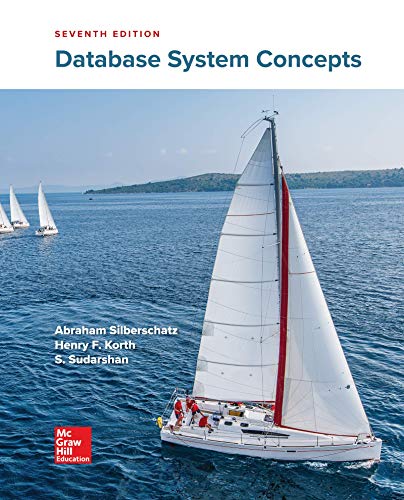
Concept explainers
I need help with this Java problem to output as it's explained in this image below:
import java.util.Scanner;
import java.util.ArrayList;
import java.util.Collections;
public class LabProgram {
static public int recursions = 0;
static public int comparisons = 0;
private static ArrayList<Integer> readNums(Scanner scnr) {
int size = scnr.nextInt();
ArrayList<Integer> nums = new ArrayList<Integer>();
for (int i = 0; i < size; ++i) {
nums.add(scnr.nextInt());
}
return nums;
}
static public int binarySearch(int target, ArrayList<Integer> integers, int lower, int upper) {
recursions+=1;
int index = (lower+upper)/2;
comparisons+=1;
if(target == integers.get(index)){
return index;
}
if(lower == upper){
comparisons+=1;
if(target == integers.get(lower)){
return lower;
}
else{
return -1;
}
}
else{
comparisons+=1;
if(integers.get(index) > target){
return binarySearch(target,integers,lower,index-1);
}
else{
return binarySearch(target,integers,index + 1,upper);
}
}
}
public static void main(String [] args) {
Scanner scnr = new Scanner(System.in);
ArrayList<Integer> integers = readNums(scnr);
int target = scnr.nextInt();
int index = binarySearch(target, integers, 0, integers.size()- 1);
System.out.printf("index: %d, recursions: %d, comparisons: %d", index, recursions, comparisons);
System.out.println();
}
}
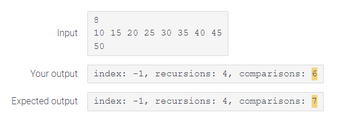

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Please use the template provided below and make sure the output matches exactly. import java.util.Scanner;import java.util.ArrayList; public class PhotoLineups { // TODO: Write method to create and output all permutations of the list of names. public static void printAllPermutations(ArrayList<String> permList, ArrayList<String> nameList) { } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); ArrayList<String> nameList = new ArrayList<String>(); ArrayList<String> permList = new ArrayList<String>(); String name; // TODO: Read in a list of names; stop when -1 is read. Then call recursive method. }}arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardWrite in Java What is this program's exact outputarrow_forward
- need help finishing/fixing this java code so it prints out all the movie names that start with the first 2 characters the user inputs the code is as follows: Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String…arrow_forwardI need help with this Java Problem as described in the image below: import java.util.Scanner; public class LabProgram { public static int fibonacci(int n) { /* Type your code here. */ } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int startNum; startNum = scnr.nextInt(); System.out.println("fibonacci(" + startNum + ") is " + fibonacci(startNum)); }}arrow_forwardModify this code to make a moving animated house. import java.awt.Color;import java.awt.Font;import java.awt.Graphics;import java.awt.Graphics2D; /**** @author**/public class MyHouse { public static void main(String[] args) { DrawingPanel panel = new DrawingPanel(750, 500); panel.setBackground (new Color(65,105,225)); Graphics g = panel.getGraphics(); background(g); house (g); houseRoof (g); lawnRoof (g); windows (g); windowsframes (g); chimney(g); } /** * * @param g */ static public void background(Graphics g) { g.setColor (new Color (225,225,225));//clouds g.fillOval (14,37,170,55); g.fillOval (21,21,160,50); g.fillOval (351,51,170,55); g.fillOval (356,36,160,50); } static public void house (Graphics g){g.setColor (new Color(139,69,19)); //houseg.fillRect (100,250,400,200);g.fillRect (499,320,200,130);g.setColor(new Color(190,190,190)); //chimney and doorsg.fillRect (160,150,60,90);g.fillRect…arrow_forward
- import java.util.ArrayList;import java.util.Scanner;public class CIS231A4JLeh {public static void main(String[] args) {Scanner scnr = new Scanner(System.in);ArrayList<Integer> integers = new ArrayList<>();String input = scnr.nextLine();while (input.isEmpty()) {String[] parts = input.split("\\s+");for (String part : parts) {try {integers.add(Integer.parseInt(part));} catch (NumberFormatException ignored) {}}input = scnr.nextLine();}System.out.println("Jakob");System.out.println("Number of integers input: " + integers.size());System.out.println("All values input, in ascending order:");printIntegers(integers);System.out.println("Lowest value input: " + integers.get(0));System.out.println("Highest value input: " + integers.get(integers.size() - 1));System.out.printf("Average of all values input: %.2f%n", calculateAverage(integers));System.out.println("Mode of the data set and its frequency: " + calculateMode(integers));}private static void printIntegers(ArrayList<Integer>…arrow_forwardI need help with my java compiler program by Generating intermediate code from the AST, such as three-address code or bytecode import java.util.*; public class SimpleCalculator { private final String input;private int position;private boolean hasDivByZero = false;private Map<String, String> symbolTable; public SimpleCalculator(String input) { this.input = input; this.position = 0; this.symbolTable = new HashMap<>();} public static void main(String[] args) { SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)"); int result = calculator.parseExpression(); if (calculator.hasDivByZero) { System.out.println("Error: division by zero"); } else { System.out.println("Result: " + result); }} public int parseExpression() { int value = parseTerm(); while (true) { if (consume('+')) { value += parseTerm(); } else if (consume('-')) { value -= parseTerm(); } else {…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
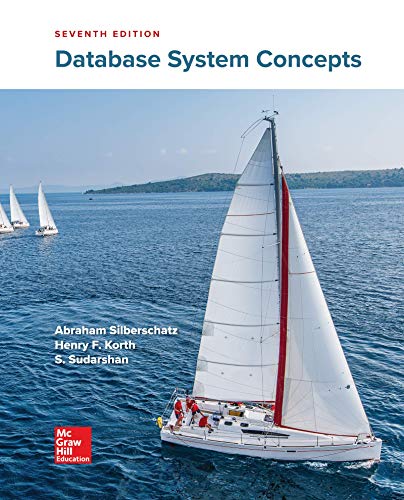
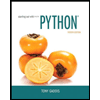
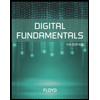
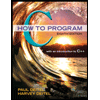
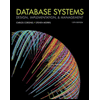
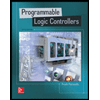