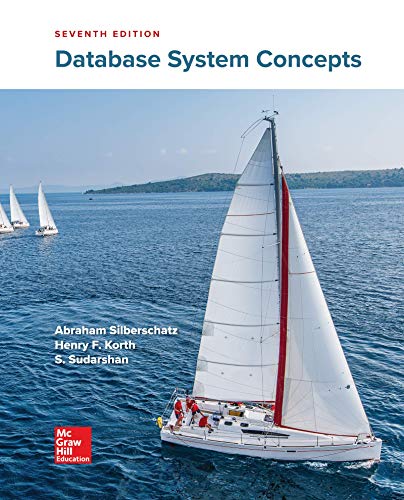
I need help with a Java question so that it can output them described in the image below:
import java.util.Scanner;
public class LabProgram {
public static void sortArray(int[] myArr, int arrSize) {
for (int i = 0; i < arrSize - 1; i++) {
for (int j = 0; j < arrSize - i - 1; j++) {
if (myArr[j] < myArr[j + 1]) {
// Swap elements if they are in the wrong order
int temp = myArr[j];
myArr[j] = myArr[j + 1];
myArr[j + 1] = temp;
}
}
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Read the number of elements in the array
int arrSize = scanner.nextInt();
int[] myArr = new int[arrSize];
// Read and populate the array with elements
for (int i = 0; i < arrSize; i++) {
myArr[i] = scanner.nextInt();
}
// Call the sorting method
sortArray(myArr, arrSize);
// Output the sorted array
for (int i = 0; i < arrSize; i++) {
System.out.print(myArr[i]);
if (i < arrSize - 1) {
System.out.print(",");
}
}
System.out.println();
}
}
![### Test Case Analysis: Sorting Function
This page provides an analysis of test cases for a sorting function, focusing on discrepancies between the actual output and expected results.
#### Test Case 1
- **Input**: `[5, 10, 4, 39, 12, 2]`
- **Your output**: `[39, 12, 10, 4, 2]`
- **Expected output**: `[39, 12, 10, 4, 2, ]`
**Issue**: The output is missing a trailing comma.
---
#### Test Case 2
- **Input**: `[3, 99, 3, 27]`
- **Your output**: `[99, 27, 3]`
- **Expected output**: `[99, 27, 3, ]`
**Issue**: The output is missing a trailing comma.
---
#### Test Case 3
- **Input**: `[1, 25]`
- **Your output**: `[25]`
- **Expected output**: `[25, ]`
**Issue**: The output is missing a trailing comma.
---
### Unit Test Analysis
- **Function call**: `sortArray([61, 92, 0, 22])`
- **Test feedback**:
- `sortArray([61, 92, 0, 22]) correctly returned [92, 61, 22, 0, ]`
The function executed successfully for this input, producing the correct result with the trailing comma included.
---
### Conclusion
The discrepancies highlighted indicate a consistent issue with missing trailing commas in the function's output. Adjustments are necessary to ensure that the output format matches the expected results consistently across tests.](https://content.bartleby.com/qna-images/question/4e135d5c-f867-4afd-9f0d-b505f4f19664/2379047b-6adc-4ac5-8904-cf2ab0555fa2/6c132r9_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Java - How to make this a horizontal statement with a newline. Whenever I use System.out.println, it makes the output vertical instead of horizontal. What am I doing wrong? Program below. import java.util.Scanner; public class LabProgram { public static void main(String[] args) { int i; String InputString; Scanner sc = new Scanner(System.in); InputString = sc.nextLine(); char[] strArray = new char[InputString.length()]; for(i = 0; i < InputString.length(); i++){ strArray[i] = InputString.charAt(i); } for(i = 0 ; i< InputString.length() ; i++) if(strArray[i] >= 'a' && strArray[i] <= 'z' || strArray[i] >= 'A' && strArray[i] <= 'Z') System.out.print(strArray[i]); (the problem line) }}arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardWrite in Java What is this program's exact outputarrow_forward
- I need help with this Java Problem as described in the image below: import java.util.Scanner; public class LabProgram { public static int fibonacci(int n) { /* Type your code here. */ } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int startNum; startNum = scnr.nextInt(); System.out.println("fibonacci(" + startNum + ") is " + fibonacci(startNum)); }}arrow_forwardModify this code to make a moving animated house. import java.awt.Color;import java.awt.Font;import java.awt.Graphics;import java.awt.Graphics2D; /**** @author**/public class MyHouse { public static void main(String[] args) { DrawingPanel panel = new DrawingPanel(750, 500); panel.setBackground (new Color(65,105,225)); Graphics g = panel.getGraphics(); background(g); house (g); houseRoof (g); lawnRoof (g); windows (g); windowsframes (g); chimney(g); } /** * * @param g */ static public void background(Graphics g) { g.setColor (new Color (225,225,225));//clouds g.fillOval (14,37,170,55); g.fillOval (21,21,160,50); g.fillOval (351,51,170,55); g.fillOval (356,36,160,50); } static public void house (Graphics g){g.setColor (new Color(139,69,19)); //houseg.fillRect (100,250,400,200);g.fillRect (499,320,200,130);g.setColor(new Color(190,190,190)); //chimney and doorsg.fillRect (160,150,60,90);g.fillRect…arrow_forwardI need help with this Java problem to output as it's explained in this image below: import java.util.Scanner;import java.util.ArrayList;import java.util.Collections;public class LabProgram { static public int recursions = 0; static public int comparisons = 0; private static ArrayList<Integer> readNums(Scanner scnr) { int size = scnr.nextInt(); ArrayList<Integer> nums = new ArrayList<Integer>(); for (int i = 0; i < size; ++i) { nums.add(scnr.nextInt()); } return nums; } static public int binarySearch(int target, ArrayList<Integer> integers, int lower, int upper) { recursions+=1; int index = (lower+upper)/2; comparisons+=1; if(target == integers.get(index)){ return index; } if(lower == upper){ comparisons+=1; if(target == integers.get(lower)){ return lower; } else{…arrow_forward
- I need help with this Java problem to output as it's explained in this image below import java.util.Scanner; public class LabProgram { public static int fibonacci(int n) { if (n <= 1) { return n; } else { return fibonacci(n - 1) + fibonacci(n - 2); } } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int startNum; startNum = scnr.nextInt(); System.out.println("fibonacci(" + startNum + ") is " + fibonacci(startNum)); }}arrow_forwardI need help with my java compiler program by Generating intermediate code from the AST, such as three-address code or bytecode import java.util.*; public class SimpleCalculator { private final String input;private int position;private boolean hasDivByZero = false;private Map<String, String> symbolTable; public SimpleCalculator(String input) { this.input = input; this.position = 0; this.symbolTable = new HashMap<>();} public static void main(String[] args) { SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)"); int result = calculator.parseExpression(); if (calculator.hasDivByZero) { System.out.println("Error: division by zero"); } else { System.out.println("Result: " + result); }} public int parseExpression() { int value = parseTerm(); while (true) { if (consume('+')) { value += parseTerm(); } else if (consume('-')) { value -= parseTerm(); } else {…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
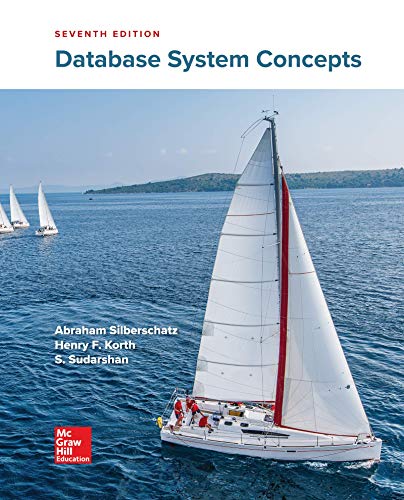
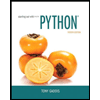
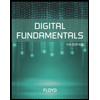
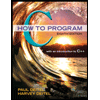
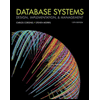
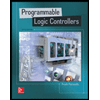