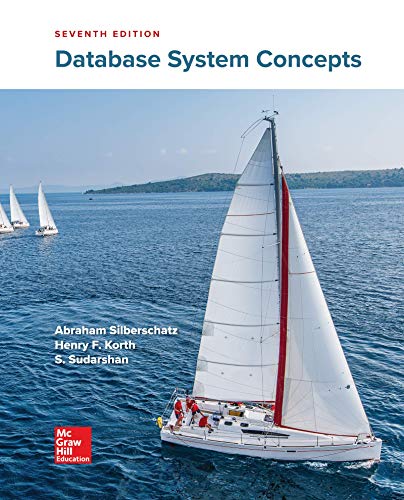
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
I need help with this Java Problem as described in the image below:
import java.util.Scanner;
public class LabProgram {
public static int fibonacci(int n) {
/* Type your code here. */
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int startNum;
startNum = scnr.nextInt();
System.out.println("fibonacci(" + startNum + ") is " + fibonacci(startNum));
}
}
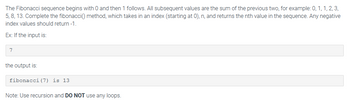
Transcribed Image Text:The Fibonacci sequence begins with 0 and then 1 follows. All subsequent values are the sum of the previous two, for example: 0, 1, 1, 2, 3,
5, 8, 13. Complete the fibonacci() method, which takes in an index (starting at 0), n, and returns the nth value in the sequence. Any negative
index values should return -1.
Ex: If the input is:
7
the output is:
fibonacci (7) is 13
Note: Use recursion and DO NOT use any loops.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- I'm getting this progm to run but vis producing no out put. import java.util.Scanner;import java.lang.Math; public class CarValue { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Car myCar = new Car(); int userYear = scnr.nextInt(); int userPrice = scnr.nextInt(); int userCurrentYear = scnr.nextInt(); myCar.setModelYear(userYear); myCar.setPurchasePrice(userPrice); myCar.calcCurrentValue(userCurrentYear); myCar.printInfo(); }} the calculations are performed in this prigram. private int currentValue; public void setModelYear(int userYear){ modelYear = userYear; } public int getModelYear() { return modelYear; } // Define setPurchasePrice() method public double setPurchasePrice(int userPrice){ return purchasePrice; } // Define getPurchasePrice() method public double getPurchasePrice(int userPrice){ return purchasePrice; }…arrow_forwardI have my code here and I am getting errors (I have also attached my code): " import java.util.Compiler; public class Test Score { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Ënter the first exam Score: "); Double Score1 = scan.nextDouble(); System.out.println("Enter the second exam Score: "); Double Score2 = scan.nextDouble(); System.out.println("Enter the third exam Score: "); Double Score3 = scan.nextDouble(); System.out.println("Enter the fourth exam Score: "); Double Score4 = scan.nextDouble(); System.out.println("Enter the fifth exam Score: "); Double Score5 = scan.nextDouble(); Double total = Score1 + Score2 + Score3 + Score4 + Score5 Double average = total/5; if (average >= 90) { System.out.printf("your average was a: %f and recieved an A in this Class", average ); } else if (average >= 80) {…arrow_forwardHow do I remove the space at the end of the result? Code: import java.util.Scanner; public class FinalExamAnswers{ public static void main(String [] args) { manipulateString(); //calls function } //your code here public static void manipulateString() { Scanner sc=new Scanner(System.in); //create Scanner instance System.out.println("Enter a sentence"); String sentence=sc.nextLine(); //input a sentence String[] words=sentence.split(" "); //split the sentence at space and store it in array for(int i=0;i<words.length;i++) //i from 0 to last index { if(i%2==0) //if even index words[i]=words[i].toUpperCase(); //converted to upper case else //if odd index words[i]=words[i].toLowerCase(); //converted to lower case } for(int i=words.length-1;i>=0;i--) //i from last index to 0 {…arrow_forward
- JAVA CODE: check outputarrow_forwardThe language is Java. The code provided is what I have however it is not outputting the correct number, only 0.arrow_forwardI need help with fixing this java program as described in the image below: import java.util.Scanner; public class LabProgram { /* TODO: Write recursive drawTriangle() method here. */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int baseLength; baseLength = scnr.nextInt(); drawTriangle(baseLength); } }arrow_forward
- Create the UML Diagram for this java code import java.util.Scanner; interface Positive{ void Number();} class Square implements Positive{ public void Number() { Scanner in=new Scanner(System.in); System.out.println("Enter a number: "); int a = in.nextInt(); if(a>0) { System.out.println("Positive number"); } else { System.out.println("Negative number"); } System.out.println("\nThe square of " + a +" is " + a*a); System.out.println("\nThe cubic of "+ a + " is "+ a*a*a); }} class Sum implements Positive{ public void Number() { Scanner in = new Scanner(System.in); System.out.println("\nEnter the value for a: "); int a = in.nextInt(); System.out.println("Enter the value for b" ); int b= in.nextInt(); System.out.printf("The Difference of two numbers: %d\n", a-b); System.out.printf("The…arrow_forwardPlease help and thank you in advance. The language is Java. I have to write a program that takes and validates a date but it won't output the full date. Here is my current code: import java.util.Scanner; public class P1 { publicstaticvoidmain(String[]args) { // project 1 // date input // date output // Create a Scanner object to read from the keyboard. Scannerkeyboard=newScanner(System.in); // Identifier declarations charmonth; intday; intyear; booleanisLeapyear; StringmonthName; intmaxDay; // Asks user to input date System.out.println("Please enter month: "); month=(char)keyboard.nextInt(); System.out.println("Please enter day: "); day=keyboard.nextInt(); System.out.println("Please enter year: "); year=keyboard.nextInt(); // Formating months switch(month){ case1: monthName="January"; break; case2: monthName="February"; break; case3: monthName="March"; break; case4: monthName="April"; break; case5: monthName="May"; break; case6: monthName="June"; break; case7:…arrow_forwardimport java.util.Scanner; public class LabProgram { public static Roster getInput(){ /* Reads course title, creates a roster object with the input title. Note that */ /* the course title might have spaces as in "COP 3804" (i.e. use nextLine) */ /* reads input student information one by one, creates a student object */ /* with each input student and adds the student object to the roster object */ /* the input is formatted as in the sample input and is terminated with a "q" */ /* returns the created roster */ /* Type your code here */ } public static void main(String[] args) { Roster course = getInput(); course.display(); course.dislayScores(); }} public class Student { String id; int score; public Student(String id, int score) { /* Student Employee */ /* Type your code here */ } public String getID() { /* returns student's id */…arrow_forward
- I need help with a java code problem that needs this output shown in image below: import java.util.Scanner;import java.util.NoSuchElementException; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int val1 = 0, val2 = 0, val3 = 0; // Initialize the variables int max; int inputCounter = 0; // Counter to keep track of the number of inputs read try { String inputLine = scnr.nextLine(); String[] parts = inputLine.split(" "); if (parts.length > 0 && !parts[0].isEmpty()) { val1 = Integer.parseInt(parts[0]); inputCounter++; } if (parts.length > 1 && !parts[1].isEmpty()) { val2 = Integer.parseInt(parts[1]); inputCounter++; } if (parts.length > 2 && !parts[2].isEmpty()) { val3 =…arrow_forwardThis is my program. I need help with the math. import java.util.*;import javax.swing.JOptionPane;public class Craps { public static void main(String[] args) { int Bet, Die; int WinC = 0, LossC = 0, GameC = 0, GameN = 1; double startingBal = 0, curBal = 0; double balD, balDe, balDec, balI, balIn, balInc; char Answer = '\0'; Scanner crapsGame = new Scanner(System.in); System.out.println("Welcome to the Craps Game"); curBal = StartingBal(startingBal); System.out.println("Your starting balance is: $" + curBal); do { System.out.println("Please input your bet for the game>> "); Bet = crapsGame.nextInt(); System.out.println("Game #" + GameN + " Starting with bet of: $" + Bet ); if (Bet < 1 || Bet > curBal) { System.out.println("Invalid bet – Your balance is only: $" + curBal); while (Bet < 1 || Bet > curBal)…arrow_forwardGiven string inputStr on one line and integers idx1 and idx2 on a second line, output "Match found" if the character at index idx1 of inputStr is equal to the character at index idx2. Otherwise, output "Match not found". End with a newline. Ex: If the input is: eerie 4 1 then the output is: Match found Note: Assume the length of string inputStr is greater than or equal to both idx1 and idx2.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
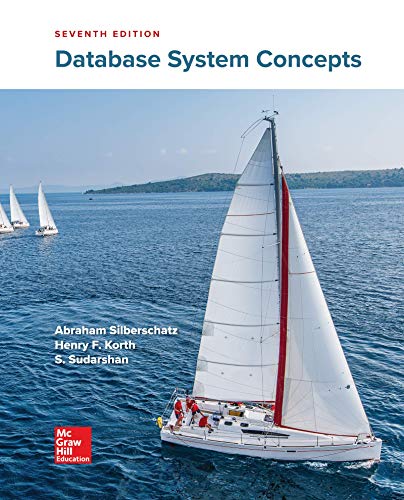
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
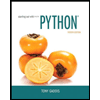
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
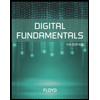
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
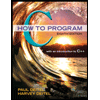
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
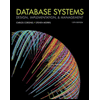
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
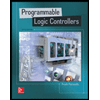
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education