepGoing 'y'; * Your solution goes here */ while { nextBid = nextBid + 3; System.out.println ("I'll bid $" + nextBid + "!") ; System.out.print ("Continue bidding? (y/n) "); keepGoing = scnr.next ().charAt (0); } System.out.println (""); }arrow_forward1 import java.util.Scanner; 2 3 public class RedBalloon { public static void main (String 0 args) { Scanner scnr = new Scanner(System.in); boolean isRed; boolean isBalloon; 4 5 6 7 8 isRed = scnr.nextBoolean(); isBalloon = scnr.nextBoolean(); 9 10 11 12 13 } 15 } 14arrow_forward
- package lab06;;public class gradereport { public static void main(String[] args) { Scanner in = new Scanner(System.in);double[] Scores = new double[10]; for(int i=0;i<10;i++){ System.out.println("Enter score " + (i+1));scores[i]=in.nextdouble(); } for(int i=0;i<10;i++){ if (scores[i] >=80) System.out.println("Score " + (i+1) + " receives a grade of HD"); else if (scores[i]>=70) System.out.println("Score " + (i+1) + " receives a grade of D"); else if (scores[i] >=60) System.out.println("Score "+ (i+1) + " receives a grade of C"); else if (scores[i] >=50) System.out.println("Score " + (i+1) + " receives a grade of P"); else if (scores[i] >=40) System.out.println("Score " + (i+1) + " receives a grade of MF"); else if (scores[i] >=0) System.out.println("Score " +…arrow_forwardimport java.util.Scanner;public class main{public static void main(String[] args){Scanner sc = new Scanner(System.in):int year, day, weekday;String month;String outday = "";System.out.printf("Enter the month%n");month = sc.nextLine();System.out.printf("Enter the day%n");month = sc.nextInt();weekay = find_day(month, day);outday = out_weekday(weekday);System.out.printf("The day is: %s%n", outday);}} Change the program from the previous example to take command line arguments instead of using the Scanner. For example, running the program like this:> java OutDays March 14will output the weekday string (which is Thursday) on the console for March 14, 2019). public static void main(String[] args){ int year, day, weekday; String month; String outday = ""; // #### your code here for accessing the command line arguments weekday = find_day(month, day); outday = out_weekday(weekday); System.out.printf("The day is: %s%n", outday);}arrow_forwardConvert the following java code to C++ //LabProgram.javaimport java.util.Scanner;public class LabProgram {public static void main(String[] args) {//defining a Scanner to read input from the userScanner input = new Scanner(System.in);//reading the value for Nint N = input.nextInt();//creating a 1xN matrixint[] m1 = new int[N];//creating an NxN matrixint[][] m2 = new int[N][N];//creating a 1xN matrix to store the resultint[] result = new int[N];//looping and reading N integers into m1for (int i = 0; i < N; i++) {m1[i] = input.nextInt();}//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//reading an integer and storing it into m2 at position i,jm2[i][j] = input.nextInt();}}//multiply m1 and m2, store result in result//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//multiplying value at index j in m1 with value at j,i in m2, adding to current value at index i// on…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
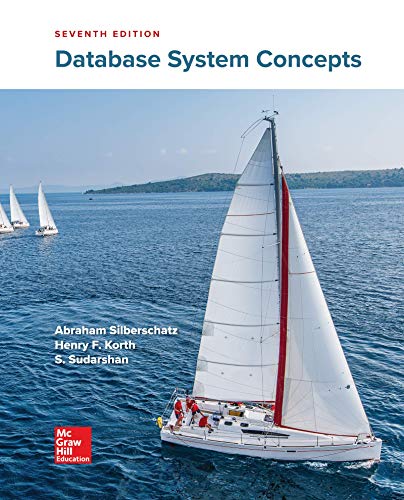
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
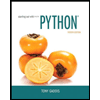
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
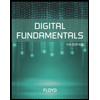
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
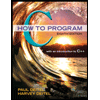
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
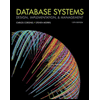
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
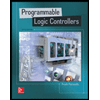
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education